Masalah
Salah satu masalah Java adalah verbositasnya dan jumlah kode standar yang dibutuhkan. Ini adalah pengetahuan umum.
Mari kita lihat kelas Cat sederhana di Jawa. Kami ingin setiap objek Cat memiliki atribut (bidang) berikut:
Cukup sederhana, bukan? Sekarang mari kita lihat kode di Jawa. Untuk mempermudah, mari kita buat kelas kita tidak berubah (tidak bisa diubah) - tanpa setter, kita akan mengonfigurasi semua yang ada di konstruktor kita.
public final class Cat {
private final String name;
private final int numberOfLives;
private final String color;
public Cat(String name, int numberOfLives, String color) {
this.name = name;
this.numberOfLives = numberOfLives;
this.color = color;
}
public String getName() {
return name;
}
public int getNumberOfLives() {
return numberOfLives;
}
public String getColor() {
return color;
}
}
Sudah cukup lama, bukan?
. equals() hashCode().
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Cat cat = (Cat) o;
return numberOfLives == cat.numberOfLives &&
Objects.equals(name, cat.name) &&
Objects.equals(color, cat.color);
}
@Override
public int hashCode() {
return Objects.hash(name, numberOfLives, color);
}
? , toString():
@Override
public String toString() {
return "Cat{" +
"name='" + name + '\'' +
", numberOfLives=" + numberOfLives +
", color='" + color + '\'' +
'}';
}
. ! ( IDE ) . , (, ) .
, :
private final String name;
private final int numberOfLives;
private final String color;
โ , . IDE , , Lombok, .
Java , , Cat. โ , , equals(), hashCode() toString(). , , . โ , . . , hashCode() equals()?
Records
Java 14 , Record, JEP 359: Records (Preview).
50 , :
public record Cat(String name, int numberOfLives, String color) { }
, ?
, โ :
- equals(), hashCode() toString()
, .
public final class Cat extends java.lang.Record {
private final java.lang.String name;
private final int numberOfLives;
private final java.lang.String color;
public Cat(java.lang.String name, int numberOfLives, java.lang.String color) { }
public java.lang.String toString() { }
public final int hashCode() { }
public final boolean equals(java.lang.Object o) { }
public java.lang.String name() { }
public int numberOfLives() { }
public java.lang.String color() { }
}
, Cat. , , โ getColor() color().
java.lang.Record.
equals() , . toString() :
Cat[name=Fluffy, numberOfLives=9, color=White]
, , .
, .
, . , , , . :
public record Cat(String name, int numberOfLives, String color) {
public boolean isAlive() {
return numberOfLives >= 0;
}
}
.
(Custom)
, . , Cat :
Cat cat = new Cat("Fluffy", 9, "White");
, โ , .
, , 9. , , 9 . , .
public record Cat(String name, int numberOfLives, String color) {
public Cat(String name, String color) {
this(name, 9, color);
}
}
. . , . , , :
public record Cat(String name, int numberOfLives, String color) {
public Cat(String name,int numberOfLives, String color) {
if(numberOfLives < 0) {
throw new IllegalArgumentException("Number of lives cannot be less than 0.");
}
if(numberOfLives > 9) {
throw new IllegalArgumentException("Cats cannot have that many lives.");
}
this.name = name;
this.numberOfLives = numberOfLives;
this.color = color;
}
}
, ( ), . , .
public record Cat(String name, int numberOfLives, String color) {
public Cat {
}
}
java.lang.Class , , .
isRecord(). , , - :
Cat cat = new Cat("Fluffy", 9, "White");
if(cat.getClass().isRecord()) {
}
getRecordComponents(). , . java.lang.reflect.RecordComponent. , , :
!
, , Java 14 ( 2/2020).
Preview feature ( )
(Records) Java 14. . ?
VM โ Java SE, , , . JDK ; , Java SE.
JDK , , Java SE , , . , ( ), ( ), .
JDK, . . , , , .
, JDK 14.
IntelliJ IDEA
IntelliJ IDEA Preview feature File โ Project Structure.
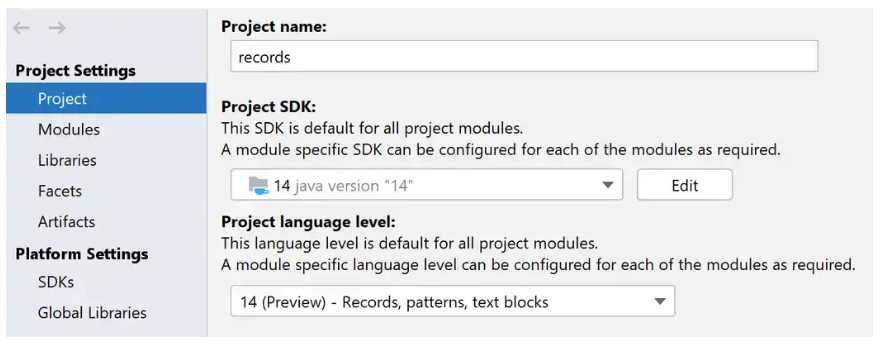
Untuk menggunakan entri dalam IntelliJ IDEA, Anda perlu versi 2020.1 dan yang lebih baru. Per 2/2020, tersedia sebagai membangun Program Akses Awal . IDEA saat ini memiliki dukungan dasar untuk rekaman, tetapi dukungan penuh harus tersedia dalam versi rilis.
Kompilasi manual
Alternatifnya adalah dengan merakit proyek secara manual. Maka Anda perlu memberikan opsi berikut untuk javac:
javac --release 14 --enable-preview ...
Ini untuk kompilasi. Saat runtime, Anda cukup memberikan --enable-preview
java --enable-preview ...
Proyek Maven
Untuk build Maven, Anda dapat menggunakan konfigurasi berikut:
<build>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<release>14</release>
<compilerArgs>
--enable-preview
</compilerArgs>
```14</source>
<target>14</target>
</configuration>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<argLine>--enable-preview</argLine>
</configuration>
</plugin>
<plugin>
<artifactId>maven-failsafe-plugin</artifactId>
<configuration>
<argLine>--enable-preview</argLine>
</configuration>
</plugin>
</plugins>
</build>