Introduction
Today I will talk about how to recognize the contour of the desired color using python / opencv, such a task is often found in robotics, and all kinds of automation.Using the proposed solution, you can, for example, distinguish the contour of a line beyond which a robot should not travel, or an object for a copter. Such a task may arise if you need to, for example, see that an orange is put in the basket .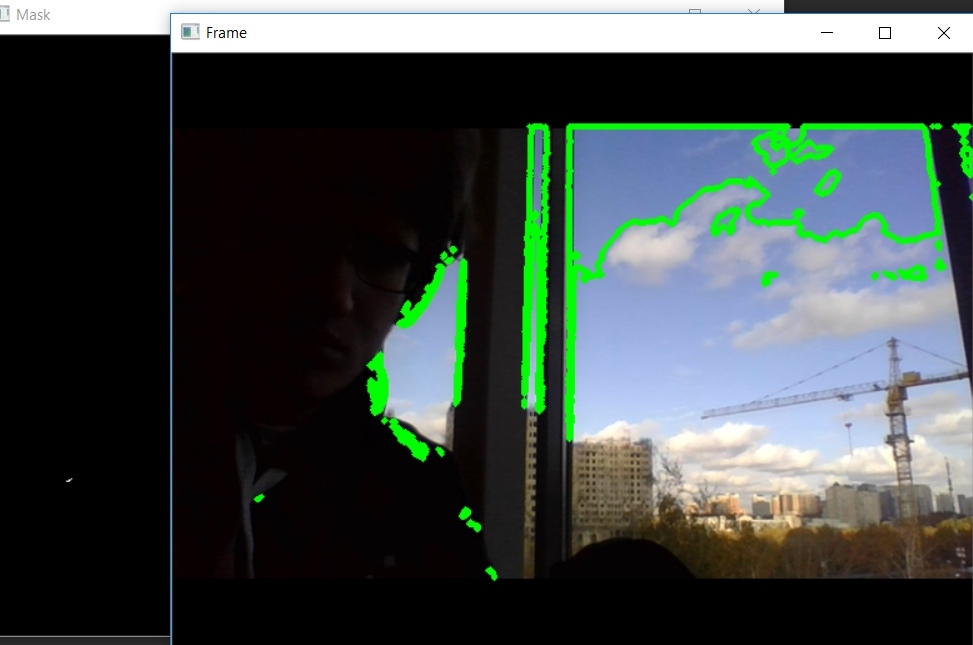
What to learn
Initially, I assume that a person has a python ide (practically any will do) and basic knowledge of python.Install the OpenCV library using the following command:pip install opencv-python
And numpy:pip install numpy
The code
Add the necessary libraries:import cv2
import numpy as np
Create a cup object where the video will go. 0 - default camera, 1 - custom.cap = cv2.VideoCapture(0)
_, frame = cap.read()
reads a frame. instead of _ there may be a rate - fps cameras will go there, but in this case I do not use it.And now let's process the frame for further convenience. Reduce:
frameresized = cv2.resize(frame, None, fx=2, fy=2, interpolation=cv2.INTER_CUBIC)
hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
hsv = cv2.blur(hsv, (5, 5))
mask = cv2.inRange(hsv, (89, 124, 73), (255, 255, 255))
lower_blue = np.array([38, 86, 0])
Let's take a closer look at the resize function:frame -
fx -
fy - Y
interpolation - INTER_NEAREST -
INTER_LINEAR - ( )
INTER_AREA - . INTER_NEAREST method.
INTER_CUBIC - 4x4
INTER_LANCZOS4 - 8x8
And now let's recognize the contours on the processed frame and sort them: contours, _ = cv2.findContours(mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_NONE)
counturs = sorted(contours, key=cv2.contourArea, reverse=True)
Now let's look at findContours in more detail: cv.CHAIN_APPROX_NONE - ( )
cv.CHAIN_APPROX_SIMPLE - - ( )
cv.RETR_TREE -
cv.RETR_EXTERNAL -
cv.RETR_CCOMP - 2 , 1 ,
cv2.RETR_TREE -
Visualization RETR_CCOMP:
Visualization RETR_TREE:
And now we will draw the contours that we found:or contour in counturs:
cv2.drawContours(frame, counturs[0], -1, (255, 0, 255), 3)
cv2.imshow("Counturs", frame)
key = cv2.waitKey(1)
if key == 27:
break
And at the touch of a button, we stop reading the camera and close all the windows:cap.release()
cv2.destroyAllWindows()
Repository
Thanks for reading the article.I would be grateful if you subscribe to my channel intelegram
my game
Upd - itโs funny that the article got about 8 times less views than expected, but at the same time, the estimated number of โbookmarksโ is only twice as many. That's how we live.