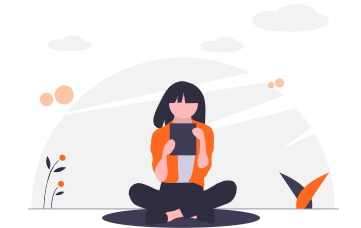
Você pode usar este manual para vários propósitos:
- Para entender o que é o Spring Framework
- Como seus principais recursos funcionam: como injeção de dependência ou Web MVC
- Esta também é uma FAQ exaustiva (Lista de perguntas freqüentes)
Nota: um artigo com ~ 9.000 palavras provavelmente não vale a pena ler em um dispositivo móvel. Marque-o como favorito e volte mais tarde. E mesmo no computador, coma este elefante um pedaço de cada vez :-)
Conteúdo
- Introdução
- Noções básicas de injeção de dependência
- COI de primavera / recipiente de injeção de dependência
- Spring AOP (- )
- Spring
- Spring Web MVC
- Spring Framework
- Spring Framework:
:
. .
Spring
Spring, spring.io, , Spring 21 . !
, Spring , , Spring Boot Spring Data.
, : Spring Framework. ?
, Spring Framework . Spring Boot, Spring Data, Spring Batch — Spring.
:
- Spring Framework . , . Spring Boot, , , Spring Framework .
- ~ 15 , 80% Spring Framework, .
Spring Framework?
:
Spring Framework , (: , , - , RPC, - MVC). Java-.
, ?
, :
.
, , Spring IOC / Dependency Injection. .
?
, Java , . DAO ( ) . , UserDAO.
public class UserDao {
public User findById(Integer id) {
}
}
UserDAO , .
SQL , UserDAO . Java , , , DataSource. , :
import javax.sql.DataSource;
public class UserDao {
public User findById(Integer id) throws SQLException {
try (Connection connection = dataSource.getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where id = ?");
}
}
}
- , UserDao ? , DAO SQL-.
new()
, , . , MySQL UserDAO :
import com.mysql.cj.jdbc.MysqlDataSource;
public class UserDao {
public User findById(Integer id) {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
try (Connection connection = dataSource.getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where id = ?");
return user;
}
}
}
- MySQL, MysqlDataSource url/username/password .
- .
, , , UserDao , findByFirstName.
, . UserDAO , newDataSource.
import com.mysql.cj.jdbc.MysqlDataSource;
public class UserDao {
public User findById(Integer id) {
try (Connection connection = newDataSource().getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where id = ?");
}
}
public User findByFirstName(String firstName) {
try (Connection connection = newDataSource().getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where first_name = ?");
}
}
public DataSource newDataSource() {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
return dataSource;
}
}
- findById newDataSource().
- findByFirstName newDataSource().
- , .
, :
, ProductDAO, SQL? ProductDAO DataSource, UserDAO. , DataSource.
SQL. , DataSource - Java- . . , , , . UserDao, , DAO.
, Application, :
import com.mysql.cj.jdbc.MysqlDataSource;
public enum Application {
INSTANCE;
private DataSource dataSource;
public DataSource dataSource() {
if (dataSource == null) {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
this.dataSource = dataSource;
}
return dataSource;
}
}
UserDAO :
import com.yourpackage.Application;
public class UserDao {
public User findById(Integer id) {
try (Connection connection = Application.INSTANCE.dataSource().getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where id = ?");
}
}
public User findByFirstName(String firstName) {
try (Connection connection = Application.INSTANCE.dataSource().getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where first_name = ?");
}
}
}
:
- UserDAO DataSource, Application . DAO.
- ( , INSTANCE), DataSource.
:
- UserDAO , , → Application.INSTANCE.dataSource().
- , , Application.java, . / ..
(IoC, Inversion of Control)
.
, UserDAO ? , Application.INSTANCE.dataSource(), UserDAO (-) , , , / / ?
, (Inversion of Control).
, UserDAO, .
import javax.sql.DataSource;
public class UserDao {
private DataSource dataSource;
private UserDao(DataSource dataSource) {
this.dataSource = dataSource;
}
public User findById(Integer id) {
try (Connection connection = dataSource.getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where id = ?");
}
}
public User findByFirstName(String firstName) {
try (Connection connection = dataSource.getConnection()) {
PreparedStatement selectStatement = connection.prepareStatement("select * from users where first_name = ?");
}
}
}
- , UserDao , .
- findByX .
UserDao . , , . , « ( ) , ».
, . «new UserService()», UserDao(dataSource).
public class MyApplication {
public static void main(String[] args) {
UserDao userDao = new UserDao(Application.INSTANCE.dataSource());
User user1 = userDao.findById(1);
User user2 = userDao.findById(2);
}
}
, , , , UserDAO , , DataSource .
, - , UserDAO DataSource, , ? : DataSource UserDao?
- , Spring.
Spring IOC / Dependency Injection
, Spring Framework , (. ). , .
ApplicationContext? ?
, (: ), ApplicationContext Spring.
, ( UserDao DataSource , , ):
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import javax.sql.DataSource;
public class MyApplication {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(someConfigClass);
UserDao userDao = ctx.getBean(UserDao.class); // (2)
User user1 = userDao.findById(1);
User user2 = userDao.findById(2);
DataSource dataSource = ctx.getBean(DataSource.class); // (3)
}
}
- Spring ApplicationContext. , , .
- ApplicationContext UserDao, DataSource.
- ApplicationContext , , UserDao.
, ? , , , ApplicationContext !
?
ApplicationContextConfiguration? ApplicationContexts .
someConfigClass AnnotationConfigApplicationContext. :
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class MyApplication {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(someConfigClass);
}
}
, ApplicationContext, , :
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyApplicationContextConfiguration {
@Bean
public DataSource dataSource() {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
return dataSource;
}
@Bean
public UserDao userDao() {
return new UserDao(dataSource());
}
}
- ApplicationContext,
@Configuration
, Application.java . - , DataSource
@Bean
Spring. - , UserDao UserDao, bean- dataSource.
Spring.
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class MyApplication {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(MyApplicationContextConfiguration.class);
UserDao userDao = ctx.getBean(UserDao.class);
// User user1 = userDao.findById(1);
DataSource dataSource = ctx.getBean(DataSource.class);
}
}
, Spring AnnotationConfigApplicationContext , .
AnnotationConfigApplicationContext? ApplicationContext?
Spring ApplicationContext, , XML, Java . ApplicationContext.
MyApplicationContextConfiguration . Java, Spring. ConfigApplicationContext.
ApplicationContext XML, ClassPathXmlApplicationContext.
, Spring , .
@Bean
? Spring Bean?
ApplicationContext . , , UserDao, , DataSource.
, , bean-. : ( Spring) , .
: Spring?
Spring bean scope?
DAO Spring? , bean scope ( ).
- Spring singleton: DAO ?
- Spring prototype: DAO ?
- , : HttpRequest? HttpSession? WebSocket?
, , .
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Scope;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyApplicationContextConfiguration {
@Bean
@Scope("singleton")
public DataSource dataSource() {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
return dataSource;
}
}
(scope annotation) , Spring. , , :
- Scope("singleton") → , .. .
- Scope("prototype") → , - , Spring . ( , , ).
- Scope("session") → HTTP .
- ..
: Spring bean-, bean- (, , , websocket ..).
, ApplicationContexts, Beans & Scopes, , UserDAO DataSource.
Spring Java Config?
bean- ApplicationContext @Bean
Java.
, Spring Java Config, XML, Spring. , :
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyApplicationContextConfiguration {
@Bean
public DataSource dataSource() {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
return dataSource;
}
@Bean
public UserDao userDao() {
return new UserDao(dataSource());
}
}
- : UserDao() dataSource()? Spring ?
@ComponentScan
.
@ComponentScan
?
, , — @ComponentScan
.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan
public class MyApplicationContextConfiguration {
@Bean
public DataSource dataSource() {
MysqlDataSource dataSource = new MysqlDataSource();
dataSource.setUser("root");
dataSource.setPassword("s3cr3t");
dataSource.setURL("jdbc:mysql://localhost:3306/myDatabase");
return dataSource;
}
}
@ComponentScan
.- , UserDAO !
, @ComponentScan
, Spring: Java , , Spring Bean!
, , MyApplicationContextConfiguration com.marcobehler, Spring , , com.marcobehler Spring.
Spring , - Spring? : , @Component
.
@Component
@Autowired
?
@Component
UserDAO.
import javax.sql.DataSource;
import org.springframework.stereotype.Component;
@Component
public class UserDao {
private DataSource dataSource;
private UserDao(DataSource dataSource) {
this.dataSource = dataSource;
}
}
- Spring,
@Bean
, : , , @Component
@ComponentScan
, Spring, , !
( , @Controller
, @Service
@Repository
, , , , @Component
!).
. Spring , DataSource, @Bean
, UserDAO DataSource?
, : @Autowired
. , .
import javax.sql.DataSource;
import org.springframework.stereotype.Component;
import org.springframework.beans.factory.annotation.Autowired;
@Component
public class UserDao {
private DataSource dataSource;
private UserDao(@Autowired DataSource dataSource) {
this.dataSource = dataSource;
}
}
Spring , bean- UserDAO:
- UserDAO
@Component
→ Spring - UserDAO
@Autowired
→ Spring , @Bean
- Spring , NoSuchBeanDefinition .
Autowired
- . Spring ( 4.2) @Autowired
, .
Spring , @Autowired
. .
@Component
public class UserDao {
private DataSource dataSource;
private UserDao(DataSource dataSource) {
this.dataSource = dataSource;
}
}
@Autowired
? , @Autowired
, .
— — .
Field Injection? Setter Injection?
, Spring .
.
import javax.sql.DataSource;
import org.springframework.stereotype.Component;
import org.springframework.beans.factory.annotation.Autowired;
@Component
public class UserDao {
@Autowired
private DataSource dataSource;
}
, Spring .
import javax.sql.DataSource;
import org.springframework.stereotype.Component;
import org.springframework.beans.factory.annotation.Autowired;
@Component
public class UserDao {
private DataSource dataSource;
@Autowired
public void setDataSource(DataSource dataSource) {
this.dataSource = dataSource;
}
}
(, ) , : Spring Bean. , , .
, , , , .
, , (setter) .
:
- , . , .
- : 80% , 10% 10%.
- Spring : / . : .
Spring IoC
, Spring.
, , ApplicationContexts, Beans, , .
, Spring, .
Spring AOP (- )
, , Spring. ApplicationContextConfiguration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyApplicationContextConfiguration {
@Bean
public UserService userService() {
return new UserService();
}
}
- , UserService — , .
Spring:
Spring , @Bean
, , Spring , UserService.
Spring - , UserService. ? ?
Spring
Spring @Bean
, ( ) UserService, .
.
- - UserService, , .

, Spring - Cglib, - (, - JDK): Cglib - . ( , .)
Spring ?
Spring . , , - (: AOP) .
AOP — Spring @Transactional
.
Spring @Transactional
UserService :
import org.springframework.stereotype.Component;
import org.springframework.transaction.annotation.Transactional;
@Component
public class UserService {
@Transactional
public User activateUser(Integer id) {
}
}
- activUser, SQL-, , , .
@Transactional
Spring, / . Spring .
: Spring UserService applicationContext, UserService. , .
, UserService, . , - , , UserService.
ContextConfiguration.
@Configuration
@EnableTransactionManagement
public class MyApplicationContextConfiguration {
@Bean
public UserService userService() {
return new UserService();
}
}
- , Spring: ,
@Transactional
, Cglib . - Spring UserService . Cglib- , , UserService, UserService .
, Spring . - Spring : , — . , , .
Spring Cglib?
AOP Spring. , AspectJ, -. AspectJ .
: Spring AOP AspectJ?
Spring AOP
, - , , Spring AOP, @Transactional
Spring Security, @Secured
. AOP, .
, , @Transactional
Spring, @Transactional
.
Spring
. , Spring. Spring.
, Java HTTP FTP. URL Java .
, ? , - ( , packaged.jar).
, , , , , (URL-, , ).
: Spring. .
import org.springframework.core.io.Resource;
public class MyApplication {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(someConfigClass);
Resource aClasspathTemplate = ctx.getResource("classpath:somePackage/application.properties");
Resource aFileTemplate = ctx.getResource("file:///someDirectory/application.properties");
Resource anHttpTemplate = ctx.getResource("https://marcobehler.com/application.properties");
Resource depends = ctx.getResource("myhost.com/resource/path/myTemplate.txt");
Resource s3Resources = ctx.getResource("s3://myBucket/myFile.txt");
}
}
- , ApplicationContext .
- getResource() applicationContext , classpath:, Spring application classpath.
- getResource() , file:, Spring .
- getResource() , https: ( http), Spring .
- , , . .
- Spring Framework, , Spring Cloud, s3://.
, Spring . :
public interface Resource extends InputStreamSource {
boolean exists();
String getFilename();
File getFile() throws IOException;
InputStream getInputStream() throws IOException;
}
, :
, , , , .
, , Spring: Properties.
Spring Environment?
— (properties), , , ..
.properties, :
Spring environment.
import org.springframework.core.env.Environment;
public class MyApplication {
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(someConfigClass);
Environment env = ctx.getEnvironment();
String databaseUrl = env.getProperty("database.url");
boolean containsPassword = env.containsProperty("database.password");
}
}
- applicationContext (environment) Spring .
- (environment), , , , .
environment?
Spring @PropertySources
?
, Spring . :
- /mydir/application.properties
- classpath:/application-default.properties
(. , «dev» «production», ).
- Spring MVC ServletConfig/Context, JNDI JVM. , , .
, @PropertySources
:
import org.springframework.context.annotation.PropertySources;
import org.springframework.context.annotation.PropertySource;
@Configuration
@PropertySources(
{@PropertySource("classpath:/com/${my.placeholder:default/path}/app.properties"),
@PropertySource("file://myFolder/app-production.properties")})
public class MyApplicationContextConfiguration {
}
, Spring . .
@PropertySource
Spring Spring: , : http://, file://, classpath: ..,
@PropertySources
— , , , ? , .
Spring @Value
bean-, , @Autowired
. @Value
.
import org.springframework.stereotype.Component;
import org.springframework.beans.factory.annotation.Value;
@Component
public class PaymentService {
@Value("${paypal.password}")
private String paypalPassword;
public PaymentService(@Value("${paypal.url}") String paypalUrl) {
this.paypalUrl = paypalUrl;
}
}
@Value
...- .
. , @Value
, Spring () — , .
Spring Web MVC
Spring Web MVC, Spring MVC, - Spring. , , - -. , Spring Boot.
MVC?
MVC, Model-View-Controller , .
HTML-, , , MVC Spring:
- Model () , -. HTML, Java (, ), .
- View () HTML-, HTML-, . , , , .
- Controller ()
@Controller
, HTTP- /account , HTTP- Java, Java HTML.
, Spring, HTTP-, (/account), HTML-, , .
- JSON XML.
, , - -- Java: API Java ( Spring MVC). ).
HttpServlet
MVC: -, HTTP Java, , , HttpServlets ( : , , ). HTTP-, HTTP-.
, Tomcat Jetty. , , URL - . "/*", HTTP- .
:
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class MyServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
if (request.getRequestURI().startsWith("/account")) {
String userId = request.getParameter("userId");
} else if (request.getRequestURI().startsWith("/status")) {
}
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) {
}
}
- Java HttpServlet.
- doGet(), Http GET. "/" GET. , «/status», «/info», «/account» doGet.
- doPost() POST Http. "/" POST. , «/register», «/submit-form», «/password-recovery» doPost().
— , , MVC, .
, MyServlet () HTTP- , URI , , requestParameters .
, Spring . DispatcherServlet.
DispatcherServlet?
Uber- Spring MVC Spring , DispatcherServlet.
DispatcherServlet, HTTP-, Java Controller.
, HTML / JSON / XML, , . ( , DispatcherServlet ).

, .
- Spring HTTP .
- .
- () ( Java ).
Controller .
Controller
, Controller , /account.
/account HTML- , , , ..
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@Controller
public class AccountController {
@GetMapping("/account/{userId}")
public String account(@PathVariable Integer userId) {
return "templates/account";
}
}
, , .
AccountController, @Controller
. Spring: HTTP- , DispatcherServlet .
Java-, account(). , @GetMapping
. DispatcherServlet, , /account/{userId}, . , dispatcherServlet {userId}, !
Java account. , (HTML-). .
HTML (view) Spring Web MVC
Spring MVC , HTML. , , HTML- , , Velocity Freemarker. Spring .
, ():
classpath:/templates/account.vm
<html>
<body>
Hello $user.name, this is your account!
</body>
</html>
HTML-, , $user.name. ? . .
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@Controller
public class AccountController {
@GetMapping("/account/{userId}")
public String account(Model model, @PathVariable Integer userId) {
model.addAttribute("user", userDao.findById(userId));
return "templates/account";
}
}
- Spring "Model" . , , , .. .
- Spring map (), .
- , view, , .
, Spring MVC !
JSON / XML () Spring Web MVC
- HTML, XML JSON. , Spring MVC .
, , Jackson, , Controller , Spring: , Java XML / JSON, , .
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HealthController {
@GetMapping("/health")
@ResponseBody
public HealthStatus health() {
return new HealthStatus();
}
}
@ResponseBody
, Spring, Java- HealthStatus HttpResponse (, XML JSON).- Java- , .
Spring , XML, JSON - ?
(content negotiation) Spring MVC
, , , Spring MVC, Spring MVC.
- Accept, «Accept: application/json» «Accept: application/xml». " ", XML JSON.
- URL , /health.json /health.xml. Spring MVC .
- , /health?Format = json. Spring MVC .
Spring : @ResponseBody
, «JSON». , , JSON? , , HealthStatus JSON. .
, .
HTTP- Spring?
Spring MVC , HTTP — .
, JSON, XML HTTP (Multipart) Fileupload, Spring Java.
HTTP- Spring MVC?
Spring MVC HttpServletResponse — .
HTML, JSON, XML WebSocket. , Java .
Spring MVC?
Spring MVC , , -.
, RequestParams, , , ViewHandlers, RootContexts, , , . , .
.
: Spring Boot
Spring Boot, , @RestController
, , , Spring Boot.
, Spring Boot ( ), , , Spring MVC. :
@Controller
@ResponseBody
public @interface RestController {
}
, Spring MVC @RestController
— , Spring MVC @Controller
Spring MVC @ResponseBody
— , - Spring Boot.
: Spring MVC
Spring MVC — MVC, HTML / JSON / XML - -. Spring, .
: Java-, , , Http- , .
Spring Framework
IoC Spring, Spring Web MVC Spring. . ?
Spring Framework?
Spring Framework , . 20 Spring spring.io. , Spring.
, ?
, , Spring , Java. JDK, . Spring Framework .
: Java API , , , . .
Spring API- Java Mail API, , , , Spring.
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
public class SpringMailSender {
@Autowired
private JavaMailSender mailSender;
public void sendInvoice(User user, File pdf) throws Exception {
MimeMessage mimeMessage = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, true);
helper.setTo("john@rambo.com");
helper.setText("Check out your new invoice!");
FileSystemResource file = new FileSystemResource(pdf);
helper.addAttachment("invoice.pdf", file);
mailSender.send(mimeMessage);
}
}
- , (URL, , ), MailSender, Spring, , .
- Spring , MimeMessageHelper, , , , .
, , Spring Framework , «» Java, , , API Spring.
Spring Framework?
, , Spring. , . .
Spring Framework: FAQ
Spring ?
Spring :
- Spring Boot, Spring . , Spring Boot 2.2.x, Spring 5.2.x ( ).
- Spring , , . Spring 5.2.3.RELEASE, Spring.
- Spring , Spring, ( EOL ) — Spring (. ).
Spring 4.x-5.x, , , Spring 3.x ( : 2009).
Spring? ?
, Spring:

, Spring ~ 17 , 3-4 . , .
- , Spring 4.3 2016 2020 .
- Spring 5.0 5.1 2020 , Spring 5.2, 2019 .
. EOL() ( ) Spring, 5.2, 31 2020 .
, Spring?
, , Spring. spring-context. , Spring.
Maven Gradle, (. : Spring ?) — .jar .
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.3.RELEASE</version>
</dependency>
// Gradle
compile group: 'org.springframework', name: 'spring-context', version: '5.2.3.RELEASE'
Spring ( Spring JDBC JMS) .
Spring, Maven artifactIds . :
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.3.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.3.RELEASE</version>
</dependency>
Spring?
JVM, Spring , , ( ) - Spring 1.0 xml Spring 5.0 ( , , ). , , , 3 5 (. ).
, , Spring Spring ( , , Python 2 3). , , Spring 3 4, Spring 5.
, 7 Spring, :
:
( , ..) . Spring Java, , -, ..
20 Spring.io? -?
, , , , .
: Spring Framework .
Spring Spring Boot?
, , Spring Boot Spring. , Spring Boot, , « » Spring Boot.
Spring .properties , @PropertySource
. JSON REST Web MVC.
, . Spring Boot, , . :
- application.properties .
- Tomcat, Rest .
- / JSON, Maven / Gradle.
Java, @SpringBootApplication
. , Spring Boot Maven / Gradle, .jar, :
java -jar mySpringBootApp.jar
, Spring Boot — , , Spring Framework, .
Spring AOP AspectJ?
Spring Cglib?" Spring AOP . , . , AOP, Spring.
AspectJ, , - . .
Spring AOP AspectJ AOP .
, :
Spring Spring Batch?
Spring Batch — , , .. « 95 CSV- 3 ».
, Spring Framework, .
, , Spring Framework Spring Batch.
Spring Spring Web MVC?
, , Spring Web MVC Spring.
Spring- - DispatcherServlet, @Controller
.
RestControllers ( XML JSON ) HTML-, HTML , Thymeleaf, Velocity Freemarker.
Spring Struts?
: Spring Web MVC Struts?
: Spring Web MVC Struts, , , Spring (. ).
, , Struts 2, , , Spring Web MVC , Spring. Spring Webflow RestControllers Spring Boot.
? Spring XML Java ?
Spring XML. , / Java .
, XML , , Java .
:
- , XML / / Java Config , .
- Spring, XML, Java, .
? ?
, . , : 83% 17%.
Spring: , / , .
, : .
Spring?
, Java:
, Dagger , . Guice , ( Guice Persist).
, , Spring Framework.
, Spring ( Spring Boot Spring Data) , , « Spring ?»
, (~ = ).
Spring Framework — DIY- (~ = ), , (~ = / ) (~ = Web MVC) . ( Java).
(nota: não me pergunte como eu fiz essas comparações;))
Isso é tudo por hoje. Se você tiver alguma dúvida ou sugestão, escreva para marco@marcobehler.com ou deixe um comentário abaixo. Para exercícios práticos, consulte o tutorial do Spring Framework .
Obrigado pela leitura. Auf Wiedersehen.
Agradecimentos
Muito Obrigado:
- Patricio Moschcovich, por ter feito um trabalho incrível ao ler este artigo e apontar vários pequenos erros.
- Maciej Walkowiak por apontar que
@RestController
sempre fez parte do Spring MVC, não do Spring Boot.
(Agradecimentos ao autor do artigo original - aprox. Transl.)