处理传入的HTTP请求时,需要执行大量操作,例如:
- 记录传入的HTTP请求
- 验证HTTP方法
- 身份验证(基本,MS AD等)
- 令牌验证(如有必要)
- 读取传入请求的正文
- 读取传入请求的标头
- 实际处理请求并生成响应
- 安装HSTS Strict-Transport-Security
- 设置外发响应的内容类型
- 记录传出HTTP响应
- 记录传出响应的标题
- 记录传出响应正文
- 错误处理和记录
- 推迟恢复过程以在可能出现紧急情况后进行恢复
这些动作大多数都是典型的,并不取决于请求的类型及其处理。
为了进行生产操作,对于每个操作,都必须提供错误处理和日志记录。
在每个HTTP处理程序中重复所有这些操作效率极低。
即使将所有代码放入单独的子函数中,对于每个HTTP处理程序,您仍然可以获得大约80-100行代码,而无需考虑请求的实际处理和响应的形成。
以下内容描述了我在不使用代码生成器和第三方库的情况下简化Golang中HTTP处理程序编写的方法。
backend Golang
.
, , — , - .
HTTP
UML HTTP EchoHandler.
, , :
- defer . UML — RecoverWrap.func1, .
- . HTTP handler. UML Process — .
- HTTP handler. UML EchoHandler.func1 — .
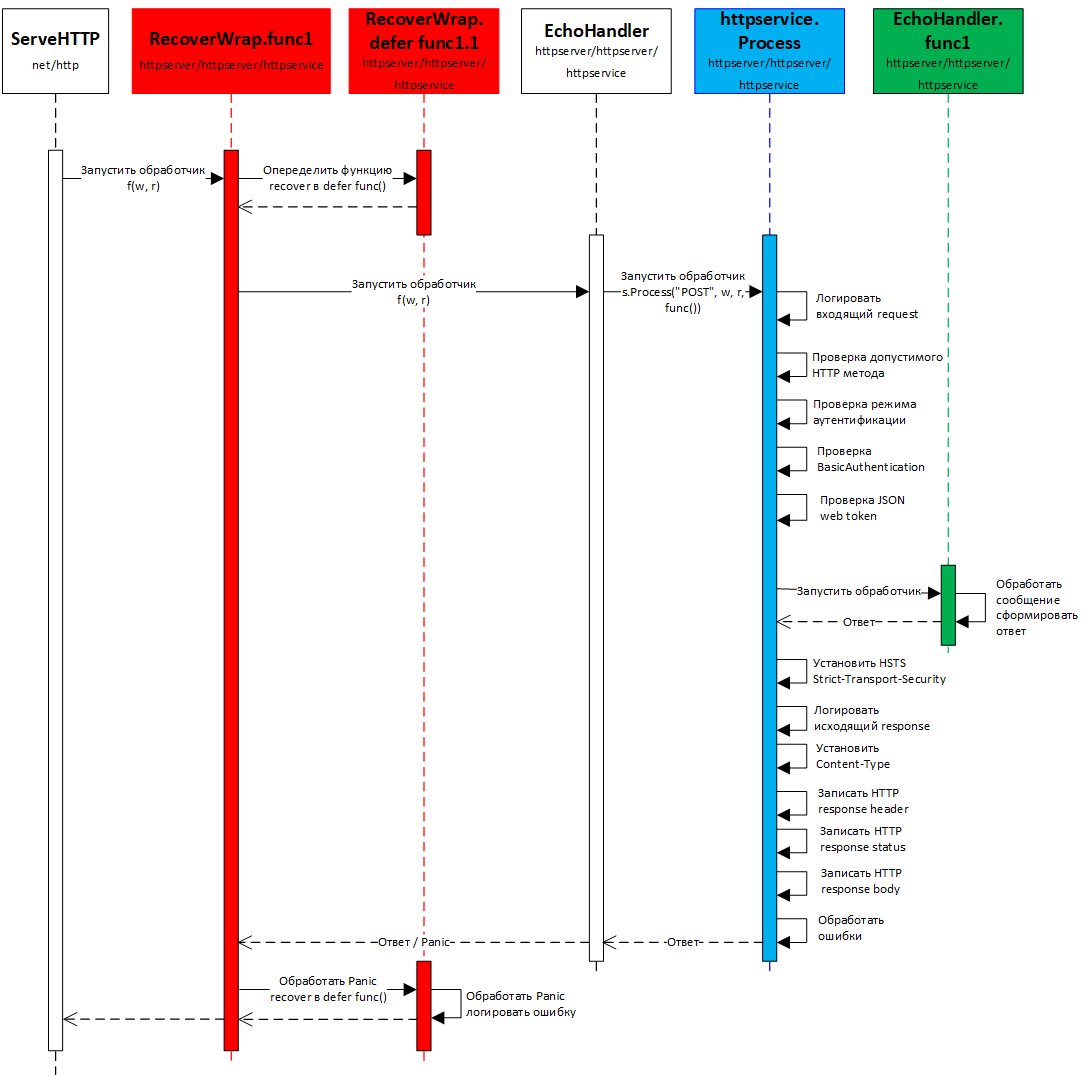
.
HTTP EchoHandler, "" .
, EchoHandler, ( RecoverWrap), EchoHandler.
router.HandleFunc("/echo", service.RecoverWrap(http.HandlerFunc(service.EchoHandler))).Methods("GET")
RecoverWrap .
defer func() EchoHandler.
func (s *Service) RecoverWrap(handlerFunc http.HandlerFunc) http.HandlerFunc {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
defer func() {
var myerr error
r := recover()
if r != nil {
msg := "HTTP Handler recover from panic"
switch t := r.(type) {
case string:
myerr = myerror.New("8888", msg, t)
case error:
myerr = myerror.WithCause("8888", msg, t)
default:
myerr = myerror.New("8888", msg)
}
s.processError(myerr, w, http.StatusInternalServerError, 0)
}
}()
if handlerFunc != nil {
handlerFunc(w, r)
}
})
}
, EchoHandler. , HTTP Process .
func (s *Service) EchoHandler(w http.ResponseWriter, r *http.Request) {
_ = s.Process("POST", w, r, func(requestBuf []byte, reqID uint64) ([]byte, Header, int, error) {
header := Header{}
for key := range r.Header {
header[key] = r.Header.Get(key)
}
return requestBuf, header, http.StatusOK, nil
})
}
HTTP Process. :
- method string — HTTP HTTP
- w http.ResponseWriter, r *http.Request —
- fn func(requestBuf []字节,reqID uint64)([]字节,Header,int,错误)-处理函数本身,它接收传入的请求缓冲区和唯一的HTTP请求号(用于记录目的),返回准备好的传出响应缓冲区,传出响应的标头,HTTP状态和错误。
func (s *Service) Process(method string, w http.ResponseWriter, r *http.Request, fn func(requestBuf []byte, reqID uint64) ([]byte, Header, int, error)) error {
var myerr error
reqID := GetNextRequestID()
if s.logger != nil {
_ = s.logger.LogHTTPInRequest(s.tx, r, reqID)
mylog.PrintfDebugMsg("Logging HTTP in request: reqID", reqID)
}
mylog.PrintfDebugMsg("Check allowed HTTP method: reqID, request.Method, method", reqID, r.Method, method)
if r.Method != method {
myerr = myerror.New("8000", "HTTP method is not allowed: reqID, request.Method, method", reqID, r.Method, method)
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Check authentication method: reqID, AuthType", reqID, s.cfg.AuthType)
if (s.cfg.AuthType == "INTERNAL" || s.cfg.AuthType == "MSAD") && !s.cfg.UseJWT {
mylog.PrintfDebugMsg("JWT is of. Need Authentication: reqID", reqID)
username, password, ok := r.BasicAuth()
if !ok {
myerr := myerror.New("8004", "Header 'Authorization' is not set")
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Get Authorization header: username", username)
if myerr = s.checkAuthentication(username, password); myerr != nil {
mylog.PrintfErrorInfo(myerr)
return myerr
}
}
if s.cfg.UseJWT {
mylog.PrintfDebugMsg("JWT is on. Check JSON web token: reqID", reqID)
cookie, err := r.Cookie("token")
if err != nil {
myerr := myerror.WithCause("8005", "JWT token does not present in Cookie. You have to authorize first.", err)
mylog.PrintfErrorInfo(myerr)
return myerr
}
if myerr = myjwt.CheckJWTFromCookie(cookie, s.cfg.JwtKey); myerr != nil {
mylog.PrintfErrorInfo(myerr)
return myerr
}
}
mylog.PrintfDebugMsg("Reading request body: reqID", reqID)
requestBuf, err := ioutil.ReadAll(r.Body)
if err != nil {
myerr = myerror.WithCause("8001", "Failed to read HTTP body: reqID", err, reqID)
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Read request body: reqID, len(body)", reqID, len(requestBuf))
mylog.PrintfDebugMsg("Calling external function handler: reqID, function", reqID, fn)
responseBuf, header, status, myerr := fn(requestBuf, reqID)
if myerr != nil {
mylog.PrintfErrorInfo(myerr)
return myerr
}
if s.cfg.UseHSTS {
w.Header().Add("Strict-Transport-Security", "max-age=63072000; includeSubDomains")
}
if s.logger != nil {
mylog.PrintfDebugMsg("Logging HTTP out response: reqID", reqID)
_ = s.logger.LogHTTPOutResponse(s.tx, header, responseBuf, status, reqID)
}
mylog.PrintfDebugMsg("Set HTTP response headers: reqID", reqID)
if header != nil {
for key, h := range header {
w.Header().Set(key, h)
}
}
mylog.PrintfDebugMsg("Set HTTP response status: reqID, Status", reqID, http.StatusText(status))
w.WriteHeader(status)
if responseBuf != nil && len(responseBuf) > 0 {
mylog.PrintfDebugMsg("Writing HTTP response body: reqID, len(body)", reqID, len(responseBuf))
respWrittenLen, err := w.Write(responseBuf)
if err != nil {
myerr = myerror.WithCause("8002", "Failed to write HTTP repsonse: reqID", err)
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Written HTTP response: reqID, len(body)", reqID, respWrittenLen)
}
return nil
}