Need to implement user color selection for your Android app? This library is a great choice. Without a long introduction, let's get started.As always, first weβll add a library to the application (file build.gradle (module.app)): Weimplementation 'com.jaredrummler:colorpicker:1.1.0'
figured it out. Now we proceed directly to the implementation of the choice of color.Create the markup:<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/activityMain"
tools:context=".MainActivity">
<Button
android:id="@+id/firstButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Color picker β1"
android:layout_margin="5dp"
android:onClick="onClickButton"
/>
<Button
android:id="@+id/secondButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Color picker β2"
android:layout_below="@id/firstButton"
android:layout_margin="5dp"
android:onClick="onClickButton"
/>
<TextView
android:id="@+id/firstText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="First text"
android:textSize="40sp"
android:textColor="@android:color/black"
/>
<TextView
android:id="@+id/secondText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Second text"
android:textSize="40sp"
android:layout_below="@+id/firstText"
android:layout_centerInParent="true"
android:textColor="@android:color/black"
/>
</RelativeLayout>
We have 2 buttons, by clicking on which a dialog box for choosing a color will open. When we selected a color, it will change in our two TextViews.Add our fields to MainActivity: Button firstButton,secondButton;
TextView firstText,secondText;
private static final int firstId = 1,secondId = 2;
... and initialize them in onCreate ():
firstButton = findViewById(R.id.firstButton);
secondButton = findViewById(R.id.secondButton);
firstText = findViewById(R.id.firstText);
secondText = findViewById(R.id.secondText);
IMPORTANT : It is also necessary that MainActivity implement the methods of the ColorPickerDialogListener interface:
Now we will create a method for creating a dialog box and the onClick method specified in the XML markup:private void createColorPickerDialog(int id) {
ColorPickerDialog.newBuilder()
.setColor(Color.RED)
.setDialogType(ColorPickerDialog.TYPE_PRESETS)
.setAllowCustom(true)
.setAllowPresets(true)
.setColorShape(ColorShape.SQUARE)
.setDialogId(id)
.show(this);
}
public void onClickButton(View view) {
switch (view.getId()) {
case R.id.firstButton:
createColorPickerDialog(firstId);
break;
case R.id.secondButton:
createColorPickerDialog(secondId);
break;
}
}
all attributes of the ColorPickerDialog classYou must also implement the methods of the ColorPickerDialogListener interface:@Override
public void onColorSelected(int dialogId, int color) {
switch (dialogId) {
case firstId:
firstText.setTextColor(color);
break;
case secondId:
secondText.setTextColor(color);
break;
}
}
@Override
public void onDialogDismissed(int dialogId) {
Toast.makeText(this, "Dialog dismissed", Toast.LENGTH_SHORT).show();
}
Launch and ... done!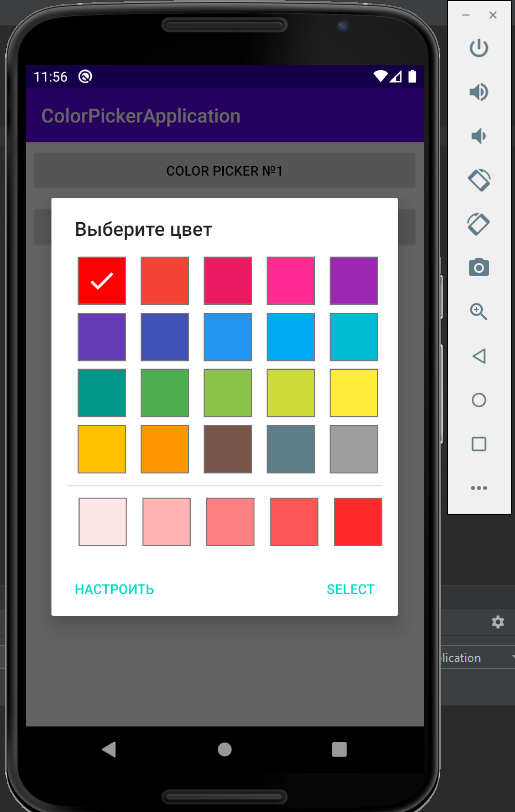
However, this is not all the possibilities of the ColorPicker library. She also adds preference for PreferenceScreen. This allows you to implement the color selection in the settings. Let's see how it works.1) Create a new SettingsActivity:
2) Open the root_preferences.xml file and change it as follows:<PreferenceScreen xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android">
<PreferenceCategory app:title=" ">
<com.jaredrummler.android.colorpicker.ColorPreferenceCompat
android:key="color_picker"
app:title=" "
/>
</PreferenceCategory>
</PreferenceScreen>
As you can see, we created a Preference of type ColorPreferenceCompat3) Create a button in activity_main.xml to go to our SettingsActivty:<Button
android:id="@+id/settingsButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_margin="5dp"
android:onClick="openSettingsActivity"
/>
4) Create an openSettingsActivity method in MainActivity and specify it in the βonClickβ field of this button:public void openSettingsActivity(View view) {
startActivity(new Intent(this,SettingsActivity.class));
}
In the same MainActivity, create a method that changes its background depending on the color selected in the settings and call this filter in onCreate:private void setBackgroundColorFromSettingsActivity() {
SharedPreferences sp = PreferenceManager.getDefaultSharedPreferences(this);
//SharedPreferences
// - :
//https://developer.android.com/reference/android/content/SharedPreferences?hl=ru
int color = sp.getInt("color_picker",Color.GREEN);
RelativeLayout layout = findViewById(R.id.activityMain);
layout.setBackgroundColor(color);
}
Redefine the onResume method (more details here ):@Override
protected void onResume() {
setBackgroundColorFromSettingsActivity();
super.onResume();
}
5) Run the application and see what happened:
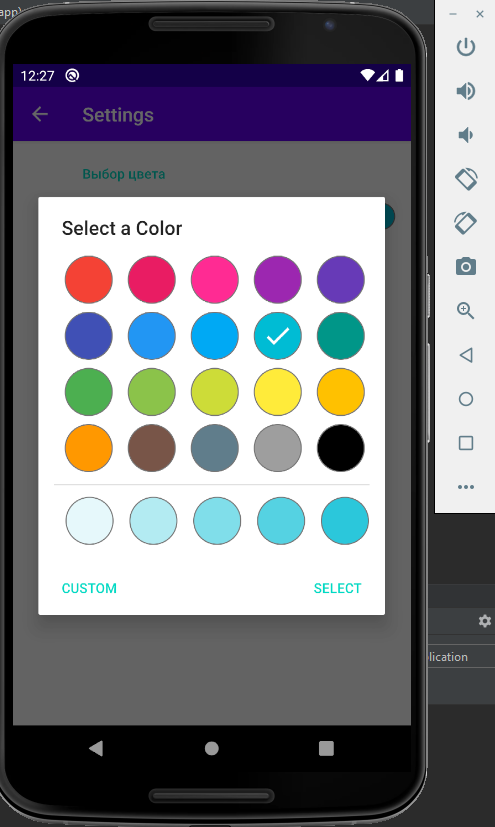
As you can see, everything works correctly.PS: Useful links:PSS: GitHub Application Code