Halo, Habr! Saya sajikan kepada Anda terjemahan artikel "Tandai ini jika Anda baru menggunakan Python (terutama jika Anda belajar sendiri Python)" dalam dua bagian ( 1 , 2 ) dengan tips dan trik sederhana namun bermanfaat dalam Python.
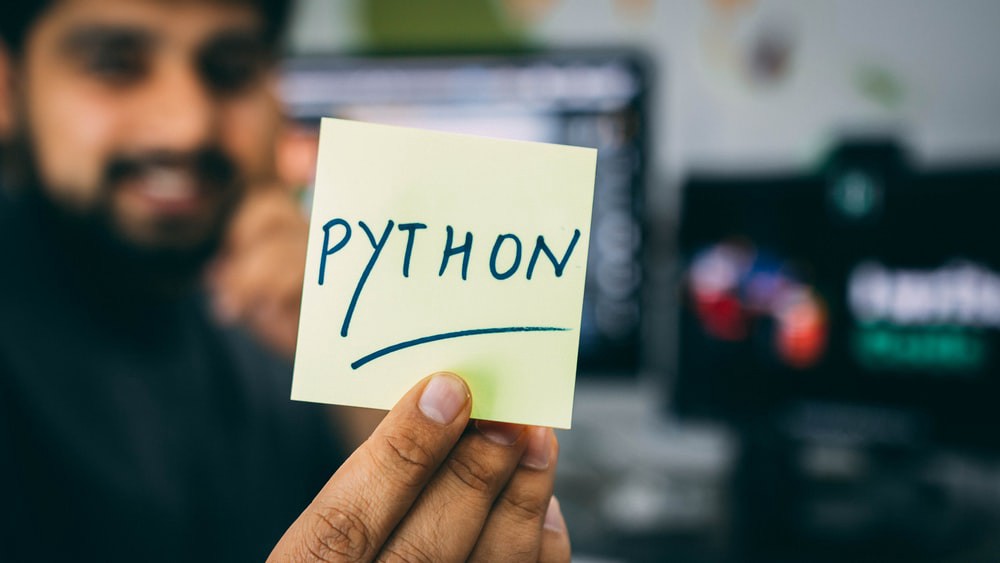
- Python, . . , Python, , .. , Python . , Python , , .
: Python 3.8. - , , , Python.
>>> a = 1
>>> b = 3
>>> a == 1
True
>>> a == 2
False
>>> a == b
False
>>> a > b
False
>>> a <= b
True
>>> if a <= b :
... print('a is less than or equal to b')
...
a is less than or equal to b
. True
False
. if-else
.
>>> def compare(a,b):
... if a> b:
... return a
... else:
... return b
...
return
.
>>> def compare(a, b):
... return a if a > b else b
...
/
>>> arr_list = [1,4,7]
>>> a = arr_list[0]
>>> b = arr_list[1]
>>> c = arr_list[2]
, :
>>> a, b, c = arr_list
>>> a
1
>>> b
4
>>> c
7
>>> arr_list = [1,4,7]
>>> result = []
>>> for i in arr_list:
... result.append(i*2)
...
>>> result
[2, 8, 14]
:
>>> result = [x*2 for x in arr_list]
>>> result
[2, 8, 14]
zip
>>> a = [1,5,8]
>>> b = [3,4,7]
>>> result = []
>>> for i in range(len(a)):
... result.append(a[i] if a[i] < b[i] else b[i])
...
>>> result
[1, 4, 7]
:
>>> result = [min(i) for i in zip(a,b)]
>>> result
[1, 4, 7]
lambda
>>> arr_list= [[1,4], [3,3], [5,7]]
>>> arr_list.sort(key=lambda x: x[1])
>>> arr_list
[[3, 3], [1, 4], [5, 7]]
filter, map
>>> arr_list = [-1, 1, 3, 5]
>>> result = []
>>> for i in arr_list:
... if i > 0:
... result.append(i**2)
...
>>> result
[1, 9, 25]
>>> result = list(map(lambda x: x**2, filter(lambda x: x > 0, arr_list)))
>>> result
[1, 9, 25]
: -, :)
Oleg Kapustin
Kuldeep Pal
.
>>> result = [i**2 for i in arr_list if i > 0]
>>> result
[1, 9, 25]
,
set
, , .
>>> arr_list = [1,4,4,6,9]
>>> len(arr_list) == len(set(arr_list))
False
>>> pi = 3.14159
>>> print('The value of pi is {:.2f}'.format(pi))
The value of pi is 3.14
>>> a, b, c = 1,5,9
>>> print('a is {}; b is {}; c is {}'.format(a,b,c))
a is 1; b is 5; c is 9
>>> print(f'The value of pi is {pi:.2f}')
The value of pi is 3.14
>>> pi
3.14159
>>> print(f'a is {a}; b is {b}; c is {c}')
a is 1; b is 5; c is 9
.
: PyFormat
enumerate
>>> arr_list = [1, 5, 9]
>>> for i in range(len(arr_list)):
... print(f'Index: {i}; Value: {arr_list[i]}')
...
Index: 0; Value: 1
Index: 1; Value: 5
Index: 2; Value: 9
:
>>> for i, j in enumerate(arr_list):
... print(f'Index: {i}; Value: {j}')
...
Index: 0; Value: 1
Index: 1; Value: 5
Index: 2; Value: 9
>>> arr_list = [1,4,6,8,10,11]
>>> a, *b, c = arr_list
>>> a
1
>>> b
[4, 6, 8, 10]
>>> c
11
itertools
>>> str_list = ['A', 'C', 'F']
>>> list(itertools.combinations(str_list,2))
[('A', 'C'), ('A', 'F'), ('C', 'F')]
>>> list(itertools.permutations(str_list,2))
[('A', 'C'), ('A', 'F'), ('C', 'A'), ('C', 'F'), ('F', 'A'), ('F', 'C')]
>>> a = 5
>>> b = 8
>>> temp = a
>>> a = b
>>> b = temp + a
>>> a
8
>>> b
13
. .
>>> a = 5
>>> b = 8
>>> a,b = b, a+b
>>> a
8
>>> b
13
(PS: ? !)
: Evaluation order
>>> str_list = ['This', 'is', 'WYFok']
>>> ' '.join(str_list)
'This is WYFok'
, , join
. .
>>> ans_list = [3,6,9]
>>> 'The answer is '+','.join(map(str,ans_list))
'The answer is 3,6,9'
map
, join
.
: str.join, map
Underscore ()
, , for
, :
>>> for i in range(3):
... print('Hello')
...
Hello
Hello
Hello
, i
for
. i
_
(). ( _
β , Python . , .)
>>> for _ in range(3):
... print('Hello')
...
Hello
Hello
Hello
Dict.keys, Dict.values, Dict.items
>>> teacher_subject = {'Ben':'English','Maria':'Math','Steve':'Science'}
>>> teacher_subject.keys()
dict_keys(['Ben', 'Maria', 'Steve'])
>>> teacher_subject.values()
dict_values(['English', 'Math', 'Science'])
>>> teacher_subject.items()
dict_items([('Ben', 'English'), ('Maria', 'Math'), ('Steve', 'Science')])
keys
values
. items
, . , .
>>> subject_teacher = {y:x for x,y in teacher_subject.items()}
>>> subject_teacher
{'English': 'Ben', 'Math': 'Maria', 'Science': 'Steve'}
: , .
(: zip
)
>>> subject = ['English','Math','Scienc']
>>> teacher = ['Ben','Maria','Steve']
>>> subject_teacher = {f:v for f,v in zip(subject,teacher)}
>>> subject_teacher
{'English': 'Ben', 'Math': 'Maria', 'Scienc': 'Steve'}
: Mapping Types β dict
>>> a = {1,2,3}
>>> b = {1,2,3,4,5}
>>> a<=b
True
>>> a>=b
False
>>> a.union(b)
{1, 2, 3, 4, 5}
>>> a.intersection(b)
{1, 2, 3}
>>> a.difference(b)
set()
>>> b.difference(a)
{4, 5}
: Set
collections.Counter
, . Counter
, .
>>> import collections
>>> arr_list = [1,1,1,1,2,2,2,3,3,5,5,5,7]
>>> c = collections.Counter(arr_list)
>>> c
Counter({1: 4, 2: 3, 5: 3, 3: 2, 7: 1})
>>> type(c)
<class 'collections.Counter'>
>>> c[1]
4
>>> c[6]
0
#
>>> dict(c)
{1: 4, 2: 3, 3: 2, 5: 3, 7: 1}
Informasi Tambahan: collections.Counter
Kesimpulan
Meskipun trik ini cukup sederhana, mereka dapat membantu menghemat waktu dan menyederhanakan kode Anda. Saya harap artikel ini membantu Anda mengetahui cara menggunakan fitur sederhana Python. Pelatihan dan kode yang bagus. Sampai jumpa lagi.