尽管此信息已经过时,但原始材料仍然是FFmpeg主题上各种有用内容的流行灵感来源。但是,仍然没有将原件完全翻译成俄文。我们更正了烦人的遗漏,因为迟到总比没有好。尽管我们尝试了,但是在如此庞大的文本中翻译的困难是不可避免的。报告错误(最好是在私人消息中)-我们在一起将做得更好。目录

EDISON.
, C C++.
! ;-)
UPD:本指南已于2015年2月更新。FFmpeg是用于创建视频应用程序和通用实用程序的出色库。 FFmpeg负责整个视频处理例程,执行所有解码,编码,复用和解复用。这极大地简化了媒体应用程序的创建。一切都非常简单,快捷,用C编写,您可以解码当今几乎所有可用的编解码器,以及编码为其他格式。唯一的不足是该文档几乎丢失了。有一个教程(在原始教程中,这是到一个不存在的网页的链接-注释翻译器),涵盖了FFmpeg的基础知识和doxygen扩展坞的自动生成。仅此而已。因此,我决定独立研究如何使用FFmpeg创建有效的数字视频和音频应用程序,同时记录该过程并以教科书的形式进行介绍。FFmpeg附带有一个FFplay程序。它很简单,用C编写,使用FFmpeg实现了完整的视频播放器。我的第一课是Martin Boehme的原始课程的更新版本(原始语言是指向已经失效的网页的链接-译者注)-我从那里拖了一些片段。在我的系列课程中,我还将展示基于ffplay.c创建有效的视频播放器的过程法布里斯·贝勒(Fabrice Bellard)。每节课将介绍一个新的想法(甚至两个),并说明其实施方式。每章都有一个C清单,您可以编译并自行运行。源文件将显示该程序的工作方式,各个部分的工作方式,并演示本指南未涵盖的次要技术细节。完成后,我们将使用不到1000行代码编写一个可正常工作的视频播放器!创建播放器时,我们将使用SDL输出音频和视频媒体文件。 SDL是一个出色的跨平台多媒体库,可用于MPEG播放程序,仿真器和许多视频游戏。您需要在系统上下载并安装SDL库,才能从本指南中编译程序。本教程适用于具有良好编程经验的人。至少,您需要了解C,并且还需要了解队列,互斥对象等概念。应该对多媒体有所了解;例如,诸如波形之类的东西。但是,不必在这些问题上担任专家,因为在上课过程中将解释许多概念。请随时通过Dranger Doggy Gmail dot Com向我发送错误消息,问题,评论,想法,功能等。
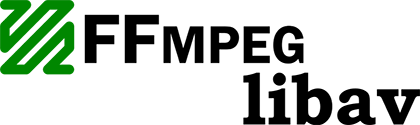
另请参阅
EDISON公司的博客:
FFmpeg libav手册
第1课:创建屏幕截图← ⇑ →
完整清单:tutorial01.c
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <stdio.h>
#if LIBAVCODEC_VERSION_INT < AV_VERSION_INT(55,28,1)
#define av_frame_alloc avcodec_alloc_frame
#define av_frame_free avcodec_free_frame
#endif
void SaveFrame(AVFrame *pFrame, int width, int height, int iFrame) {
FILE *pFile;
char szFilename[32];
int y;
sprintf(szFilename, "frame%d.ppm", iFrame);
pFile=fopen(szFilename, "wb");
if(pFile==NULL)
return;
fprintf(pFile, "P6\n%d %d\n255\n", width, height);
for(y=0; y<height; y++)
fwrite(pFrame->data[0]+y*pFrame->linesize[0], 1, width*3, pFile);
fclose(pFile);
}
int main(int argc, char *argv[]) {
AVFormatContext *pFormatCtx = NULL;
int i, videoStream;
AVCodecContext *pCodecCtxOrig = NULL;
AVCodecContext *pCodecCtx = NULL;
AVCodec *pCodec = NULL;
AVFrame *pFrame = NULL;
AVFrame *pFrameRGB = NULL;
AVPacket packet;
int frameFinished;
int numBytes;
uint8_t *buffer = NULL;
struct SwsContext *sws_ctx = NULL;
if(argc < 2) {
printf("Please provide a movie file\n");
return -1;
}
av_register_all();
if(avformat_open_input(&pFormatCtx, argv[1], NULL, NULL)!=0)
return -1;
if(avformat_find_stream_info(pFormatCtx, NULL)<0)
return -1;
av_dump_format(pFormatCtx, 0, argv[1], 0);
videoStream=-1;
for(i=0; i<pFormatCtx->nb_streams; i++)
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO) {
videoStream=i;
break;
}
if(videoStream==-1)
return -1;
pCodecCtxOrig=pFormatCtx->streams[videoStream]->codec;
pCodec=avcodec_find_decoder(pCodecCtxOrig->codec_id);
if(pCodec==NULL) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if(avcodec_copy_context(pCodecCtx, pCodecCtxOrig) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(avcodec_open2(pCodecCtx, pCodec, NULL)<0)
return -1;
pFrame=av_frame_alloc();
pFrameRGB=av_frame_alloc();
if(pFrameRGB==NULL)
return -1;
numBytes=avpicture_get_size(PIX_FMT_RGB24, pCodecCtx->width,
pCodecCtx->height);
buffer=(uint8_t *)av_malloc(numBytes*sizeof(uint8_t));
avpicture_fill((AVPicture *)pFrameRGB, buffer, PIX_FMT_RGB24,
pCodecCtx->width, pCodecCtx->height);
sws_ctx = sws_getContext(pCodecCtx->width,
pCodecCtx->height,
pCodecCtx->pix_fmt,
pCodecCtx->width,
pCodecCtx->height,
PIX_FMT_RGB24,
SWS_BILINEAR,
NULL,
NULL,
NULL
);
i=0;
while(av_read_frame(pFormatCtx, &packet)>=0) {
if(packet.stream_index==videoStream) {
avcodec_decode_video2(pCodecCtx, pFrame, &frameFinished, &packet);
if(frameFinished) {
sws_scale(sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, pCodecCtx->height,
pFrameRGB->data, pFrameRGB->linesize);
if(++i<=5)
SaveFrame(pFrameRGB, pCodecCtx->width, pCodecCtx->height,
i);
}
}
av_free_packet(&packet);
}
av_free(buffer);
av_frame_free(&pFrameRGB);
av_frame_free(&pFrame);
avcodec_close(pCodecCtx);
avcodec_close(pCodecCtxOrig);
avformat_close_input(&pFormatCtx);
return 0;
}
总览
电影文件具有几个主要组成部分。首先,文件本身称为容器,容器的类型决定了文件中数据的表示方式。容器的示例是AVI和Quicktime。此外,文件中有多个线程。特别地,通常存在音频流和视频流。 (“流”是一个有趣的词,意为“根据时间轴可用的一系列数据项。”)流中的数据项称为帧。每个流由一种或另一种类型的编解码器编码。编解码器确定如何将实际数据发送到 diruyutsya和December已审核-编解码器的名称。DivX和MP3是编解码器的示例。然后从流中读取数据包。数据包是数据片段,可以包含解码为原始帧的数据位,我们最终可以在应用程序中对其进行操作。为了我们的目的,每个数据包都包含完整帧(如果是音频,则包含几个帧)。即使在最基本的级别上,使用视频和音频流也非常简单:10 OPEN video_stream FROM video.avi
20 READ packet FROM video_stream INTO frame
30 IF frame NOT COMPLETE GOTO 20
40 DO SOMETHING WITH frame
50 GOTO 20
使用FFmpeg使用多媒体几乎与该程序一样简单,尽管在某些程序中“ MAKE”步骤可能非常困难。在本教程中,我们将打开文件,计算其中的视频流,然后我们的“ MAKE”将帧写入PPM文件。打开文件
首先,让我们看看打开文件时首先发生的情况。首先使用FFmpeg初始化所需的库:#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <ffmpeg/swscale.h>
...
int main(int argc, charg *argv[]) {
av_register_all();
这会在库中注册所有可用的文件格式和编解码器,因此在打开具有适当格式/编解码器的文件时将自动使用它们。请注意,您只需要调用av_register_all()一次,因此我们在main()中进行此操作。如果愿意,可以仅注册选择性文件格式和编解码器,但通常没有特殊原因。现在打开文件:AVFormatContext *pFormatCtx = NULL;
if(avformat_open_input(&pFormatCtx, argv[1], NULL, 0, NULL)!=0)
return -1;
从第一个参数获取文件名。此函数读取文件头并将文件格式信息存储在我们传递的AVFormatContext结构中。最后三个参数用于指定文件格式,缓冲区大小和格式参数。通过将它们设置为NULL或0,libavformat将自动检测所有内容。该函数仅查看标头,因此现在我们需要检查文件中的流信息:
if(avformat_find_stream_info(pFormatCtx, NULL)<0)
return -1;
该函数将有效数据传递给pFormatCtx- > stream。我们熟悉了方便的调试功能,向我们展示了其中的内容:
av_dump_format(pFormatCtx, 0, argv[1], 0);
现在pFormatCtx- > streams只是一个大小为pFormatCtx- > nb_streams的指针数组。我们将仔细研究直到找到视频流:int i;
AVCodecContext *pCodecCtxOrig = NULL;
AVCodecContext *pCodecCtx = NULL;
videoStream=-1;
for(i=0; i<pFormatCtx->nb_streams; i++)
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO) {
videoStream=i;
break;
}
if(videoStream==-1)
return -1;
pCodecCtx=pFormatCtx->streams[videoStream]->codec;
有关流中编解码器的信息位于称为“ 编解码器上下文 ”的位置。它包含有关流使用的编解码器的所有信息,现在我们有一个指向它的指针。但是我们仍然必须找到真正的编解码器并打开它:AVCodec *pCodec = NULL;
pCodec=avcodec_find_decoder(pCodecCtx->codec_id);
if(pCodec==NULL) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if(avcodec_copy_context(pCodecCtx, pCodecCtxOrig) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(avcodec_open2(pCodecCtx, pCodec)<0)
return -1;
请注意,您不能直接从视频流中使用AVCodecContext!因此,您必须使用vcodec_copy_context()将上下文复制到新位置(当然,在为它分配内存之后)。数据存储
现在我们需要一个存放框架的地方:AVFrame *pFrame = NULL;
pFrame=av_frame_alloc();
由于我们计划输出以24位RGB存储的PPM文件,因此我们需要将帧从其自身的格式转换为RGB。FFmpeg将为我们做到这一点。对于大多数项目(包括此项目),您需要将起始帧转换为特定格式。为转换后的帧选择一个帧:
pFrameRGB=av_frame_alloc();
if(pFrameRGB==NULL)
return -1;
尽管我们选择了框架,但在转换原始数据时仍需要一个地方来容纳原始数据。我们使用avpicture_get_size来获取正确的大小并手动分配必要的空间:uint8_t *buffer = NULL;
int numBytes;
numBytes=avpicture_get_size(PIX_FMT_RGB24, pCodecCtx->width,
pCodecCtx->height);
buffer=(uint8_t *)av_malloc(numBytes*sizeof(uint8_t));
av_malloc是FFmpeg 的C函数malloc的类似物,它是malloc的简单包装,提供内存地址等的对齐方式。顺便说一句,这不能防止内存泄漏,双重释放或malloc发生的其他问题。现在,我们使用avpicture_fill将帧与新分配的缓冲区关联。关于AVPicture:所述AVPicture结构是的一个子集AVFrame结构-的开头AVFrame结构是相同于AVPicture结构。
avpicture_fill((AVPicture *)pFrameRGB, buffer, PIX_FMT_RGB24,
pCodecCtx->width, pCodecCtx->height);
我们已经到了终点线!现在我们准备从流中读取信息!读取数据
现在,要读取整个视频流,我们将读取下一个包,并在帧中对其进行解密,解密完成后,立即转换帧并保存:struct SwsContext *sws_ctx = NULL;
int frameFinished;
AVPacket packet;
sws_ctx = sws_getContext(pCodecCtx->width,
pCodecCtx->height,
pCodecCtx->pix_fmt,
pCodecCtx->width,
pCodecCtx->height,
PIX_FMT_RGB24,
SWS_BILINEAR,
NULL,
NULL,
NULL
);
i=0;
while(av_read_frame(pFormatCtx, &packet)>=0) {
if(packet.stream_index==videoStream) {
avcodec_decode_video2(pCodecCtx, pFrame, &frameFinished, &packet);
if(frameFinished) {
sws_scale(sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, pCodecCtx->height,
pFrameRGB->data, pFrameRGB->linesize);
if(++i<=5)
SaveFrame(pFrameRGB, pCodecCtx->width,
pCodecCtx->height, i);
}
}
av_free_packet(&packet);
}
没什么复杂的:av_read_frame()读取包并将其保存在AVPacket结构中。请注意,我们仅分发包的结构-FFmpeg向我们提供了packet.data指向的内部数据。稍后释放av_free_packet()。avcodec_decode_video()将数据包转换为帧。然而,我们可能没有,我们需要对分组进行解码后的帧的所有信息,因此avcodec_decode_video()组frameFinished当我们有下一帧。最后,我们使用sws_scale()从我们自己的格式进行转换(pCodecCtx- >pix_fmt)在RGB中。请记住,可以将 AVFrame指针强制转换为 AVPicture指针。最后,我们传递有关 SaveFrame函数的框架,高度和宽度的信息。说起包裹。从技术上讲,数据包只能包含一部分帧以及其他数据位。但是,FFmpeg解析器保证我们收到的数据包包含完整帧或什至几个帧。现在剩下要做的就是使用 SaveFrame函数将RGB信息写入PPM文件。尽管我们只是在表面上处理PPM格式;相信我,一切都在这里工作:void SaveFrame(AVFrame *pFrame, int width, int height, int iFrame) {
FILE *pFile;
char szFilename[32];
int y;
sprintf(szFilename, "frame%d.ppm", iFrame);
pFile=fopen(szFilename, "wb");
if(pFile==NULL)
return;
fprintf(pFile, "P6\n%d %d\n255\n", width, height);
for(y=0; y<height; y++)
fwrite(pFrame->data[0]+y*pFrame->linesize[0], 1, width*3, pFile);
fclose(pFile);
}
我们执行标准文件打开等操作,然后记录RGB数据。该文件逐行写入。 PPM文件只是其中RGB信息以长行显示的文件。如果您知道HTML的颜色,则就像标记每个像素从头到尾的颜色一样,例如#ff0000#ff0000 ....,就像红色屏幕一样。 (实际上,它以二进制格式存储,没有分隔符,但我希望您能理解。)标题指示图像的宽度和高度,以及RGB值的最大大小。现在回到我们的main()函数。一旦我们完成了从视频流中读取的内容,我们只需清除所有内容:
av_free(buffer);
av_free(pFrameRGB);
av_free(pFrame);
avcodec_close(pCodecCtx);
avcodec_close(pCodecCtxOrig);
avformat_close_input(&pFormatCtx);
return 0;
如您所见,我们将av_free用于使用avcode_alloc_frame和av_malloc分配的内存。这就是全部代码!现在,如果您使用的是Linux或类似平台,请运行:gcc -o tutorial01 tutorial01.c -lavutil -lavformat -lavcodec -lz -lavutil -lm
如果您使用的是较旧版本的FFmpeg,则可能需要删除-lavutil:gcc -o tutorial01 tutorial01.c -lavformat -lavcodec -lz -lm
大多数图形程序必须打开PPM格式。在使用我们的程序制作了屏幕截图的一些电影文件上进行检查。
第2课:显示屏幕← ⇑ →
完整清单:tutorial02.c
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <SDL.h>
#include <SDL_thread.h>
#ifdef __MINGW32__
#undef main
#endif
#include <stdio.h>
#if LIBAVCODEC_VERSION_INT < AV_VERSION_INT(55,28,1)
#define av_frame_alloc avcodec_alloc_frame
#define av_frame_free avcodec_free_frame
#endif
int main(int argc, char *argv[]) {
AVFormatContext *pFormatCtx = NULL;
int i, videoStream;
AVCodecContext *pCodecCtxOrig = NULL;
AVCodecContext *pCodecCtx = NULL;
AVCodec *pCodec = NULL;
AVFrame *pFrame = NULL;
AVPacket packet;
int frameFinished;
float aspect_ratio;
struct SwsContext *sws_ctx = NULL;
SDL_Overlay *bmp;
SDL_Surface *screen;
SDL_Rect rect;
SDL_Event event;
if(argc < 2) {
fprintf(stderr, "Usage: test <file>\n");
exit(1);
}
av_register_all();
if(SDL_Init(SDL_INIT_VIDEO | SDL_INIT_AUDIO | SDL_INIT_TIMER)) {
fprintf(stderr, "Could not initialize SDL - %s\n", SDL_GetError());
exit(1);
}
if(avformat_open_input(&pFormatCtx, argv[1], NULL, NULL)!=0)
return -1;
if(avformat_find_stream_info(pFormatCtx, NULL)<0)
return -1;
av_dump_format(pFormatCtx, 0, argv[1], 0);
videoStream=-1;
for(i=0; i<pFormatCtx->nb_streams; i++)
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO) {
videoStream=i;
break;
}
if(videoStream==-1)
return -1;
pCodecCtxOrig=pFormatCtx->streams[videoStream]->codec;
pCodec=avcodec_find_decoder(pCodecCtxOrig->codec_id);
if(pCodec==NULL) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if(avcodec_copy_context(pCodecCtx, pCodecCtxOrig) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(avcodec_open2(pCodecCtx, pCodec, NULL)<0)
return -1;
pFrame=av_frame_alloc();
#ifndef __DARWIN__
screen = SDL_SetVideoMode(pCodecCtx->width, pCodecCtx->height, 0, 0);
#else
screen = SDL_SetVideoMode(pCodecCtx->width, pCodecCtx->height, 24, 0);
#endif
if(!screen) {
fprintf(stderr, "SDL: could not set video mode - exiting\n");
exit(1);
}
bmp = SDL_CreateYUVOverlay(pCodecCtx->width,
pCodecCtx->height,
SDL_YV12_OVERLAY,
screen);
sws_ctx = sws_getContext(pCodecCtx->width,
pCodecCtx->height,
pCodecCtx->pix_fmt,
pCodecCtx->width,
pCodecCtx->height,
PIX_FMT_YUV420P,
SWS_BILINEAR,
NULL,
NULL,
NULL
);
i=0;
while(av_read_frame(pFormatCtx, &packet)>=0) {
if(packet.stream_index==videoStream) {
avcodec_decode_video2(pCodecCtx, pFrame, &frameFinished, &packet);
if(frameFinished) {
SDL_LockYUVOverlay(bmp);
AVPicture pict;
pict.data[0] = bmp->pixels[0];
pict.data[1] = bmp->pixels[2];
pict.data[2] = bmp->pixels[1];
pict.linesize[0] = bmp->pitches[0];
pict.linesize[1] = bmp->pitches[2];
pict.linesize[2] = bmp->pitches[1];
sws_scale(sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, pCodecCtx->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(bmp);
rect.x = 0;
rect.y = 0;
rect.w = pCodecCtx->width;
rect.h = pCodecCtx->height;
SDL_DisplayYUVOverlay(bmp, &rect);
}
}
av_free_packet(&packet);
SDL_PollEvent(&event);
switch(event.type) {
case SDL_QUIT:
SDL_Quit();
exit(0);
break;
default:
break;
}
}
av_frame_free(&pFrame);
avcodec_close(pCodecCtx);
avcodec_close(pCodecCtxOrig);
avformat_close_input(&pFormatCtx);
return 0;
}
SDL和视频
为了在屏幕上绘图,我们将使用SDL。 SDL代表简单直接层。它是许多项目中使用的出色的跨平台多媒体库。您可以在官方网站上获得该库,或者下载适用于您的操作系统的开发人员软件包。您将需要库来编译本课程中的代码(顺便说一下,所有其他课程也都适用)。SDL有许多在屏幕上绘制的方法。显示电影的一种方法是所谓的YUV覆盖。正式上,甚至不是YUV,而是YCbCr。顺便说一句,当“ YCbCr”被称为“ YUV”时,某些人会非常燃烧。一般来说,YUV是模拟格式,而YCbCr是数字格式。 FFmpeg和SDL在其代码和宏中将YCbCr指定为YUV,但这是。YUV是一种存储原始图像数据(例如RGB)的方法。大致来说,Y是亮度的组成部分,而U和V是颜色的组成部分。 (这比RGB更复杂,因为部分颜色信息被丢弃,并且每2个Y值只能有1个U和V值。)YUV叠加SDL中的接受原始YUV数据集并显示它。它接受4种不同的YUV格式,但是YV12是其中最快的一种。除了交换U和V的数组之外,还有另一种YUV格式称为YUV420P,它与YV12匹配。 420表示以4:2:0的比率对其进行采样,也就是说,每4次亮度测量都进行1次颜色测量,因此颜色信息按四分之一分布。这是节省带宽的好方法,因为人眼仍然没有注意到这些变化。在名称拉丁字母“P”表示该格式是“平面”,它简单地意味着该组件是ÿ,U和V在单独的数组中。FFmpeg可以将图像转换为YUV420P,这非常有帮助,因为许多视频流已经以这种格式存储或易于转换为它。因此,我们当前的计划是替换上一课中的SaveFrame()函数,并显示我们的框架。但是首先,您需要熟悉SDL库的基本功能。首先,连接库并初始化SDL:#include <SDL.h>
#include <SDL_thread.h>
if(SDL_Init(SDL_INIT_VIDEO | SDL_INIT_AUDIO | SDL_INIT_TIMER)) {
fprintf(stderr, "Could not initialize SDL - %s\n", SDL_GetError());
exit(1);
}
SDL_Init()本质上告诉库我们将使用哪些函数。SDL_GetError(),当然,这是我们方便的调试功能。显示制作
现在我们需要在屏幕上放置一个位置来排列元素。使用SDL显示图像的主要区域称为表面:SDL_Surface *screen;
screen = SDL_SetVideoMode(pCodecCtx->width, pCodecCtx->height, 0, 0);
if(!screen) {
fprintf(stderr, "SDL: could not set video mode - exiting\n");
exit(1);
}
因此,我们设置了具有给定宽度和高度的屏幕。下一个选项是屏幕的位深度-0-这是一个特殊值,表示“与当前显示相同”。现在,我们在此屏幕上创建一个YUV叠加层,以便我们可以向其输出视频,并配置SWSContext将图像数据转换为YUV420:SDL_Overlay *bmp = NULL;
struct SWSContext *sws_ctx = NULL;
bmp = SDL_CreateYUVOverlay(pCodecCtx->width, pCodecCtx->height,
SDL_YV12_OVERLAY, screen);
sws_ctx = sws_getContext(pCodecCtx->width,
pCodecCtx->height,
pCodecCtx->pix_fmt,
pCodecCtx->width,
pCodecCtx->height,
PIX_FMT_YUV420P,
SWS_BILINEAR,
NULL,
NULL,
NULL
);
如前所述,我们使用YV12来显示图像并从FFmpeg 获取YUV420数据。图像显示
好吧,这很容易!现在我们只需要显示图像。让我们一直走到完成拍摄的地方。我们可以摆脱RGB帧的所有内容,并用显示代码替换SaveFrame()。为了显示图像,我们将创建一个AVPicture结构,并为YUV叠加层设置其数据指针和行大小: if(frameFinished) {
SDL_LockYUVOverlay(bmp);
AVPicture pict;
pict.data[0] = bmp->pixels[0];
pict.data[1] = bmp->pixels[2];
pict.data[2] = bmp->pixels[1];
pict.linesize[0] = bmp->pitches[0];
pict.linesize[1] = bmp->pitches[2];
pict.linesize[2] = bmp->pitches[1];
sws_scale(sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, pCodecCtx->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(bmp);
首先,我们计划覆盖该覆盖层,因为我们计划对其进行写入。这是一个好习惯,这样以后就没有问题了。如上所示,AVPicture结构具有一个数据指针,该数据指针是4个指针的数组。由于在这里我们要处理YUV420P,所以我们只有3个通道,因此只有3个数据集。其他格式可能具有用于alpha通道或其他内容的第四个指针。行大小就是它的样子。YUV叠加层中的类似结构是像素和高度的变量。 (间距,间距-如果用SDL表示以表示给定数据字符串的宽度。)因此,我们在叠加层上表示了三个pict.data数组,因此当我们写入时pict,实际上是我们在叠加层中进行记录,当然,叠加层已经为它专门分配了必要的空间。以同样的方式,我们直接从叠加层中获得线径信息。我们将转换格式更改为PIX_FMT_YUV420P并像以前一样使用sws_scale。图像绘图
但是我们仍然需要指定SDL,以便它真正显示我们提供给它的数据。我们还向该函数传递了一个矩形,该矩形指示影片应该放哪里,应缩放影片的宽度和高度。因此,SDL可为我们扩展,这可以帮助您的GPU更快地扩展:SDL_Rect rect;
if(frameFinished) {
sws_scale(sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, pCodecCtx->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(bmp);
rect.x = 0;
rect.y = 0;
rect.w = pCodecCtx->width;
rect.h = pCodecCtx->height;
SDL_DisplayYUVOverlay(bmp, &rect);
}
现在,我们的视频已显示!让我们展示SDL的另一个功能:事件系统。 SDL的配置方式使得当您在SDL应用程序中输入或移动鼠标或向其发送信号时,会生成一个事件。然后,如果程序打算处理用户输入,则检查这些事件。您的程序还可以创建事件以将SDL事件发送到系统。这对于使用SDL进行多线程编程特别有用,我们将在第4课中看到这一点。在我们的程序中,我们将在处理程序包后立即检查事件。目前,我们将处理SDL_QUIT事件,以便我们可以退出:SDL_Event event;
av_free_packet(&packet);
SDL_PollEvent(&event);
switch(event.type) {
case SDL_QUIT:
SDL_Quit();
exit(0);
break;
default:
break;
}
这样我们就活着!我们摆脱了所有旧的垃圾,并准备好进行编译。如果您使用Linux或类似Linux的软件,则使用SDL库进行编译的最佳方法是:gcc -o tutorial02 tutorial02.c -lavformat -lavcodec -lswscale -lz -lm \
`sdl-config --cflags --libs`
sdl-config仅显示gcc的必要标志,以正确启用SDL库。您可能需要做其他事情才能在您的系统上进行编译。请为任何消防员检查系统的SDL文档。编译后,继续运行。运行此程序会怎样?该视频似乎快要疯了!实际上,我们只需显示所有视频帧,就可以从电影文件中提取它们一样快。我们现在没有代码来确定何时需要显示视频。最后(第5课),我们将开始同步视频。但是此刻,我们缺少了同样重要的东西:声音!
第3课:播放声音← ⇑ →
完整清单:tutorial03.c
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <SDL.h>
#include <SDL_thread.h>
#ifdef __MINGW32__
#undef main
#endif
#include <stdio.h>
#include <assert.h>
#if LIBAVCODEC_VERSION_INT < AV_VERSION_INT(55,28,1)
#define av_frame_alloc avcodec_alloc_frame
#define av_frame_free avcodec_free_frame
#endif
#define SDL_AUDIO_BUFFER_SIZE 1024
#define MAX_AUDIO_FRAME_SIZE 192000
typedef struct PacketQueue {
AVPacketList *first_pkt, *last_pkt;
int nb_packets;
int size;
SDL_mutex *mutex;
SDL_cond *cond;
} PacketQueue;
PacketQueue audioq;
int quit = 0;
void packet_queue_init(PacketQueue *q) {
memset(q, 0, sizeof(PacketQueue));
q->mutex = SDL_CreateMutex();
q->cond = SDL_CreateCond();
}
int packet_queue_put(PacketQueue *q, AVPacket *pkt) {
AVPacketList *pkt1;
if(av_dup_packet(pkt) < 0) {
return -1;
}
pkt1 = av_malloc(sizeof(AVPacketList));
if (!pkt1)
return -1;
pkt1->pkt = *pkt;
pkt1->next = NULL;
SDL_LockMutex(q->mutex);
if (!q->last_pkt)
q->first_pkt = pkt1;
else
q->last_pkt->next = pkt1;
q->last_pkt = pkt1;
q->nb_packets++;
q->size += pkt1->pkt.size;
SDL_CondSignal(q->cond);
SDL_UnlockMutex(q->mutex);
return 0;
}
static int packet_queue_get(PacketQueue *q, AVPacket *pkt, int block)
{
AVPacketList *pkt1;
int ret;
SDL_LockMutex(q->mutex);
for(;;) {
if(quit) {
ret = -1;
break;
}
pkt1 = q->first_pkt;
if (pkt1) {
q->first_pkt = pkt1->next;
if (!q->first_pkt)
q->last_pkt = NULL;
q->nb_packets--;
q->size -= pkt1->pkt.size;
*pkt = pkt1->pkt;
av_free(pkt1);
ret = 1;
break;
} else if (!block) {
ret = 0;
break;
} else {
SDL_CondWait(q->cond, q->mutex);
}
}
SDL_UnlockMutex(q->mutex);
return ret;
}
int audio_decode_frame(AVCodecContext *aCodecCtx, uint8_t *audio_buf, int buf_size) {
static AVPacket pkt;
static uint8_t *audio_pkt_data = NULL;
static int audio_pkt_size = 0;
static AVFrame frame;
int len1, data_size = 0;
for(;;) {
while(audio_pkt_size > 0) {
int got_frame = 0;
len1 = avcodec_decode_audio4(aCodecCtx, &frame, &got_frame, &pkt);
if(len1 < 0) {
audio_pkt_size = 0;
break;
}
audio_pkt_data += len1;
audio_pkt_size -= len1;
data_size = 0;
if(got_frame) {
data_size = av_samples_get_buffer_size(NULL,
aCodecCtx->channels,
frame.nb_samples,
aCodecCtx->sample_fmt,
1);
assert(data_size <= buf_size);
memcpy(audio_buf, frame.data[0], data_size);
}
if(data_size <= 0) {
continue;
}
return data_size;
}
if(pkt.data)
av_free_packet(&pkt);
if(quit) {
return -1;
}
if(packet_queue_get(&audioq, &pkt, 1) < 0) {
return -1;
}
audio_pkt_data = pkt.data;
audio_pkt_size = pkt.size;
}
}
void audio_callback(void *userdata, Uint8 *stream, int len) {
AVCodecContext *aCodecCtx = (AVCodecContext *)userdata;
int len1, audio_size;
static uint8_t audio_buf[(MAX_AUDIO_FRAME_SIZE * 3) / 2];
static unsigned int audio_buf_size = 0;
static unsigned int audio_buf_index = 0;
while(len > 0) {
if(audio_buf_index >= audio_buf_size) {
audio_size = audio_decode_frame(aCodecCtx, audio_buf, sizeof(audio_buf));
if(audio_size < 0) {
audio_buf_size = 1024;
memset(audio_buf, 0, audio_buf_size);
} else {
audio_buf_size = audio_size;
}
audio_buf_index = 0;
}
len1 = audio_buf_size - audio_buf_index;
if(len1 > len)
len1 = len;
memcpy(stream, (uint8_t *)audio_buf + audio_buf_index, len1);
len -= len1;
stream += len1;
audio_buf_index += len1;
}
}
int main(int argc, char *argv[]) {
AVFormatContext *pFormatCtx = NULL;
int i, videoStream, audioStream;
AVCodecContext *pCodecCtxOrig = NULL;
AVCodecContext *pCodecCtx = NULL;
AVCodec *pCodec = NULL;
AVFrame *pFrame = NULL;
AVPacket packet;
int frameFinished;
struct SwsContext *sws_ctx = NULL;
AVCodecContext *aCodecCtxOrig = NULL;
AVCodecContext *aCodecCtx = NULL;
AVCodec *aCodec = NULL;
SDL_Overlay *bmp;
SDL_Surface *screen;
SDL_Rect rect;
SDL_Event event;
SDL_AudioSpec wanted_spec, spec;
if(argc < 2) {
fprintf(stderr, "Usage: test <file>\n");
exit(1);
}
av_register_all();
if(SDL_Init(SDL_INIT_VIDEO | SDL_INIT_AUDIO | SDL_INIT_TIMER)) {
fprintf(stderr, "Could not initialize SDL - %s\n", SDL_GetError());
exit(1);
}
if(avformat_open_input(&pFormatCtx, argv[1], NULL, NULL)!=0)
return -1;
if(avformat_find_stream_info(pFormatCtx, NULL)<0)
return -1;
av_dump_format(pFormatCtx, 0, argv[1], 0);
videoStream=-1;
audioStream=-1;
for(i=0; i<pFormatCtx->nb_streams; i++) {
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO &&
videoStream < 0) {
videoStream=i;
}
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_AUDIO &&
audioStream < 0) {
audioStream=i;
}
}
if(videoStream==-1)
return -1;
if(audioStream==-1)
return -1;
aCodecCtxOrig=pFormatCtx->streams[audioStream]->codec;
aCodec = avcodec_find_decoder(aCodecCtxOrig->codec_id);
if(!aCodec) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
aCodecCtx = avcodec_alloc_context3(aCodec);
if(avcodec_copy_context(aCodecCtx, aCodecCtxOrig) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
wanted_spec.freq = aCodecCtx->sample_rate;
wanted_spec.format = AUDIO_S16SYS;
wanted_spec.channels = aCodecCtx->channels;
wanted_spec.silence = 0;
wanted_spec.samples = SDL_AUDIO_BUFFER_SIZE;
wanted_spec.callback = audio_callback;
wanted_spec.userdata = aCodecCtx;
if(SDL_OpenAudio(&wanted_spec, &spec) < 0) {
fprintf(stderr, "SDL_OpenAudio: %s\n", SDL_GetError());
return -1;
}
avcodec_open2(aCodecCtx, aCodec, NULL);
packet_queue_init(&audioq);
SDL_PauseAudio(0);
pCodecCtxOrig=pFormatCtx->streams[videoStream]->codec;
pCodec=avcodec_find_decoder(pCodecCtxOrig->codec_id);
if(pCodec==NULL) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if(avcodec_copy_context(pCodecCtx, pCodecCtxOrig) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(avcodec_open2(pCodecCtx, pCodec, NULL)<0)
return -1;
pFrame=av_frame_alloc();
#ifndef __DARWIN__
screen = SDL_SetVideoMode(pCodecCtx->width, pCodecCtx->height, 0, 0);
#else
screen = SDL_SetVideoMode(pCodecCtx->width, pCodecCtx->height, 24, 0);
#endif
if(!screen) {
fprintf(stderr, "SDL: could not set video mode - exiting\n");
exit(1);
}
bmp = SDL_CreateYUVOverlay(pCodecCtx->width,
pCodecCtx->height,
SDL_YV12_OVERLAY,
screen);
sws_ctx = sws_getContext(pCodecCtx->width,
pCodecCtx->height,
pCodecCtx->pix_fmt,
pCodecCtx->width,
pCodecCtx->height,
PIX_FMT_YUV420P,
SWS_BILINEAR,
NULL,
NULL,
NULL
);
i=0;
while(av_read_frame(pFormatCtx, &packet)>=0) {
if(packet.stream_index==videoStream) {
avcodec_decode_video2(pCodecCtx, pFrame, &frameFinished, &packet);
if(frameFinished) {
SDL_LockYUVOverlay(bmp);
AVPicture pict;
pict.data[0] = bmp->pixels[0];
pict.data[1] = bmp->pixels[2];
pict.data[2] = bmp->pixels[1];
pict.linesize[0] = bmp->pitches[0];
pict.linesize[1] = bmp->pitches[2];
pict.linesize[2] = bmp->pitches[1];
sws_scale(sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, pCodecCtx->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(bmp);
rect.x = 0;
rect.y = 0;
rect.w = pCodecCtx->width;
rect.h = pCodecCtx->height;
SDL_DisplayYUVOverlay(bmp, &rect);
av_free_packet(&packet);
}
} else if(packet.stream_index==audioStream) {
packet_queue_put(&audioq, &packet);
} else {
av_free_packet(&packet);
}
SDL_PollEvent(&event);
switch(event.type) {
case SDL_QUIT:
quit = 1;
SDL_Quit();
exit(0);
break;
default:
break;
}
}
av_frame_free(&pFrame);
avcodec_close(pCodecCtxOrig);
avcodec_close(pCodecCtx);
avcodec_close(aCodecCtxOrig);
avcodec_close(aCodecCtx);
avformat_close_input(&pFormatCtx);
return 0;
}
音讯
现在,我们希望声音在应用程序中播放。SDL还为我们提供了播放声音的方法。SDL_OpenAudio()函数用于打开音频设备本身。它以SDL_AudioSpec结构作为参数,该结构包含有关我们将要播放的音频的所有信息。在展示如何配置它之前,我们首先说明计算机通常如何处理音频。数字音频包含大量样本,每个代表声波的特定含义。声音以特定的采样率记录,该采样率仅指示每个样本的播放速度,并通过每秒的样本数进行测量。大概的采样频率是每秒22,050和44,100个采样,分别是用于无线电和CD的速度。此外,大多数音频可以有多个声道用于立体声或环绕声,因此,例如,如果样本为立体声,则样本一次将为两个。当我们从电影文件中获取数据时,我们不知道将获得多少样本,但是FFmpeg不会产生残缺的样本-这也意味着它也不会分离立体声样本。在SDL中播放音频的方法如下。声音参数已配置:采样频率,通道数等。并同时设置回调函数和用户数据。当我们开始播放声音时,SDL将不断调用此回调函数,并要求它用一定数量的字节填充音频缓冲区。将这些信息放入SDL_AudioSpec结构之后,我们调用SDL_OpenAudio(),它将打开音频设备并向我们返回另一个AudioSpec结构。这些是我们将实际使用的特征-无法保证我们会完全得到我们所要求的!音讯设定
暂时请记住,因为我们还没有有关音频流的任何信息!让我们回到代码中找到视频流并找出音频流是哪个位置的地方:
videoStream=-1;
audioStream=-1;
for(i=0; i < pFormatCtx->nb_streams; i++) {
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO
&&
videoStream < 0) {
videoStream=i;
}
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_AUDIO &&
audioStream < 0) {
audioStream=i;
}
}
if(videoStream==-1)
return -1;
if(audioStream==-1)
return -1;
在这里,我们可以从流中从AVCodecContext获得所需的所有信息,就像我们对视频流所做的那样:AVCodecContext *aCodecCtxOrig;
AVCodecContext *aCodecCtx;
aCodecCtxOrig=pFormatCtx->streams[audioStream]->codec;
如果您还记得的话,在上一课中,我们仍然必须打开音频编解码器本身。这很简单:AVCodec *aCodec;
aCodec = avcodec_find_decoder(aCodecCtx->codec_id);
if(!aCodec) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
aCodecCtx = avcodec_alloc_context3(aCodec);
if(avcodec_copy_context(aCodecCtx, aCodecCtxOrig) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
avcodec_open2(aCodecCtx, aCodec, NULL);
在编解码器的上下文中包含配置音频所需的所有信息:wanted_spec.freq = aCodecCtx->sample_rate;
wanted_spec.format = AUDIO_S16SYS;
wanted_spec.channels = aCodecCtx->channels;
wanted_spec.silence = 0;
wanted_spec.samples = SDL_AUDIO_BUFFER_SIZE;
wanted_spec.callback = audio_callback;
wanted_spec.userdata = aCodecCtx;
if(SDL_OpenAudio(&wanted_spec, &spec) < 0) {
fprintf(stderr, "SDL_OpenAudio: %s\n", SDL_GetError());
return -1;
}
让我们来看一下每个项目:- freq(频率):采样率,如前所述。
- format (): SDL , . «S» «S16SYS» «», 16 , 16 , «SYS» , , . , avcodec_decode_audio2 .
- channels (): .
- silence (): , . 0.
- samples (): , , SDL , . - 512 8192; FFplay, , 1024.
- callback(callback):这里我们传递真实的回调函数。稍后我们将详细讨论回调函数。
- userdata:SDL将为我们的回调提供一个空指针,指向我们想要的任何用户数据。我们想让他知道我们的编解码器环境;稍微降低一点就清楚了。
最后,使用SDL_OpenAudio打开音频。Queue列
这是必要的!现在,我们准备从流中提取音频信息。但是如何处理此信息?我们将不断从电影文件中接收数据包,但是与此同时,SDL将调用回调函数!解决方案将是创建某种全局结构,我们可以在其中插入音频数据包,以便我们的audio_callback可以接收音频数据!因此,这是我们将创建数据包队列的工作。 FFmpeg甚至有一个结构可以帮助解决这个问题:AVPacketList,它只是软件包的链表。这是我们的队列结构:typedef struct PacketQueue {
AVPacketList *first_pkt, *last_pkt;
int nb_packets;
int size;
SDL_mutex *mutex;
SDL_cond *cond;
} PacketQueue;
首先,我们必须指出nb_packets的大小不同-大小是指我们从packet-> size获得的字节的大小。注意,我们有一个互斥锁和一个条件变量。这是因为SDL将音频处理作为单独的流执行。如果我们没有适当地阻塞队列,那么我们确实会破坏我们的数据。让我们看看如何实现队列。每个自重的程序员都应该知道如何创建队列,但是我们还将展示如何执行队列,以便您更轻松地学习SDL函数。首先,我们创建一个函数来初始化队列:void packet_queue_init(PacketQueue *q) {
memset(q, 0, sizeof(PacketQueue));
q->mutex = SDL_CreateMutex();
q->cond = SDL_CreateCond();
}
然后创建一个函数以将对象放入我们的队列中:int packet_queue_put(PacketQueue *q, AVPacket *pkt) {
AVPacketList *pkt1;
if(av_dup_packet(pkt) < 0) {
return -1;
}
pkt1 = av_malloc(sizeof(AVPacketList));
if (!pkt1)
return -1;
pkt1->pkt = *pkt;
pkt1->next = NULL;
SDL_LockMutex(q->mutex);
if (!q->last_pkt)
q->first_pkt = pkt1;
else
q->last_pkt->next = pkt1;
q->last_pkt = pkt1;
q->nb_packets++;
q->size += pkt1->pkt.size;
SDL_CondSignal(q->cond);
SDL_UnlockMutex(q->mutex);
return 0;
}
SDL_LockMutex()阻止队列中的互斥锁,以便我们可以添加一些东西,然后SDL_CondSignal()通过条件变量将信号发送到我们的get函数(如果期望),以告诉它有数据并且可以继续进行进一步操作解锁互斥锁。这是相应的get函数。请注意,如果我们告诉SDL_CondWait()如何执行此操作,它将创建该功能块(即暂停直到获取数据):int quit = 0;
static int packet_queue_get(PacketQueue *q, AVPacket *pkt, int block) {
AVPacketList *pkt1;
int ret;
SDL_LockMutex(q->mutex);
for(;;) {
if(quit) {
ret = -1;
break;
}
pkt1 = q->first_pkt;
if (pkt1) {
q->first_pkt = pkt1->next;
if (!q->first_pkt)
q->last_pkt = NULL;
q->nb_packets--;
q->size -= pkt1->pkt.size;
*pkt = pkt1->pkt;
av_free(pkt1);
ret = 1;
break;
} else if (!block) {
ret = 0;
break;
} else {
SDL_CondWait(q->cond, q->mutex);
}
}
SDL_UnlockMutex(q->mutex);
return ret;
}
如您所见,我们将函数包装在一个永恒的周期中,因此,如果我们要阻塞它,肯定会得到一些数据。我们避免使用SDL_CondWait()函数永远循环。本质上,CondWait所做的只是等待来自SDL_CondSignal()(或SDL_CondBroadcast())的信号,然后继续。但是,好像我们将其捕获在互斥锁中一样-如果我们持有锁,则put函数将无法排队任何东西!但是,SDL_CondWait()对我们的作用还在于取消阻止提供给它的互斥锁,然后在收到信号后立即再次尝试将其锁定。对于每个消防员
您还将看到我们检查了一个全局退出变量,以确保未在程序中设置输出信号(SDL自动处理TERM信号等)。否则,线程将永远继续,并且我们将不得不使用kill -9终止程序: SDL_PollEvent(&event);
switch(event.type) {
case SDL_QUIT:
quit = 1;
我们将退出标志设置为1。我们提供包裹
它仅用于配置队列:PacketQueue audioq;
main() {
...
avcodec_open2(aCodecCtx, aCodec, NULL);
packet_queue_init(&audioq);
SDL_PauseAudio(0);
SDL_PauseAudio()最终启动音频单元。如果不接收数据,它将重现静默状态。但这不会立即发生。因此,我们已经配置了一个队列,现在我们可以将数据包发送给她了。我们进入包装阅读周期:while(av_read_frame(pFormatCtx, &packet)>=0) {
if(packet.stream_index==videoStream) {
....
}
} else if(packet.stream_index==audioStream) {
packet_queue_put(&audioq, &packet);
} else {
av_free_packet(&packet);
}
请注意,排队后我们不会释放包裹。我们稍后将在解密时将其释放。取包
现在,让我们最后使用audio_callback函数从队列中获取数据包。回调应如下所示:void callback(void *userdata, Uint8 *stream, int len)
userdata是我们提供SDL的指针,stream是我们将音频数据写入其中的缓冲区,len是此缓冲区的大小。这是代码:void audio_callback(void *userdata, Uint8 *stream, int len) {
AVCodecContext *aCodecCtx = (AVCodecContext *)userdata;
int len1, audio_size;
static uint8_t audio_buf[(AVCODEC_MAX_AUDIO_FRAME_SIZE * 3) / 2];
static unsigned int audio_buf_size = 0;
static unsigned int audio_buf_index = 0;
while(len > 0) {
if(audio_buf_index >= audio_buf_size) {
audio_size = audio_decode_frame(aCodecCtx, audio_buf,
sizeof(audio_buf));
if(audio_size < 0) {
audio_buf_size = 1024;
memset(audio_buf, 0, audio_buf_size);
} else {
audio_buf_size = audio_size;
}
audio_buf_index = 0;
}
len1 = audio_buf_size - audio_buf_index;
if(len1 > len)
len1 = len;
memcpy(stream, (uint8_t *)audio_buf + audio_buf_index, len1);
len -= len1;
stream += len1;
audio_buf_index += len1;
}
}
实际上,这是一个简单的循环,它从我们编写的另一个函数audio_decode_frame()中提取数据,将结果保存在中间缓冲区中,尝试将len字节写入流中,如果我们仍然没有足够的字节,则尝试接收更多数据,或者将其保存以备后用,如果我们还有东西。大小audio_buf是最大的音频帧大小的1.5倍的FFmpeg会给我们,这给了我们一个很好的利润率。最终音频解密
让我们看一下audio_decode_frame解码器的内部:int audio_decode_frame(AVCodecContext *aCodecCtx, uint8_t *audio_buf,
int buf_size) {
static AVPacket pkt;
static uint8_t *audio_pkt_data = NULL;
static int audio_pkt_size = 0;
static AVFrame frame;
int len1, data_size = 0;
for(;;) {
while(audio_pkt_size > 0) {
int got_frame = 0;
len1 = avcodec_decode_audio4(aCodecCtx, &frame, &got_frame, &pkt);
if(len1 < 0) {
audio_pkt_size = 0;
break;
}
audio_pkt_data += len1;
audio_pkt_size -= len1;
data_size = 0;
if(got_frame) {
data_size = av_samples_get_buffer_size(NULL,
aCodecCtx->channels,
frame.nb_samples,
aCodecCtx->sample_fmt,
1);
assert(data_size <= buf_size);
memcpy(audio_buf, frame.data[0], data_size);
}
if(data_size <= 0) {
continue;
}
return data_size;
}
if(pkt.data)
av_free_packet(&pkt);
if(quit) {
return -1;
}
if(packet_queue_get(&audioq, &pkt, 1) < 0) {
return -1;
}
audio_pkt_data = pkt.data;
audio_pkt_size = pkt.size;
}
}
整个过程实际上是在函数结尾处开始的,我们在此调用packet_queue_get()。我们从队列中取出数据包并保存其中的信息。然后,当我们可以使用该程序包时,我们调用avcodec_decode_audio4(),与它的姐妹函数avcodec_decode_video()非常相似,不同的是,在这种情况下,该程序包可以具有多个帧。因此,您可能需要多次调用它才能从数据包中获取所有数据。收到帧后,我们只需将其复制到我们的音频缓冲区中,确保data_size小于我们的音频缓冲区。另外,请记住有关投射audio_buf的信息正确的类型,因为SDL提供了一个8位的int缓冲区,而FFmpeg提供了一个16位的int缓冲区中的数据。您还应该考虑len1和data_size之间的区别。len1是我们使用的包的大小,data_size是返回的原始数据量。当我们有一些数据时,我们立即返回以找出是否需要从队列中获取更多数据或已经完成。如果我们仍然需要处理包裹,请坚持下去。如果您已完成软件包,请最终将其释放。这就是全部!我们已将音频从主读取循环传输到队列,然后由audio_callback函数读取,它将这些数据传输到SDL,然后SDL传输到您的声卡。继续编译:gcc -o tutorial03 tutorial03.c -lavutil -lavformat -lavcodec -lswscale -lz -lm \
`sdl-config --cflags --libs`
Gip-gip-hooray!视频仍以最大速度传输,但是声音已经按预期播放。这是为什么?是的,因为音频信息具有采样频率-我们以最快的速度输出音频信息,但是音频只是根据其采样频率在此流中播放。对于视频和音频同步,我们几乎已经成熟,但是首先我们需要对该程序进行小的重组。使用单独的流对声音进行排队和播放的方法效果很好:它使代码更易于管理和模块化。在开始将视频与音频同步之前,我们需要简化代码。在下一个系列中,我们将产生控制流!
第4课:多线程← ⇑ →
完整列表tutorial04.c
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <SDL.h>
#include <SDL_thread.h>
#ifdef __MINGW32__
#undef main
#endif
#include <stdio.h>
#include <assert.h>
#include <math.h>
#if LIBAVCODEC_VERSION_INT < AV_VERSION_INT(55,28,1)
#define av_frame_alloc avcodec_alloc_frame
#define av_frame_free avcodec_free_frame
#endif
#define SDL_AUDIO_BUFFER_SIZE 1024
#define MAX_AUDIO_FRAME_SIZE 192000
#define MAX_AUDIOQ_SIZE (5 * 16 * 1024)
#define MAX_VIDEOQ_SIZE (5 * 256 * 1024)
#define FF_REFRESH_EVENT (SDL_USEREVENT)
#define FF_QUIT_EVENT (SDL_USEREVENT + 1)
#define VIDEO_PICTURE_QUEUE_SIZE 1
typedef struct PacketQueue {
AVPacketList *first_pkt, *last_pkt;
int nb_packets;
int size;
SDL_mutex *mutex;
SDL_cond *cond;
} PacketQueue;
typedef struct VideoPicture {
SDL_Overlay *bmp;
int width, height;
int allocated;
} VideoPicture;
typedef struct VideoState {
AVFormatContext *pFormatCtx;
int videoStream, audioStream;
AVStream *audio_st;
AVCodecContext *audio_ctx;
PacketQueue audioq;
uint8_t audio_buf[(AVCODEC_MAX_AUDIO_FRAME_SIZE * 3) / 2];
unsigned int audio_buf_size;
unsigned int audio_buf_index;
AVFrame audio_frame;
AVPacket audio_pkt;
uint8_t *audio_pkt_data;
int audio_pkt_size;
AVStream *video_st;
AVCodecContext *video_ctx;
PacketQueue videoq;
struct SwsContext *sws_ctx;
VideoPicture pictq[VIDEO_PICTURE_QUEUE_SIZE];
int pictq_size, pictq_rindex, pictq_windex;
SDL_mutex *pictq_mutex;
SDL_cond *pictq_cond;
SDL_Thread *parse_tid;
SDL_Thread *video_tid;
char filename[1024];
int quit;
} VideoState;
SDL_Surface *screen;
SDL_mutex *screen_mutex;
VideoState *global_video_state;
void packet_queue_init(PacketQueue *q) {
memset(q, 0, sizeof(PacketQueue));
q->mutex = SDL_CreateMutex();
q->cond = SDL_CreateCond();
}
int packet_queue_put(PacketQueue *q, AVPacket *pkt) {
AVPacketList *pkt1;
if(av_dup_packet(pkt) < 0) {
return -1;
}
pkt1 = av_malloc(sizeof(AVPacketList));
if (!pkt1)
return -1;
pkt1->pkt = *pkt;
pkt1->next = NULL;
SDL_LockMutex(q->mutex);
if (!q->last_pkt)
q->first_pkt = pkt1;
else
q->last_pkt->next = pkt1;
q->last_pkt = pkt1;
q->nb_packets++;
q->size += pkt1->pkt.size;
SDL_CondSignal(q->cond);
SDL_UnlockMutex(q->mutex);
return 0;
}
static int packet_queue_get(PacketQueue *q, AVPacket *pkt, int block)
{
AVPacketList *pkt1;
int ret;
SDL_LockMutex(q->mutex);
for(;;) {
if(global_video_state->quit) {
ret = -1;
break;
}
pkt1 = q->first_pkt;
if (pkt1) {
q->first_pkt = pkt1->next;
if (!q->first_pkt)
q->last_pkt = NULL;
q->nb_packets--;
q->size -= pkt1->pkt.size;
*pkt = pkt1->pkt;
av_free(pkt1);
ret = 1;
break;
} else if (!block) {
ret = 0;
break;
} else {
SDL_CondWait(q->cond, q->mutex);
}
}
SDL_UnlockMutex(q->mutex);
return ret;
}
int audio_decode_frame(VideoState *is, uint8_t *audio_buf, int buf_size) {
int len1, data_size = 0;
AVPacket *pkt = &is->audio_pkt;
for(;;) {
while(is->audio_pkt_size > 0) {
int got_frame = 0;
len1 = avcodec_decode_audio4(is->audio_ctx, &is->audio_frame, &got_frame, pkt);
if(len1 < 0) {
is->audio_pkt_size = 0;
break;
}
data_size = 0;
if(got_frame) {
data_size = av_samples_get_buffer_size(NULL,
is->audio_ctx->channels,
is->audio_frame.nb_samples,
is->audio_ctx->sample_fmt,
1);
assert(data_size <= buf_size);
memcpy(audio_buf, is->audio_frame.data[0], data_size);
}
is->audio_pkt_data += len1;
is->audio_pkt_size -= len1;
if(data_size <= 0) {
continue;
}
return data_size;
}
if(pkt->data)
av_free_packet(pkt);
if(is->quit) {
return -1;
}
if(packet_queue_get(&is->audioq, pkt, 1) < 0) {
return -1;
}
is->audio_pkt_data = pkt->data;
is->audio_pkt_size = pkt->size;
}
}
void audio_callback(void *userdata, Uint8 *stream, int len) {
VideoState *is = (VideoState *)userdata;
int len1, audio_size;
while(len > 0) {
if(is->audio_buf_index >= is->audio_buf_size) {
audio_size = audio_decode_frame(is, is->audio_buf, sizeof(is->audio_buf));
if(audio_size < 0) {
is->audio_buf_size = 1024;
memset(is->audio_buf, 0, is->audio_buf_size);
} else {
is->audio_buf_size = audio_size;
}
is->audio_buf_index = 0;
}
len1 = is->audio_buf_size - is->audio_buf_index;
if(len1 > len)
len1 = len;
memcpy(stream, (uint8_t *)is->audio_buf + is->audio_buf_index, len1);
len -= len1;
stream += len1;
is->audio_buf_index += len1;
}
}
static Uint32 sdl_refresh_timer_cb(Uint32 interval, void *opaque) {
SDL_Event event;
event.type = FF_REFRESH_EVENT;
event.user.data1 = opaque;
SDL_PushEvent(&event);
return 0;
}
static void schedule_refresh(VideoState *is, int delay) {
SDL_AddTimer(delay, sdl_refresh_timer_cb, is);
}
void video_display(VideoState *is) {
SDL_Rect rect;
VideoPicture *vp;
float aspect_ratio;
int w, h, x, y;
int i;
vp = &is->pictq[is->pictq_rindex];
if(vp->bmp) {
if(is->video_ctx->sample_aspect_ratio.num == 0) {
aspect_ratio = 0;
} else {
aspect_ratio = av_q2d(is->video_ctx->sample_aspect_ratio) *
is->video_ctx->width / is->video_ctx->height;
}
if(aspect_ratio <= 0.0) {
aspect_ratio = (float)is->video_ctx->width /
(float)is->video_ctx->height;
}
h = screen->h;
w = ((int)rint(h * aspect_ratio)) & -3;
if(w > screen->w) {
w = screen->w;
h = ((int)rint(w / aspect_ratio)) & -3;
}
x = (screen->w - w) / 2;
y = (screen->h - h) / 2;
rect.x = x;
rect.y = y;
rect.w = w;
rect.h = h;
SDL_LockMutex(screen_mutex);
SDL_DisplayYUVOverlay(vp->bmp, &rect);
SDL_UnlockMutex(screen_mutex);
}
}
void video_refresh_timer(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
if(is->video_st) {
if(is->pictq_size == 0) {
schedule_refresh(is, 1);
} else {
vp = &is->pictq[is->pictq_rindex];
schedule_refresh(is, 40);
video_display(is);
if(++is->pictq_rindex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_rindex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size--;
SDL_CondSignal(is->pictq_cond);
SDL_UnlockMutex(is->pictq_mutex);
}
} else {
schedule_refresh(is, 100);
}
}
void alloc_picture(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
vp = &is->pictq[is->pictq_windex];
if(vp->bmp) {
SDL_FreeYUVOverlay(vp->bmp);
}
SDL_LockMutex(screen_mutex);
vp->bmp = SDL_CreateYUVOverlay(is->video_ctx->width,
is->video_ctx->height,
SDL_YV12_OVERLAY,
screen);
SDL_UnlockMutex(screen_mutex);
vp->width = is->video_ctx->width;
vp->height = is->video_ctx->height;
vp->allocated = 1;
}
int queue_picture(VideoState *is, AVFrame *pFrame) {
VideoPicture *vp;
int dst_pix_fmt;
AVPicture pict;
SDL_LockMutex(is->pictq_mutex);
while(is->pictq_size >= VIDEO_PICTURE_QUEUE_SIZE &&
!is->quit) {
SDL_CondWait(is->pictq_cond, is->pictq_mutex);
}
SDL_UnlockMutex(is->pictq_mutex);
if(is->quit)
return -1;
vp = &is->pictq[is->pictq_windex];
if(!vp->bmp ||
vp->width != is->video_ctx->width ||
vp->height != is->video_ctx->height) {
SDL_Event event;
vp->allocated = 0;
alloc_picture(is);
if(is->quit) {
return -1;
}
}
if(vp->bmp) {
SDL_LockYUVOverlay(vp->bmp);
dst_pix_fmt = PIX_FMT_YUV420P;
pict.data[0] = vp->bmp->pixels[0];
pict.data[1] = vp->bmp->pixels[2];
pict.data[2] = vp->bmp->pixels[1];
pict.linesize[0] = vp->bmp->pitches[0];
pict.linesize[1] = vp->bmp->pitches[2];
pict.linesize[2] = vp->bmp->pitches[1];
sws_scale(is->sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, is->video_ctx->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(vp->bmp);
if(++is->pictq_windex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_windex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size++;
SDL_UnlockMutex(is->pictq_mutex);
}
return 0;
}
int video_thread(void *arg) {
VideoState *is = (VideoState *)arg;
AVPacket pkt1, *packet = &pkt1;
int frameFinished;
AVFrame *pFrame;
pFrame = av_frame_alloc();
for(;;) {
if(packet_queue_get(&is->videoq, packet, 1) < 0) {
break;
}
avcodec_decode_video2(is->video_ctx, pFrame, &frameFinished, packet);
if(frameFinished) {
if(queue_picture(is, pFrame) < 0) {
break;
}
}
av_free_packet(packet);
}
av_frame_free(&pFrame);
return 0;
}
int stream_component_open(VideoState *is, int stream_index) {
AVFormatContext *pFormatCtx = is->pFormatCtx;
AVCodecContext *codecCtx = NULL;
AVCodec *codec = NULL;
SDL_AudioSpec wanted_spec, spec;
if(stream_index < 0 || stream_index >= pFormatCtx->nb_streams) {
return -1;
}
codec = avcodec_find_decoder(pFormatCtx->streams[stream_index]->codec->codec_id);
if(!codec) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
codecCtx = avcodec_alloc_context3(codec);
if(avcodec_copy_context(codecCtx, pFormatCtx->streams[stream_index]->codec) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(codecCtx->codec_type == AVMEDIA_TYPE_AUDIO) {
wanted_spec.freq = codecCtx->sample_rate;
wanted_spec.format = AUDIO_S16SYS;
wanted_spec.channels = codecCtx->channels;
wanted_spec.silence = 0;
wanted_spec.samples = SDL_AUDIO_BUFFER_SIZE;
wanted_spec.callback = audio_callback;
wanted_spec.userdata = is;
if(SDL_OpenAudio(&wanted_spec, &spec) < 0) {
fprintf(stderr, "SDL_OpenAudio: %s\n", SDL_GetError());
return -1;
}
}
if(avcodec_open2(codecCtx, codec, NULL) < 0) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
switch(codecCtx->codec_type) {
case AVMEDIA_TYPE_AUDIO:
is->audioStream = stream_index;
is->audio_st = pFormatCtx->streams[stream_index];
is->audio_ctx = codecCtx;
is->audio_buf_size = 0;
is->audio_buf_index = 0;
memset(&is->audio_pkt, 0, sizeof(is->audio_pkt));
packet_queue_init(&is->audioq);
SDL_PauseAudio(0);
break;
case AVMEDIA_TYPE_VIDEO:
is->videoStream = stream_index;
is->video_st = pFormatCtx->streams[stream_index];
is->video_ctx = codecCtx;
packet_queue_init(&is->videoq);
is->video_tid = SDL_CreateThread(video_thread, is);
is->sws_ctx = sws_getContext(is->video_ctx->width, is->video_ctx->height,
is->video_ctx->pix_fmt, is->video_ctx->width,
is->video_ctx->height, PIX_FMT_YUV420P,
SWS_BILINEAR, NULL, NULL, NULL
);
break;
default:
break;
}
}
int decode_thread(void *arg) {
VideoState *is = (VideoState *)arg;
AVFormatContext *pFormatCtx;
AVPacket pkt1, *packet = &pkt1;
int video_index = -1;
int audio_index = -1;
int i;
is->videoStream=-1;
is->audioStream=-1;
global_video_state = is;
if(avformat_open_input(&pFormatCtx, is->filename, NULL, NULL)!=0)
return -1;
is->pFormatCtx = pFormatCtx;
if(avformat_find_stream_info(pFormatCtx, NULL)<0)
return -1;
av_dump_format(pFormatCtx, 0, is->filename, 0);
for(i=0; i<pFormatCtx->nb_streams; i++) {
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO &&
video_index < 0) {
video_index=i;
}
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_AUDIO &&
audio_index < 0) {
audio_index=i;
}
}
if(audio_index >= 0) {
stream_component_open(is, audio_index);
}
if(video_index >= 0) {
stream_component_open(is, video_index);
}
if(is->videoStream < 0 || is->audioStream < 0) {
fprintf(stderr, "%s: could not open codecs\n", is->filename);
goto fail;
}
for(;;) {
if(is->quit) {
break;
}
if(is->audioq.size > MAX_AUDIOQ_SIZE ||
is->videoq.size > MAX_VIDEOQ_SIZE) {
SDL_Delay(10);
continue;
}
if(av_read_frame(is->pFormatCtx, packet) < 0) {
if(is->pFormatCtx->pb->error == 0) {
SDL_Delay(100);
continue;
} else {
break;
}
}
if(packet->stream_index == is->videoStream) {
packet_queue_put(&is->videoq, packet);
} else if(packet->stream_index == is->audioStream) {
packet_queue_put(&is->audioq, packet);
} else {
av_free_packet(packet);
}
}
while(!is->quit) {
SDL_Delay(100);
}
fail:
if(1){
SDL_Event event;
event.type = FF_QUIT_EVENT;
event.user.data1 = is;
SDL_PushEvent(&event);
}
return 0;
}
int main(int argc, char *argv[]) {
SDL_Event event;
VideoState *is;
is = av_mallocz(sizeof(VideoState));
if(argc < 2) {
fprintf(stderr, "Usage: test <file>\n");
exit(1);
}
av_register_all();
if(SDL_Init(SDL_INIT_VIDEO | SDL_INIT_AUDIO | SDL_INIT_TIMER)) {
fprintf(stderr, "Could not initialize SDL - %s\n", SDL_GetError());
exit(1);
}
#ifndef __DARWIN__
screen = SDL_SetVideoMode(640, 480, 0, 0);
#else
screen = SDL_SetVideoMode(640, 480, 24, 0);
#endif
if(!screen) {
fprintf(stderr, "SDL: could not set video mode - exiting\n");
exit(1);
}
screen_mutex = SDL_CreateMutex();
av_strlcpy(is->filename, argv[1], sizeof(is->filename));
is->pictq_mutex = SDL_CreateMutex();
is->pictq_cond = SDL_CreateCond();
schedule_refresh(is, 40);
is->parse_tid = SDL_CreateThread(decode_thread, is);
if(!is->parse_tid) {
av_free(is);
return -1;
}
for(;;) {
SDL_WaitEvent(&event);
switch(event.type) {
case FF_QUIT_EVENT:
case SDL_QUIT:
is->quit = 1;
SDL_Quit();
return 0;
break;
case FF_REFRESH_EVENT:
video_refresh_timer(event.user.data1);
break;
default:
break;
}
}
return 0;
}
总览
上一次,我们使用SDL音频功能添加了音频支持。SDL启动了一个线程,用于在每次需要声音时为我们定义的函数进行回调。现在,我们将对视频显示执行相同的操作。这使代码更具模块化,并且更易于使用-特别是在您要添加同步的情况下。那么我们从哪里开始呢?请注意,我们的主要功能处理很多事情:它通过事件循环,读取数据包并对视频进行解码。我们要做的是将一切都分成几部分:我们将有一个流负责对数据包进行解码;然后将这些数据包添加到队列中,并由相应的音频和视频流读取。我们已经根据需要调整了音频流。使用视频流会有些困难,因为我们必须确保视频是自己显示的。我们将实际的显示代码添加到主循环中。但是,我们不会在每次执行循环时都显示视频,而是将视频显示集成到事件循环中。想法是解码视频,将接收到的帧保存在另一个队列中,然后创建自己的事件(FF_REFRESH_EVENT),然后将其添加到事件系统中,然后在事件循环中看到该事件时,它将显示队列中的下一帧。以下是发生的情况的便捷ASCII插图:通过事件循环移动视频显示控件的主要原因是,通过SDL_Delay流,我们可以精确控制下一个视频帧何时出现在屏幕上。当我们最终在下一课中同步视频时,只需添加代码即可安排下一次视频更新,以便正确的图像在正确的时间显示在屏幕上。简化代码
让我们清除一下代码。我们拥有有关音频和视频编解码器的所有信息,并且我们将添加队列,缓冲区,上帝知道还有什么。所有这些都是针对某个逻辑单元的,即针对电影。因此,我们打算创建一个包含所有这些信息的大型结构,称为VideoState。typedef struct VideoState {
AVFormatContext *pFormatCtx;
int videoStream, audioStream;
AVStream *audio_st;
AVCodecContext *audio_ctx;
PacketQueue audioq;
uint8_t audio_buf[(AVCODEC_MAX_AUDIO_FRAME_SIZE * 3) / 2];
unsigned int audio_buf_size;
unsigned int audio_buf_index;
AVPacket audio_pkt;
uint8_t *audio_pkt_data;
int audio_pkt_size;
AVStream *video_st;
AVCodecContext *video_ctx;
PacketQueue videoq;
VideoPicture pictq[VIDEO_PICTURE_QUEUE_SIZE];
int pictq_size, pictq_rindex, pictq_windex;
SDL_mutex *pictq_mutex;
SDL_cond *pictq_cond;
SDL_Thread *parse_tid;
SDL_Thread *video_tid;
char filename[1024];
int quit;
} VideoState;
在这里,我们看到了最终的提示。首先,我们了解基本信息-音频和视频流的格式上下文以及索引,以及相应的AVStream对象。然后,我们看到其中一些音频缓冲区已移至此结构。它们(audio_buf,audio_buf_size等)旨在获取有关仍然存在(或丢失)的音频的信息。我们为视频添加了另一个队列和一个缓冲区(将用作队列;为此,我们不需要任何多余的队列)用于已解码的帧(保存为叠加层)。VideoPicture结构-这是我们自己的创作(来到时将看到其中的内容)。您还可以注意到,我们已经为要创建的两个附加流分配了指针,并为退出标志和影片文件名分配了指针。因此,现在我们返回主函数,以了解它如何改变程序。让我们设置我们的VideoState结构:int main(int argc, char *argv[]) {
SDL_Event event;
VideoState *is;
is = av_mallocz(sizeof(VideoState));
av_mallocz()是一个很好的函数,它将为我们分配内存并将其清零。然后我们初始化显示缓冲区(pictq)的锁,因为由于事件循环调用了我们的显示功能-请记住,显示功能将从pictq检索预解码的帧。同时,我们的视频解码器会将信息放入其中-我们不知道谁先到达那里。希望您了解这是经典的比赛条件。因此,我们现在在开始任何主题之前先分发它。让我们还将电影的名称复制到VideoState中:av_strlcpy(is->filename, argv[1], sizeof(is->filename));
is->pictq_mutex = SDL_CreateMutex();
is->pictq_cond = SDL_CreateCond();
av_strlcpy是FFmpeg的函数,除了strncpy之外还执行其他一些边界检查。我们的第一个线程
让我们运行线程并做一些实际的事情:schedule_refresh(is, 40);
is->parse_tid = SDL_CreateThread(decode_thread, is);
if(!is->parse_tid) {
av_free(is);
return -1;
}
schedule_refresh是我们稍后将定义的函数。她所做的是告诉系统在指定的毫秒数后产生FF_REFRESH_EVENT。反过来,当我们在事件队列中看到视频更新功能时,它将调用视频更新功能。但是现在让我们看一下SDL_CreateThread()。SDL_CreateThread()就是这样做的-它产生一个具有对原始进程所有内存的完全访问权限的新线程,并启动我们提供的函数执行的线程。此功能还将传输用户定义的数据。在这种情况下,我们调用decode_thread()并附加我们的VideoState结构。该功能的前半部分没有新内容;它只是打开文件并查找音频和视频流的索引。我们唯一不同的方法是将格式上下文保留在我们的大型结构中。找到流索引后,我们调用定义的另一个函数stream_component_open()。这是一种很自然的分离方式,并且由于我们做了很多类似的事情来设置视频和音频编解码器,因此我们重用了一些代码,使其成为一个函数。Stream_component_open函数()是我们发现编解码器,配置声音参数,在重要结构中保存重要信息并启动音频和视频流的地方。在这里,我们还插入其他参数,例如强制使用编解码器而不是自动检测编解码器等。像这样:int stream_component_open(VideoState *is, int stream_index) {
AVFormatContext *pFormatCtx = is->pFormatCtx;
AVCodecContext *codecCtx;
AVCodec *codec;
SDL_AudioSpec wanted_spec, spec;
if(stream_index < 0 || stream_index >= pFormatCtx->nb_streams) {
return -1;
}
codec = avcodec_find_decoder(pFormatCtx->streams[stream_index]->codec->codec_id);
if(!codec) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
codecCtx = avcodec_alloc_context3(codec);
if(avcodec_copy_context(codecCtx, pFormatCtx->streams[stream_index]->codec) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(codecCtx->codec_type == AVMEDIA_TYPE_AUDIO) {
wanted_spec.freq = codecCtx->sample_rate;
wanted_spec.callback = audio_callback;
wanted_spec.userdata = is;
if(SDL_OpenAudio(&wanted_spec, &spec) < 0) {
fprintf(stderr, "SDL_OpenAudio: %s\n", SDL_GetError());
return -1;
}
}
if(avcodec_open2(codecCtx, codec, NULL) < 0) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
switch(codecCtx->codec_type) {
case AVMEDIA_TYPE_AUDIO:
is->audioStream = stream_index;
is->audio_st = pFormatCtx->streams[stream_index];
is->audio_ctx = codecCtx;
is->audio_buf_size = 0;
is->audio_buf_index = 0;
memset(&is->audio_pkt, 0, sizeof(is->audio_pkt));
packet_queue_init(&is->audioq);
SDL_PauseAudio(0);
break;
case AVMEDIA_TYPE_VIDEO:
is->videoStream = stream_index;
is->video_st = pFormatCtx->streams[stream_index];
is->video_ctx = codecCtx;
packet_queue_init(&is->videoq);
is->video_tid = SDL_CreateThread(video_thread, is);
is->sws_ctx = sws_getContext(is->video_st->codec->width, is->video_st->codec->height,
is->video_st->codec->pix_fmt, is->video_st->codec->width,
is->video_st->codec->height, PIX_FMT_YUV420P,
SWS_BILINEAR, NULL, NULL, NULL
);
break;
default:
break;
}
}
这与我们以前的代码几乎相同,只是现在它已被广泛用于音频和视频。请注意,我们已将大型结构配置为音频回调的用户数据,而不是aCodecCtx。我们还将流本身保存为audio_st和video_st。我们还添加了视频队列并像设置音频队列一样对其进行设置。最重要的是运行视频和音频流。这些位执行此操作: SDL_PauseAudio(0);
break;
is->video_tid = SDL_CreateThread(video_thread, is);
记住上一课的SDL_PauseAudio()。SDL_CreateThread()的使用方式相同。回到我们的video_thread()函数。在此之前,让我们回到解码函数()的第二部分。本质上,这只是一个for循环,它读取一个包并将其放入正确的队列中: for(;;) {
if(is->quit) {
break;
}
if(is->audioq.size > MAX_AUDIOQ_SIZE ||
is->videoq.size > MAX_VIDEOQ_SIZE) {
SDL_Delay(10);
continue;
}
if(av_read_frame(is->pFormatCtx, packet) < 0) {
if((is->pFormatCtx->pb->error) == 0) {
SDL_Delay(100);
continue;
} else {
break;
}
}
if(packet->stream_index == is->videoStream) {
packet_queue_put(&is->videoq, packet);
} else if(packet->stream_index == is->audioStream) {
packet_queue_put(&is->audioq, packet);
} else {
av_free_packet(packet);
}
}
这里没有什么真正的新东西,除了我们现在有一个最大的音频和视频队列大小,并且我们添加了读取错误检查。格式上下文在内部具有ByteIOContext结构,称为pb。ByteIOContext是一种基本上存储有关低级文件的所有信息的结构。在我们的for循环之后,我们拥有所有代码来等待程序的其余部分完成或通知它。这段代码具有指导意义,因为它显示了我们如何推送事件-稍后我们需要一些东西来显示视频: while(!is->quit) {
SDL_Delay(100);
}
fail:
if(1){
SDL_Event event;
event.type = FF_QUIT_EVENT;
event.user.data1 = is;
SDL_PushEvent(&event);
}
return 0;
我们使用SDL常量SDL_USEREVENT获得自定义事件的值。必须将第一个用户事件设置为SDL_USEREVENT,下一个SDL_USEREVENT +1等等。FF_QUIT_EVENT在我们的程序中定义为SDL_USEREVENT +1。如果需要,我们还可以传递用户数据,这里我们将指针传递给大型结构。最后,我们调用SDL_PushEvent()。在事件循环开关中,我们将其放在SDL_QUIT_EVENT部分中我们以前有过的。我们将更详细地了解事件的周期;现在,请确保在我们按FF_QUIT_EVENT时稍后再捕获它并切换退出标志。接收帧:video_thread
准备好编解码器后,就可以开始视频流了。此流从视频队列读取数据包,将视频解码为帧,然后调用queue_picture函数将处理后的帧放入图像队列:int video_thread(void *arg) {
VideoState *is = (VideoState *)arg;
AVPacket pkt1, *packet = &pkt1;
int frameFinished;
AVFrame *pFrame;
pFrame = av_frame_alloc();
for(;;) {
if(packet_queue_get(&is->videoq, packet, 1) < 0) {
break;
}
avcodec_decode_video2(is->video_st->codec, pFrame, &frameFinished, packet);
if(frameFinished) {
if(queue_picture(is, pFrame) < 0) {
break;
}
}
av_free_packet(packet);
}
av_free(pFrame);
return 0;
}
到现在为止,大多数功能都应该被理解。我们只是在此处复制了avcodec_decode_video2函数,只需替换一些参数即可。例如,我们有一个存储在大型结构中的AVStream,因此可以从那里获取编解码器。我们只是继续从视频队列中接收数据包,直到有人告诉我们退出或我们发现错误为止。队列框架
让我们看一下将已解码的pFrame存储在图像队列中的函数。由于我们的图像队列是SDL的叠加层(大概是为了使视频显示功能能够执行尽可能少的计算),因此我们需要将帧转换成它。我们存储在图像队列中的数据是我们创建的结构:typedef struct VideoPicture {
SDL_Overlay *bmp;
int width, height;
int allocated;
} VideoPicture;
我们的大型结构包含这些文件的缓冲区,我们可以在其中存储它们。但是,我们需要自己分发SDL_Overlay(请注意分配的标志,该标志表明我们是否进行了设置)。要使用此队列,我们有两个指针-写索引和读索引。我们还跟踪缓冲区中有多少实际图像。要写入队列,我们首先等待直到清除缓冲区,以便我们有一个位置来存储VideoPicture。然后检查是否在记录索引中设置了覆盖图?如果不是,则需要分配内存。如果窗口大小已更改,我们还必须重新分配缓冲区!int queue_picture(VideoState *is, AVFrame *pFrame) {
VideoPicture *vp;
int dst_pix_fmt;
AVPicture pict;
SDL_LockMutex(is->pictq_mutex);
while(is->pictq_size >= VIDEO_PICTURE_QUEUE_SIZE &&
!is->quit) {
SDL_CondWait(is->pictq_cond, is->pictq_mutex);
}
SDL_UnlockMutex(is->pictq_mutex);
if(is->quit)
return -1;
vp = &is->pictq[is->pictq_windex];
if(!vp->bmp ||
vp->width != is->video_st->codec->width ||
vp->height != is->video_st->codec->height) {
SDL_Event event;
vp->allocated = 0;
alloc_picture(is);
if(is->quit) {
return -1;
}
}
让我们看一下alloc_picture()函数:void alloc_picture(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
vp = &is->pictq[is->pictq_windex];
if(vp->bmp) {
SDL_FreeYUVOverlay(vp->bmp);
}
SDL_LockMutex(screen_mutex);
vp->bmp = SDL_CreateYUVOverlay(is->video_st->codec->width,
is->video_st->codec->height,
SDL_YV12_OVERLAY,
screen);
SDL_UnlockMutex(screen_mutex);
vp->width = is->video_st->codec->width;
vp->height = is->video_st->codec->height;
vp->allocated = 1;
}
您应该认识到函数SDL_CreateYUVOverlay,我们已经将其从主循环移到了本节。该代码现在应该已经相当清楚了。但是,现在有了互斥锁,因为两个线程无法同时将信息写入屏幕!这将不允许我们的alloc_picture函数干扰另一个将显示图片的函数。 (我们将此锁创建为全局变量,并在main()中对其进行了初始化;请参见代码。)请记住,我们将VideoPicture结构的宽度和高度保持不变,因为我们需要确保视频的大小不会由于某些原因而改变。好的,我们已经解决了,我们有叠加的YUV,专用并准备接收图像。让我们回到queue_picture,看看将帧复制到叠加层的代码。这部分您应该熟悉:int queue_picture(VideoState *is, AVFrame *pFrame) {
if(vp->bmp) {
SDL_LockYUVOverlay(vp->bmp);
dst_pix_fmt = PIX_FMT_YUV420P;
pict.data[0] = vp->bmp->pixels[0];
pict.data[1] = vp->bmp->pixels[2];
pict.data[2] = vp->bmp->pixels[1];
pict.linesize[0] = vp->bmp->pitches[0];
pict.linesize[1] = vp->bmp->pitches[2];
pict.linesize[2] = vp->bmp->pitches[1];
sws_scale(is->sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, is->video_st->codec->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(vp->bmp);
if(++is->pictq_windex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_windex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size++;
SDL_UnlockMutex(is->pictq_mutex);
}
return 0;
}
在这里,大多数只是我们之前用来用框架填充YUV叠加层的代码。最后一位只是将我们的值“添加”到队列中。队列正常工作,将值添加到队列中直到队列满为止,并在队列中至少有内容的情况下进行读取。因此,这一切都取决于价值是 - > pictq_size,这就要求我们要阻止它。因此,我们在这里做什么:增加记录指针(如有必要,重新开始),然后阻塞队列并增加其大小。现在,我们的读者将知道有更多有关该队列的信息,如果这使我们的队列已满,则记录器将对此有所了解。视频展示
这就是我们的视频主题!现在,我们已经完成了所有的免费线程,除了一个线程之外-还记得我们很久以前如何调用schedule_refresh()函数吗?看看实际发生了什么:
static void schedule_refresh(VideoState *is, int delay) {
SDL_AddTimer(delay, sdl_refresh_timer_cb, is);
}
SDL_AddTimer()是一个SDL函数,仅在一定的毫秒数后简单地执行对用户定义函数的回调(并在必要时传输一些用户定义的数据)。我们将使用此函数安排视频更新-每次调用它时,它都会设置一个计时器来触发事件,这将导致我们的main()函数调用一个函数,该函数从队列图片中提取帧并显示她! !一句话三个“哪个/哪个/哪个”!因此,我们要做的第一件事-触发此事件。这将我们发送至:static Uint32 sdl_refresh_timer_cb(Uint32 interval, void *opaque) {
SDL_Event event;
event.type = FF_REFRESH_EVENT;
event.user.data1 = opaque;
SDL_PushEvent(&event);
return 0;
}
该活动由我们的老朋友发起。FF_REFRESH_EVENT在此定义为SDL_USEREVENT +1。应该注意的是,当我们返回0时,SDL将停止计时器,因此回调不会再次执行。现在,我们再次调用了FF_REFRESH_EVENT,我们需要在事件循环中对其进行处理:for(;;) {
SDL_WaitEvent(&event);
switch(event.type) {
case FF_REFRESH_EVENT:
video_refresh_timer(event.user.data1);
break;
是什么将我们带到此功能,该功能实际上是从图像队列中提取数据:void video_refresh_timer(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
if(is->video_st) {
if(is->pictq_size == 0) {
schedule_refresh(is, 1);
} else {
vp = &is->pictq[is->pictq_rindex];
schedule_refresh(is, 80);
video_display(is);
if(++is->pictq_rindex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_rindex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size--;
SDL_CondSignal(is->pictq_cond);
SDL_UnlockMutex(is->pictq_mutex);
}
} else {
schedule_refresh(is, 100);
}
}
目前,此功能非常简单:它在有空时处理队列,设置计时器以显示下一个视频帧,调用video_display以在屏幕上实际显示视频,然后增加队列中的计数器,同时减小其大小。您可能会注意到,在此功能中,我们实际上并没有对vp做任何事情,这就是原因:这是未来。但是过了一会儿。当我们开始将视频与音频同步时,我们将使用它来访问时间信息。在这里,请看一下代码中写有“ Timing code go here”的注释的位置。在本节中,我们将找出应该显示下一帧视频的时间,然后在schedule_refresh函数中输入该值。()。目前,我们只输入一个虚拟值80。从技术上讲,您可以猜测并检查该值并为每部电影重新编译,但是:1)一段时间后它将开始变慢,2)相当愚蠢。虽然,将来我们会回到这一点。我们快完成了。剩下要做的只有一件事:显示视频!这是video_display函数:void video_display(VideoState *is) {
SDL_Rect rect;
VideoPicture *vp;
float aspect_ratio;
int w, h, x, y;
int i;
vp = &is->pictq[is->pictq_rindex];
if(vp->bmp) {
if(is->video_st->codec->sample_aspect_ratio.num == 0) {
aspect_ratio = 0;
} else {
aspect_ratio = av_q2d(is->video_st->codec->sample_aspect_ratio) *
is->video_st->codec->width / is->video_st->codec->height;
}
if(aspect_ratio <= 0.0) {
aspect_ratio = (float)is->video_st->codec->width /
(float)is->video_st->codec->height;
}
h = screen->h;
w = ((int)rint(h * aspect_ratio)) & -3;
if(w > screen->w) {
w = screen->w;
h = ((int)rint(w / aspect_ratio)) & -3;
}
x = (screen->w - w) / 2;
y = (screen->h - h) / 2;
rect.x = x;
rect.y = y;
rect.w = w;
rect.h = h;
SDL_LockMutex(screen_mutex);
SDL_DisplayYUVOverlay(vp->bmp, &rect);
SDL_UnlockMutex(screen_mutex);
}
}
由于屏幕可以是任何大小(我们安装了640x480,并且可以通过多种方式对其进行配置,以便用户调整大小),因此您需要动态确定影片的矩形区域应多大。因此,首先您需要找出我们胶片的长宽比,只是宽度除以高度。一些编解码器的样本长宽比是奇数,即一个像素或样本的宽度/高度。由于我们编解码器上下文中的高度和宽度值是以像素为单位进行测量的,因此实际的宽高比等于宽高比乘以样本的宽高比。某些编解码器的长宽比为0,这意味着每个像素的大小仅为1x1。然后我们以这种方式缩放电影使其尽可能地适合屏幕。位反转&-3简单地将值四舍五入到最接近的四倍数。然后将影片居中,并调用 SDL_DisplayYUVOverlay()以确保使用屏幕互斥锁来访问它。这就是全部吗?我们完了吗?您仍然需要重写音频代码才能使用新的 VideoStruct,但是这些都是微不足道的更改,可以在示例代码中看到。我们需要做的最后一件事是更改FFmpeg中内部退出回调函数的回调:VideoState *global_video_state;
int decode_interrupt_cb(void) {
return (global_video_state && global_video_state->quit);
}
在main()中将global_video_state
设置为较大的结构。
就是这样了!我们编译:gcc -o tutorial04 tutorial04.c -lavutil -lavformat -lavcodec -lswscale -lz -lm \
`sdl-config --cflags --libs`
并欣赏电影而无需同步!下一步,我们将最终创建一个真正有效的视频播放器!
第5课:视频同步← ⇑ →
完整列表tutorial05.c
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <SDL.h>
#include <SDL_thread.h>
#ifdef __MINGW32__
#undef main
#endif
#include <stdio.h>
#include <assert.h>
#include <math.h>
#if LIBAVCODEC_VERSION_INT < AV_VERSION_INT(55,28,1)
#define av_frame_alloc avcodec_alloc_frame
#define av_frame_free avcodec_free_frame
#endif
#define SDL_AUDIO_BUFFER_SIZE 1024
#define MAX_AUDIO_FRAME_SIZE 192000
#define MAX_AUDIOQ_SIZE (5 * 16 * 1024)
#define MAX_VIDEOQ_SIZE (5 * 256 * 1024)
#define AV_SYNC_THRESHOLD 0.01
#define AV_NOSYNC_THRESHOLD 10.0
#define FF_REFRESH_EVENT (SDL_USEREVENT)
#define FF_QUIT_EVENT (SDL_USEREVENT + 1)
#define VIDEO_PICTURE_QUEUE_SIZE 1
typedef struct PacketQueue {
AVPacketList *first_pkt, *last_pkt;
int nb_packets;
int size;
SDL_mutex *mutex;
SDL_cond *cond;
} PacketQueue;
typedef struct VideoPicture {
SDL_Overlay *bmp;
int width, height;
int allocated;
double pts;
} VideoPicture;
typedef struct VideoState {
AVFormatContext *pFormatCtx;
int videoStream, audioStream;
double audio_clock;
AVStream *audio_st;
AVCodecContext *audio_ctx;
PacketQueue audioq;
uint8_t audio_buf[(AVCODEC_MAX_AUDIO_FRAME_SIZE * 3) / 2];
unsigned int audio_buf_size;
unsigned int audio_buf_index;
AVFrame audio_frame;
AVPacket audio_pkt;
uint8_t *audio_pkt_data;
int audio_pkt_size;
int audio_hw_buf_size;
double frame_timer;
double frame_last_pts;
double frame_last_delay;
double video_clock;
AVStream *video_st;
AVCodecContext *video_ctx;
PacketQueue videoq;
struct SwsContext *sws_ctx;
VideoPicture pictq[VIDEO_PICTURE_QUEUE_SIZE];
int pictq_size, pictq_rindex, pictq_windex;
SDL_mutex *pictq_mutex;
SDL_cond *pictq_cond;
SDL_Thread *parse_tid;
SDL_Thread *video_tid;
char filename[1024];
int quit;
} VideoState;
SDL_Surface *screen;
SDL_mutex *screen_mutex;
VideoState *global_video_state;
void packet_queue_init(PacketQueue *q) {
memset(q, 0, sizeof(PacketQueue));
q->mutex = SDL_CreateMutex();
q->cond = SDL_CreateCond();
}
int packet_queue_put(PacketQueue *q, AVPacket *pkt) {
AVPacketList *pkt1;
if(av_dup_packet(pkt) < 0) {
return -1;
}
pkt1 = av_malloc(sizeof(AVPacketList));
if (!pkt1)
return -1;
pkt1->pkt = *pkt;
pkt1->next = NULL;
SDL_LockMutex(q->mutex);
if (!q->last_pkt)
q->first_pkt = pkt1;
else
q->last_pkt->next = pkt1;
q->last_pkt = pkt1;
q->nb_packets++;
q->size += pkt1->pkt.size;
SDL_CondSignal(q->cond);
SDL_UnlockMutex(q->mutex);
return 0;
}
static int packet_queue_get(PacketQueue *q, AVPacket *pkt, int block)
{
AVPacketList *pkt1;
int ret;
SDL_LockMutex(q->mutex);
for(;;) {
if(global_video_state->quit) {
ret = -1;
break;
}
pkt1 = q->first_pkt;
if (pkt1) {
q->first_pkt = pkt1->next;
if (!q->first_pkt)
q->last_pkt = NULL;
q->nb_packets--;
q->size -= pkt1->pkt.size;
*pkt = pkt1->pkt;
av_free(pkt1);
ret = 1;
break;
} else if (!block) {
ret = 0;
break;
} else {
SDL_CondWait(q->cond, q->mutex);
}
}
SDL_UnlockMutex(q->mutex);
return ret;
}
double get_audio_clock(VideoState *is) {
double pts;
int hw_buf_size, bytes_per_sec, n;
pts = is->audio_clock;
hw_buf_size = is->audio_buf_size - is->audio_buf_index;
bytes_per_sec = 0;
n = is->audio_ctx->channels * 2;
if(is->audio_st) {
bytes_per_sec = is->audio_ctx->sample_rate * n;
}
if(bytes_per_sec) {
pts -= (double)hw_buf_size / bytes_per_sec;
}
return pts;
}
int audio_decode_frame(VideoState *is, uint8_t *audio_buf, int buf_size, double *pts_ptr) {
int len1, data_size = 0;
AVPacket *pkt = &is->audio_pkt;
double pts;
int n;
for(;;) {
while(is->audio_pkt_size > 0) {
int got_frame = 0;
len1 = avcodec_decode_audio4(is->audio_ctx, &is->audio_frame, &got_frame, pkt);
if(len1 < 0) {
is->audio_pkt_size = 0;
break;
}
data_size = 0;
if(got_frame) {
data_size = av_samples_get_buffer_size(NULL,
is->audio_ctx->channels,
is->audio_frame.nb_samples,
is->audio_ctx->sample_fmt,
1);
assert(data_size <= buf_size);
memcpy(audio_buf, is->audio_frame.data[0], data_size);
}
is->audio_pkt_data += len1;
is->audio_pkt_size -= len1;
if(data_size <= 0) {
continue;
}
pts = is->audio_clock;
*pts_ptr = pts;
n = 2 * is->audio_ctx->channels;
is->audio_clock += (double)data_size /
(double)(n * is->audio_ctx->sample_rate);
return data_size;
}
if(pkt->data)
av_free_packet(pkt);
if(is->quit) {
return -1;
}
if(packet_queue_get(&is->audioq, pkt, 1) < 0) {
return -1;
}
is->audio_pkt_data = pkt->data;
is->audio_pkt_size = pkt->size;
if(pkt->pts != AV_NOPTS_VALUE) {
is->audio_clock = av_q2d(is->audio_st->time_base)*pkt->pts;
}
}
}
void audio_callback(void *userdata, Uint8 *stream, int len) {
VideoState *is = (VideoState *)userdata;
int len1, audio_size;
double pts;
while(len > 0) {
if(is->audio_buf_index >= is->audio_buf_size) {
audio_size = audio_decode_frame(is, is->audio_buf, sizeof(is->audio_buf), &pts);
if(audio_size < 0) {
is->audio_buf_size = 1024;
memset(is->audio_buf, 0, is->audio_buf_size);
} else {
is->audio_buf_size = audio_size;
}
is->audio_buf_index = 0;
}
len1 = is->audio_buf_size - is->audio_buf_index;
if(len1 > len)
len1 = len;
memcpy(stream, (uint8_t *)is->audio_buf + is->audio_buf_index, len1);
len -= len1;
stream += len1;
is->audio_buf_index += len1;
}
}
static Uint32 sdl_refresh_timer_cb(Uint32 interval, void *opaque) {
SDL_Event event;
event.type = FF_REFRESH_EVENT;
event.user.data1 = opaque;
SDL_PushEvent(&event);
return 0;
}
static void schedule_refresh(VideoState *is, int delay) {
SDL_AddTimer(delay, sdl_refresh_timer_cb, is);
}
void video_display(VideoState *is) {
SDL_Rect rect;
VideoPicture *vp;
float aspect_ratio;
int w, h, x, y;
int i;
vp = &is->pictq[is->pictq_rindex];
if(vp->bmp) {
if(is->video_ctx->sample_aspect_ratio.num == 0) {
aspect_ratio = 0;
} else {
aspect_ratio = av_q2d(is->video_ctx->sample_aspect_ratio) *
is->video_ctx->width / is->video_ctx->height;
}
if(aspect_ratio <= 0.0) {
aspect_ratio = (float)is->video_ctx->width /
(float)is->video_ctx->height;
}
h = screen->h;
w = ((int)rint(h * aspect_ratio)) & -3;
if(w > screen->w) {
w = screen->w;
h = ((int)rint(w / aspect_ratio)) & -3;
}
x = (screen->w - w) / 2;
y = (screen->h - h) / 2;
rect.x = x;
rect.y = y;
rect.w = w;
rect.h = h;
SDL_LockMutex(screen_mutex);
SDL_DisplayYUVOverlay(vp->bmp, &rect);
SDL_UnlockMutex(screen_mutex);
}
}
void video_refresh_timer(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
double actual_delay, delay, sync_threshold, ref_clock, diff;
if(is->video_st) {
if(is->pictq_size == 0) {
schedule_refresh(is, 1);
} else {
vp = &is->pictq[is->pictq_rindex];
delay = vp->pts - is->frame_last_pts;
if(delay <= 0 || delay >= 1.0) {
delay = is->frame_last_delay;
}
is->frame_last_delay = delay;
is->frame_last_pts = vp->pts;
ref_clock = get_audio_clock(is);
diff = vp->pts - ref_clock;
sync_threshold = (delay > AV_SYNC_THRESHOLD) ? delay : AV_SYNC_THRESHOLD;
if(fabs(diff) < AV_NOSYNC_THRESHOLD) {
if(diff <= -sync_threshold) {
delay = 0;
} else if(diff >= sync_threshold) {
delay = 2 * delay;
}
}
is->frame_timer += delay;
actual_delay = is->frame_timer - (av_gettime() / 1000000.0);
if(actual_delay < 0.010) {
actual_delay = 0.010;
}
schedule_refresh(is, (int)(actual_delay * 1000 + 0.5));
video_display(is);
if(++is->pictq_rindex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_rindex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size--;
SDL_CondSignal(is->pictq_cond);
SDL_UnlockMutex(is->pictq_mutex);
}
} else {
schedule_refresh(is, 100);
}
}
void alloc_picture(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
vp = &is->pictq[is->pictq_windex];
if(vp->bmp) {
SDL_FreeYUVOverlay(vp->bmp);
}
SDL_LockMutex(screen_mutex);
vp->bmp = SDL_CreateYUVOverlay(is->video_ctx->width,
is->video_ctx->height,
SDL_YV12_OVERLAY,
screen);
SDL_UnlockMutex(screen_mutex);
vp->width = is->video_ctx->width;
vp->height = is->video_ctx->height;
vp->allocated = 1;
}
int queue_picture(VideoState *is, AVFrame *pFrame, double pts) {
VideoPicture *vp;
int dst_pix_fmt;
AVPicture pict;
SDL_LockMutex(is->pictq_mutex);
while(is->pictq_size >= VIDEO_PICTURE_QUEUE_SIZE &&
!is->quit) {
SDL_CondWait(is->pictq_cond, is->pictq_mutex);
}
SDL_UnlockMutex(is->pictq_mutex);
if(is->quit)
return -1;
vp = &is->pictq[is->pictq_windex];
if(!vp->bmp ||
vp->width != is->video_ctx->width ||
vp->height != is->video_ctx->height) {
SDL_Event event;
vp->allocated = 0;
alloc_picture(is);
if(is->quit) {
return -1;
}
}
if(vp->bmp) {
SDL_LockYUVOverlay(vp->bmp);
vp->pts = pts;
dst_pix_fmt = PIX_FMT_YUV420P;
pict.data[0] = vp->bmp->pixels[0];
pict.data[1] = vp->bmp->pixels[2];
pict.data[2] = vp->bmp->pixels[1];
pict.linesize[0] = vp->bmp->pitches[0];
pict.linesize[1] = vp->bmp->pitches[2];
pict.linesize[2] = vp->bmp->pitches[1];
sws_scale(is->sws_ctx, (uint8_t const * const *)pFrame->data,
pFrame->linesize, 0, is->video_ctx->height,
pict.data, pict.linesize);
SDL_UnlockYUVOverlay(vp->bmp);
if(++is->pictq_windex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_windex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size++;
SDL_UnlockMutex(is->pictq_mutex);
}
return 0;
}
double synchronize_video(VideoState *is, AVFrame *src_frame, double pts) {
double frame_delay;
if(pts != 0) {
is->video_clock = pts;
} else {
pts = is->video_clock;
}
frame_delay = av_q2d(is->video_ctx->time_base);
frame_delay += src_frame->repeat_pict * (frame_delay * 0.5);
is->video_clock += frame_delay;
return pts;
}
int video_thread(void *arg) {
VideoState *is = (VideoState *)arg;
AVPacket pkt1, *packet = &pkt1;
int frameFinished;
AVFrame *pFrame;
double pts;
pFrame = av_frame_alloc();
for(;;) {
if(packet_queue_get(&is->videoq, packet, 1) < 0) {
break;
}
if(packet_queue_get(&is->videoq, packet, 1) < 0) {
break;
}
pts = 0;
avcodec_decode_video2(is->video_ctx, pFrame, &frameFinished, packet);
if((pts = av_frame_get_best_effort_timestamp(pFrame)) == AV_NOPTS_VALUE) {
pts = 0;
}
pts *= av_q2d(is->video_st->time_base);
if(frameFinished) {
pts = synchronize_video(is, pFrame, pts);
if(queue_picture(is, pFrame, pts) < 0) {
break;
}
}
av_free_packet(packet);
}
av_frame_free(&pFrame);
return 0;
}
int stream_component_open(VideoState *is, int stream_index) {
AVFormatContext *pFormatCtx = is->pFormatCtx;
AVCodecContext *codecCtx = NULL;
AVCodec *codec = NULL;
SDL_AudioSpec wanted_spec, spec;
if(stream_index < 0 || stream_index >= pFormatCtx->nb_streams) {
return -1;
}
codec = avcodec_find_decoder(pFormatCtx->streams[stream_index]->codec->codec_id);
if(!codec) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
codecCtx = avcodec_alloc_context3(codec);
if(avcodec_copy_context(codecCtx, pFormatCtx->streams[stream_index]->codec) != 0) {
fprintf(stderr, "Couldn't copy codec context");
return -1;
}
if(codecCtx->codec_type == AVMEDIA_TYPE_AUDIO) {
wanted_spec.freq = codecCtx->sample_rate;
wanted_spec.format = AUDIO_S16SYS;
wanted_spec.channels = codecCtx->channels;
wanted_spec.silence = 0;
wanted_spec.samples = SDL_AUDIO_BUFFER_SIZE;
wanted_spec.callback = audio_callback;
wanted_spec.userdata = is;
if(SDL_OpenAudio(&wanted_spec, &spec) < 0) {
fprintf(stderr, "SDL_OpenAudio: %s\n", SDL_GetError());
return -1;
}
is->audio_hw_buf_size = spec.size;
}
if(avcodec_open2(codecCtx, codec, NULL) < 0) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
switch(codecCtx->codec_type) {
case AVMEDIA_TYPE_AUDIO:
is->audioStream = stream_index;
is->audio_st = pFormatCtx->streams[stream_index];
is->audio_ctx = codecCtx;
is->audio_buf_size = 0;
is->audio_buf_index = 0;
memset(&is->audio_pkt, 0, sizeof(is->audio_pkt));
packet_queue_init(&is->audioq);
SDL_PauseAudio(0);
break;
case AVMEDIA_TYPE_VIDEO:
is->videoStream = stream_index;
is->video_st = pFormatCtx->streams[stream_index];
is->video_ctx = codecCtx;
is->frame_timer = (double)av_gettime() / 1000000.0;
is->frame_last_delay = 40e-3;
packet_queue_init(&is->videoq);
is->video_tid = SDL_CreateThread(video_thread, is);
is->sws_ctx = sws_getContext(is->video_ctx->width, is->video_ctx->height,
is->video_ctx->pix_fmt, is->video_ctx->width,
is->video_ctx->height, PIX_FMT_YUV420P,
SWS_BILINEAR, NULL, NULL, NULL
);
break;
default:
break;
}
}
int decode_thread(void *arg) {
VideoState *is = (VideoState *)arg;
AVFormatContext *pFormatCtx;
AVPacket pkt1, *packet = &pkt1;
int video_index = -1;
int audio_index = -1;
int i;
is->videoStream=-1;
is->audioStream=-1;
global_video_state = is;
if(avformat_open_input(&pFormatCtx, is->filename, NULL, NULL)!=0)
return -1;
is->pFormatCtx = pFormatCtx;
if(avformat_find_stream_info(pFormatCtx, NULL)<0)
return -1;
av_dump_format(pFormatCtx, 0, is->filename, 0);
for(i=0; i<pFormatCtx->nb_streams; i++) {
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO &&
video_index < 0) {
video_index=i;
}
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_AUDIO &&
audio_index < 0) {
audio_index=i;
}
}
if(audio_index >= 0) {
stream_component_open(is, audio_index);
}
if(video_index >= 0) {
stream_component_open(is, video_index);
}
if(is->videoStream < 0 || is->audioStream < 0) {
fprintf(stderr, "%s: could not open codecs\n", is->filename);
goto fail;
}
for(;;) {
if(is->quit) {
break;
}
if(is->audioq.size > MAX_AUDIOQ_SIZE ||
is->videoq.size > MAX_VIDEOQ_SIZE) {
SDL_Delay(10);
continue;
}
if(av_read_frame(is->pFormatCtx, packet) < 0) {
if(is->pFormatCtx->pb->error == 0) {
SDL_Delay(100);
continue;
} else {
break;
}
}
if(packet->stream_index == is->videoStream) {
packet_queue_put(&is->videoq, packet);
} else if(packet->stream_index == is->audioStream) {
packet_queue_put(&is->audioq, packet);
} else {
av_free_packet(packet);
}
}
while(!is->quit) {
SDL_Delay(100);
}
fail:
if(1){
SDL_Event event;
event.type = FF_QUIT_EVENT;
event.user.data1 = is;
SDL_PushEvent(&event);
}
return 0;
}
int main(int argc, char *argv[]) {
SDL_Event event;
VideoState *is;
is = av_mallocz(sizeof(VideoState));
if(argc < 2) {
fprintf(stderr, "Usage: test <file>\n");
exit(1);
}
av_register_all();
if(SDL_Init(SDL_INIT_VIDEO | SDL_INIT_AUDIO | SDL_INIT_TIMER)) {
fprintf(stderr, "Could not initialize SDL - %s\n", SDL_GetError());
exit(1);
}
#ifndef __DARWIN__
screen = SDL_SetVideoMode(640, 480, 0, 0);
#else
screen = SDL_SetVideoMode(640, 480, 24, 0);
#endif
if(!screen) {
fprintf(stderr, "SDL: could not set video mode - exiting\n");
exit(1);
}
screen_mutex = SDL_CreateMutex();
av_strlcpy(is->filename, argv[1], sizeof(is->filename));
is->pictq_mutex = SDL_CreateMutex();
is->pictq_cond = SDL_CreateCond();
schedule_refresh(is, 40);
is->parse_tid = SDL_CreateThread(decode_thread, is);
if(!is->parse_tid) {
av_free(is);
return -1;
}
for(;;) {
SDL_WaitEvent(&event);
switch(event.type) {
case FF_QUIT_EVENT:
case SDL_QUIT:
is->quit = 1;
SDL_Quit();
return 0;
break;
case FF_REFRESH_EVENT:
video_refresh_timer(event.user.data1);
break;
default:
break;
}
}
return 0;
}
警告
当我刚刚编写本指南时,我所有的同步代码都来自当时的ffplay.c版本。今天,它是一个完全不同的程序,FFmpeg库(和ffplay.c本身)中的更新已导致根本性的变化。尽管此代码仍然有效,但它已经过时,并且本指南中可以使用许多其他改进。视频如何同步
到现在为止,我们几乎没有用过的电影播放器。是的,它播放视频,是的,它播放音频,但这并不是我们所说的电影。那我们该怎么办呢?PTS和DTS
幸运的是,音频和视频流包含有关播放速度和时间的信息。音频流具有采样率,视频流具有每秒的帧数。但是,如果仅通过计数帧数并乘以帧速率来同步视频,则很有可能它与声音不同步。因此,我们将走另一条路。从流分组可以具有所谓的解码时间戳记(DTS -从d ecoding 吨 IME 小号夯实)和显示时间戳记(PTS -从p resentation 吨 IME 小号夯实)。要了解这两种含义,您需要了解电影的存储方式。某些格式(例如MPEG)使用它们称为B帧的格式(Bed,并且是双向的,England。Bidirectional)。其他两种类型的帧被称为I帧和P帧(我是内部,我 nner,和P手段预测的,p redicted)。I帧包含完整图像。P框取决于先前的I帧和P帧,并且与先前的帧不同,或者您也可以命名-增量。B帧与P 帧相似,但是取决于先前和后续帧中包含的信息!一个帧可能不包含图像本身,而是与其他帧不同的事实-解释了为什么在调用avcodec_decode_video2之后我们可能没有完整的帧。假设我们有一部电影,其中按以下顺序播放4帧:IBBP。然后,我们需要从最后一个P帧中找出信息,然后才能显示之前两个B帧中的任何一个。因此,可以按照与实际显示顺序不匹配的顺序存储帧:IPBB。这就是每个帧的解码时间戳和演示时间戳。解码时间戳告诉我们何时需要解码某些内容,演示时间戳告诉我们何时需要显示某些内容。因此,在这种情况下,我们的流可能如下所示: PTS: 1 4 2 3 DTS: 1 2 3 4流: IPBB通常,仅当正在播放的流包含B帧时,PTS和DTS才不同。当我们从av_read_frame()接收到一个包时,它包含该包内部信息的PTS和DTS值。但是我们真正需要的是我们新解码的原始帧的PTS,在这种情况下,我们知道何时需要显示它。幸运的是,FFmpeg使用av_frame_get_best_effort_timestamp()函数为我们提供了“最佳时间戳” 。同步化
为了依次显示帧,最好知道何时显示特定的视频帧。但是我们到底是怎么做到的呢?这个想法是这样的:在显示框架之后,我们确定何时应该显示下一个框架。然后暂停一下,然后我们将在这段时间后更新视频。如预期的那样,我们检查系统时钟上下一帧的PTS值,以了解我们的等待时间应该多长时间。这种方法有效,但是有两个问题需要解决。首先,问题是,下一个PTS何时生效?您会说,您可以简单地将视频添加到当前的PTS中-原则上您是正确的。但是,某些类型的视频将需要重复帧。这意味着您必须重复当前帧一定次数。这可能会导致程序过早显示下一帧。必须考虑到这一点。第二个问题是,在我们目前编写的程序中,视频和音频正在欣喜地向前冲,直到他们根本不希望同步。如果一切本身都能完美运行,我们就不必担心。但是您的计算机和许多视频文件都不是完美的。因此,我们有三个选项:将音频与视频同步,将视频与音频同步或将音频和视频与外部时钟(例如与您的计算机)同步。现在,我们将视频与音频同步。编码:接收PTS帧
现在,让我们直接写点东西。我们需要在大型结构中添加更多的零件,我们将按照需要的方式进行操作。首先,让我们看一下视频线程。还记得我们在这里收集解码流排队的数据包吗?在这部分代码中,我们需要获取avcodec_decode_video2给我们的帧的PTS 。我们谈论的第一种方法是获取最后处理的数据包的DTS,这很简单: double pts;
for(;;) {
if(packet_queue_get(&is->videoq, packet, 1) < 0) {
break;
}
pts = 0;
len1 = avcodec_decode_video2(is->video_st->codec,
pFrame, &frameFinished, packet);
if(packet->dts != AV_NOPTS_VALUE) {
pts = av_frame_get_best_effort_timestamp(pFrame);
} else {
pts = 0;
}
pts *= av_q2d(is->video_st->time_base);
如果无法确定其值,则将PTS设置为零。好吧,那很容易。技术说明:如您所见,我们将int64用于PTS。这是因为PTS被存储为整数。该值是一个时间戳,与timebb中的时间维度相对应。例如,如果流具有每秒24帧,则42中的PTS将指示应在第42帧应使用的位置使用该帧,但前提是我们每1/24秒钟更换一次帧(当然,不一定如此。事实上)。通过除以帧频,我们可以将该值转换为秒。时基值该流将等于1除以帧速率(对于具有固定帧速率的内容),因此,要获得以秒为单位的PTS,请乘以time_base。进一步编码:PTS的同步和使用
因此,现在我们拥有所有现成的PTS。现在,我们将处理这两个同步问题,这些问题将在后面进行讨论。我们将定义一个sync_ize_video函数,该函数将更新PTS以与所有内容进行同步。最后,此功能还将处理无法获得帧的PTS值的情况。同时,我们需要跟踪何时预期下一帧,以便我们可以正确设置刷新率。我们可以使用内部video_clock值来执行此操作,该值跟踪视频经过了多少时间。我们将此值添加到大型结构中:typedef struct VideoState {
double video_clock;
这是syncnize_video函数,非常清楚:double synchronize_video(VideoState *is, AVFrame *src_frame, double pts) {
double frame_delay;
if(pts != 0) {
is->video_clock = pts;
} else {
pts = is->video_clock;
}
frame_delay = av_q2d(is->video_st->codec->time_base);
frame_delay += src_frame->repeat_pict * (frame_delay * 0.5);
is->video_clock += frame_delay;
return pts;
}
如您所见,我们在此功能中考虑了重复的帧。现在,让我们获得正确的PTS,并通过添加一个新的pts参数使用queue_picture将帧排队:
if(frameFinished) {
pts = synchronize_video(is, pFrame, pts);
if(queue_picture(is, pFrame, pts) < 0) {
break;
}
}
queue_picture中
唯一发生变化的是,我们将此pts值存储在我们排队的VideoPicture结构中。因此,我们必须将pts变量添加到结构中并添加以下代码行:typedef struct VideoPicture {
...
double pts;
}
int queue_picture(VideoState *is, AVFrame *pFrame, double pts) {
... stuff ...
if(vp->bmp) {
... convert picture ...
vp->pts = pts;
... alert queue ...
}
现在,我们将图像与正确的PTS值一起排队,让我们看一下视频更新功能。您可以从上一课中回想起我们只是伪造了它,并安装了80毫秒的更新。好吧,现在我们将要找出应该真正存在的内容。我们的策略是通过简单地测量当前点和上一个点之间的时间来预测下一个PTS的时间。同时,我们需要将视频与音频同步。我们要制造一个音频时钟。:一个内部值,用于跟踪我们正在播放的音频的位置。就像任何mp3播放器上的数字读数一样。由于我们将视频与声音同步,因此视频流使用此值来确定它是太远还是太远。稍后我们将返回实施;现在,假设我们具有get_audio_clock函数这将使我们有时间使用音频时钟。一旦获得此值,如果视频和音频不同步,该怎么办?仅尝试通过搜索或其他方法跳转到正确的软件包是愚蠢的。取而代之的是,我们只需调整为下一次更新计算的值:如果PTS与音频时间相差太远,我们会将估计的延迟加倍。如果PTS比播放时间提前太多,我们将尽快更新。现在,我们已经配置了更新或延迟时间,我们将其与计算机时钟进行比较,而frame_timer仍在运行。此帧计时器总结了我们在电影播放期间的所有估计延迟。换句话说,这个frame_timer-这是指示何时显示下一帧的时间。我们只需向帧计时器添加一个新的延迟,将其与计算机时钟上的时间进行比较,然后使用该值来计划下一次更新。这可能会有些混乱,因此请仔细阅读代码:void video_refresh_timer(void *userdata) {
VideoState *is = (VideoState *)userdata;
VideoPicture *vp;
double actual_delay, delay, sync_threshold, ref_clock, diff;
if(is->video_st) {
if(is->pictq_size == 0) {
schedule_refresh(is, 1);
} else {
vp = &is->pictq[is->pictq_rindex];
delay = vp->pts - is->frame_last_pts;
if(delay <= 0 || delay >= 1.0) {
delay = is->frame_last_delay;
}
is->frame_last_delay = delay;
is->frame_last_pts = vp->pts;
ref_clock = get_audio_clock(is);
diff = vp->pts - ref_clock;
sync_threshold = (delay > AV_SYNC_THRESHOLD) ? delay : AV_SYNC_THRESHOLD;
if(fabs(diff) < AV_NOSYNC_THRESHOLD) {
if(diff <= -sync_threshold) {
delay = 0;
} else if(diff >= sync_threshold) {
delay = 2 * delay;
}
}
is->frame_timer += delay;
actual_delay = is->frame_timer - (av_gettime() / 1000000.0);
if(actual_delay < 0.010) {
actual_delay = 0.010;
}
schedule_refresh(is, (int)(actual_delay * 1000 + 0.5));
video_display(is);
if(++is->pictq_rindex == VIDEO_PICTURE_QUEUE_SIZE) {
is->pictq_rindex = 0;
}
SDL_LockMutex(is->pictq_mutex);
is->pictq_size--;
SDL_CondSignal(is->pictq_cond);
SDL_UnlockMutex(is->pictq_mutex);
}
} else {
schedule_refresh(is, 100);
}
}
我们进行一些检查:首先,我们确保当前PTS和先前PTS之间的延迟是合理的。如果不需要延迟,则音频和视频此时恰好重合,仅使用最后一个延迟。然后我们确保满足同步阈值,因为永远不会发生完美的同步。 FFplay的阈值使用0.01。我们还确保同步阈值不小于PTS值之间的间隔。最后,将最小更新值设置为10毫秒(实际上,似乎他们应该在此处跳过该帧,但是我们不必为此担心)。我们在大型结构中添加了一堆变量,所以不要忘记检查代码。同样,不要忘记在stream_component_open中初始化帧计时器和上一帧的初始延迟: is->frame_timer = (double)av_gettime() / 1000000.0;
is->frame_last_delay = 40e-3;
同步:音频时钟
现在是实现音频时钟的时候了。我们可以在audio_decode_frame函数中更新时间,在其中解码音频。现在请记住,并非每次调用此函数时都总是处理新的包,因此需要在两个区域中更新时钟。首先是获得新软件包的位置:只需将声音时钟安装在PTS软件包上即可。然后,如果数据包有几个帧,我们可以通过计算采样数并将它们乘以每秒给定的采样频率来节省音频播放时间。所以,当我们有包时:
if(pkt->pts != AV_NOPTS_VALUE) {
is->audio_clock = av_q2d(is->audio_st->time_base)*pkt->pts;
}
并且,一旦我们处理了包裹,
pts = is->audio_clock;
*pts_ptr = pts;
n = 2 * is->audio_st->codec->channels;
is->audio_clock += (double)data_size /
(double)(n * is->audio_st->codec->sample_rate);
一些细微的差别:功能模板已更改,现在包括pts_ptr,因此请确保对其进行更改。pts_ptr是我们用来告诉audio_callback音频数据包pts的指针。下次将使用它来同步音频和视频。现在,我们终于可以实现我们的get_audio_clock函数。考虑一下,这并不像获取值是 -> audio_clock那样简单。请注意,每次处理时我们都会设置PTS音频,但是如果您查看audio_callback函数,,将所有数据从音频数据包移到输出缓冲区将需要一些时间。这意味着我们音频时钟中的值可能会过高。因此,我们需要检查必须写多少。这是完整的代码:double get_audio_clock(VideoState *is) {
double pts;
int hw_buf_size, bytes_per_sec, n;
pts = is->audio_clock;
hw_buf_size = is->audio_buf_size - is->audio_buf_index;
bytes_per_sec = 0;
n = is->audio_st->codec->channels * 2;
if(is->audio_st) {
bytes_per_sec = is->audio_st->codec->sample_rate * n;
}
if(bytes_per_sec) {
pts -= (double)hw_buf_size / bytes_per_sec;
}
return pts;
}
您现在应该了解为什么此功能有效;)就这样!我们编译:gcc -o tutorial05 tutorial05.c -lavutil -lavformat -lavcodec -lswscale -lz -lm \
`sdl-config --cflags --libs`
它发生了!您可以在自制播放器上观看电影。在下一课中,我们将研究音频同步,然后学习如何搜索。爱迪生博客上的翻译: