Hace aproximadamente un mes, hablando con un desarrollador de aplicaciones en Flutter, hubo un problema al frenar el procesamiento de pequeñas (en decenas de miles) de conjuntos de datos en el teléfono del usuario.
Muchas aplicaciones requieren procesamiento de datos en el teléfono y, además, su sincronización con el backend. Por ejemplo: listas de tareas, listas de cualquier dato (análisis, notas, etc.).
No es nada genial cuando la lista de unos pocos miles de elementos, cuando eliminas uno de ellos y luego escribes en el caché o cuando buscas en el caché, comienza a disminuir.
¡Hay una solucion! Colmena: base noSql escrita en Dart puro, muy rápido. Además, las ventajas de Hive:
- Multiplataforma, ya que no hay dependencias nativas en Dart puro: móvil, escritorio, navegador.
- Alto rendimiento.
- Cifrado fuerte incorporado.
En el artículo, veremos cómo usar Hive y crear una aplicación ToDo simple, que en el próximo artículo se complementará con autorización y sincronización con la nube.
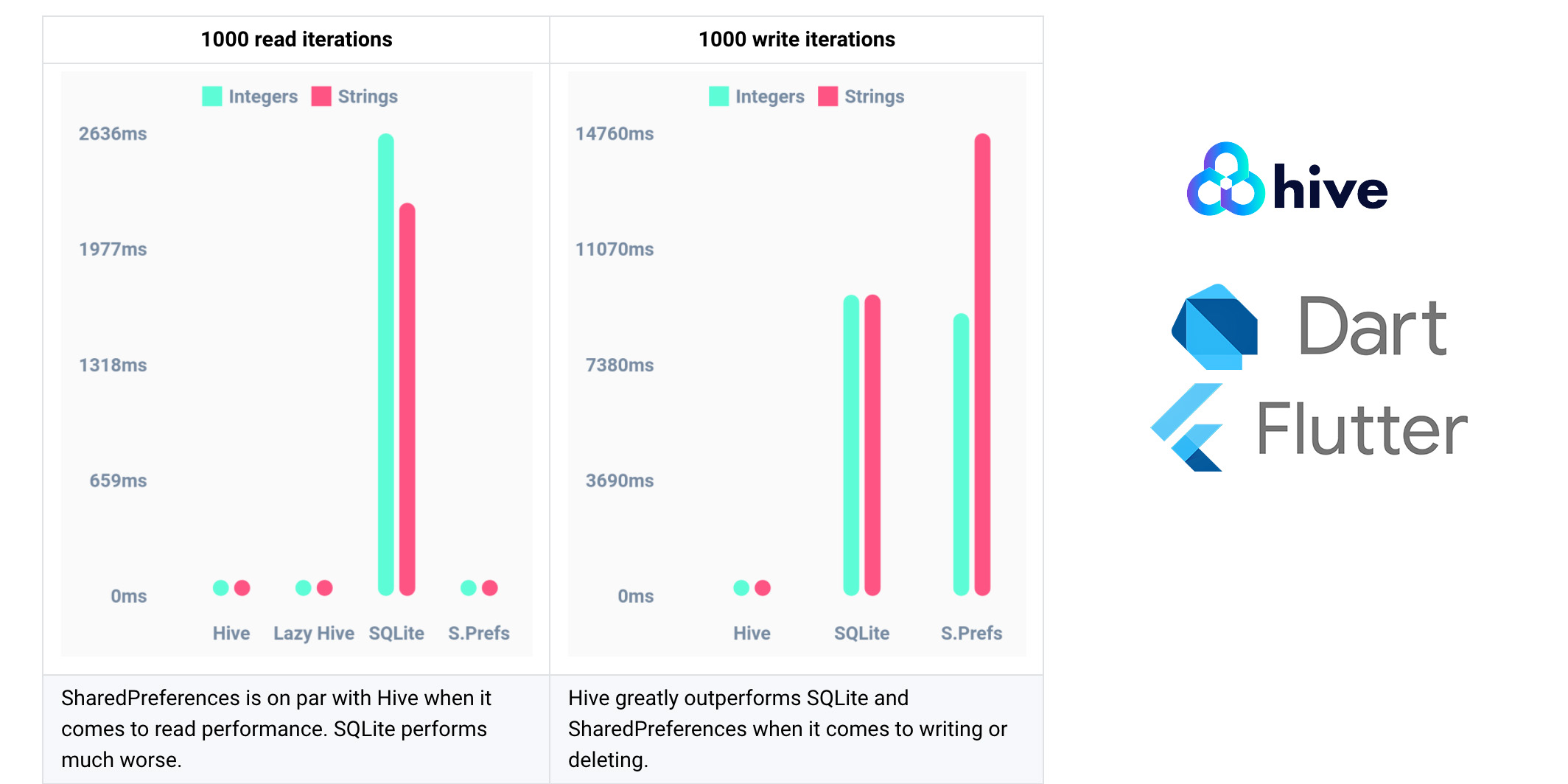
comienzo
Aquí escribiremos dicha aplicación al final del artículo, el código se publica en github .
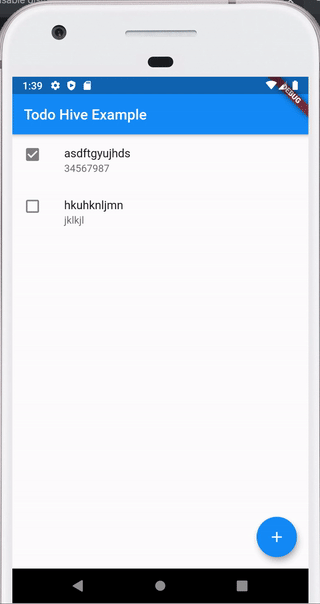
Una de las ventajas de Hive es la muy buena documentación https://docs.hivedb.dev/ : en teoría, todo está ahí. En el artículo, simplemente describiré brevemente cómo trabajar con qué y daré un ejemplo.
Entonces, para conectar la colmena al proyecto, agregue a pubspec.yaml
dependencies:
hive: ^1.4.1+1
hive_flutter: ^0.3.0+2
dev_dependencies:
hive_generator: ^0.7.0+2
build_runner: ^1.8.1
A continuación, inicialice, generalmente al comienzo de la aplicación.
await Hive.initFlutter();
, , . hive_flutter: ^0.3.0+2 — Flutter.
, Hive List, Map, DateTime, BigInt Uint8List.
, TypeAdapters. https://docs.hivedb.dev/#/custom-objects/type_adapters ( hive_generator: ^0.7.0+2).
class Todo {
bool complete;
String id;
String note;
String task;
}
id — typeId: 0
import 'package:hive/hive.dart';
part 'todo.g.dart';
@HiveType(typeId: 0)
class Todo {
@HiveField(0)
bool complete;
@HiveField(1)
String id;
@HiveField(2)
String note;
@HiveField(3)
String task;
}
, , . Hive.
flutter packages pub run build_runner build --delete-conflicting-outputs
todo.g.dart
\ Hive.
HiveObject — Todo HiveObject
class Todo extends HiveObject {
2 save() delete(), .
— Box
hive (box). , , ..
Box . , ( ).
var todoBox = await Hive.openBox<Todo>('box_for_todo');
read / write .
, :
,
var stringBox = await Hive.openBox<String>('name_of_the_box');
stringBox.put('key1', 'value1');
print(stringBox.get('key1')); // value1
stringBox.put('key2', 'value2');
print(stringBox.get('key2')); // value2
+
List. autoinrement.
: getAt(), putAt() and deleteAt()
add() .
stringBox.put('value1');
stringBox.put('value2');
stringBox.put('value3');
print(stringBox.getAt(1)); //value2
? , Hive. Listeners , . ( , ), Listeners . await .
,
var stringBox = await Hive.box<String>('name_of_the_box');
, singleton.
, , , ,
var stringBox = await Hive.openBox<String>('name_of_the_box');
, :
/// Returns a previously opened box.
Box<E> box<E>(String name);
/// Returns a previously opened lazy box.
LazyBox<E> lazyBox<E>(String name);
/// Checks if a specific box is currently open.
bool isBoxOpen(String name);
/// Closes all open boxes.
Future<void> close();
Devhack , Flutter — open source, , , .
LazyBox —
, . . , - .
, lazily
var lazyBox = await Hive.openLazyBox('myLazyBox');
var value = await lazyBox.get('lazyVal');
Hive . , Hive .
Hive AES-256 .
256-
var key = Hive.generateSecureKey();
Fortuna random number generator.
var encryptedBox = await Hive.openBox('vaultBox', encryptionKey: key);
encryptedBox.put('secret', 'Hive is cool');
print(encryptedBox.get('secret'));
:
- , plaintext.
- flutter_secure_storage .
- , , .
, , .
,
var box = Hive.box('myBox');
await box.compact();
await box.close();
todo_hive_example
, , , .
, .
:
:
1 —
, , ( +.
, .
.
(, Dart (extensions)), /hive_flutter-0.3.0+2/lib/src/box_extensions.dart
/// Flutter extensions for boxes.
extension BoxX<T> on Box<T> {
/// Returns a [ValueListenable] which notifies its listeners when an entry
/// in the box changes.
///
/// If [keys] filter is provided, only changes to entries with the
/// specified keys notify the listeners.
ValueListenable<Box<T>> listenable({List<dynamic> keys}) =>
_BoxListenable(this, keys?.toSet());
}
, .
, ,
body: ValueListenableBuilder(
valueListenable: Hive.box<Todo>(HiveBoxes.todo).listenable(),
builder: (context, Box<Todo> box, _) {
if (box.values.isEmpty)
return Center(
child: Text("Todo list is empty"),
);
return ListView.builder(
itemCount: box.values.length,
itemBuilder: (context, index) {
Todo res = box.getAt(index);
return ListTile(
title: Text(res.task),
subtitle: Text(res.note),
);
},
);
},
),
. + .
, +.
Add , .
void _onFormSubmit() {
Box<Todo> contactsBox = Hive.box<Todo>(HiveBoxes.todo);
contactsBox.add(Todo(task: task, note: note));
Navigator.of(context).pop();
}
, , Todo. Hive.
github .
2 — \
. , class Todo extends HiveObject
leading: res.complete
? Icon(Icons.check_box)
: Icon(Icons.check_box_outline_blank),
onTap: () {
res.complete = !res.complete;
res.save();
});
github .
3 — —
. dismissable .
background: Container(color: Colors.red),
key: Key(res.id),
onDismissed: (direction) {
res.delete();
},
, , .
github — https://github.com/awaik/todo_hive_example
To be continued:
- BLoC. , , Apple Id.
- Firebase GraphQl . ( ).