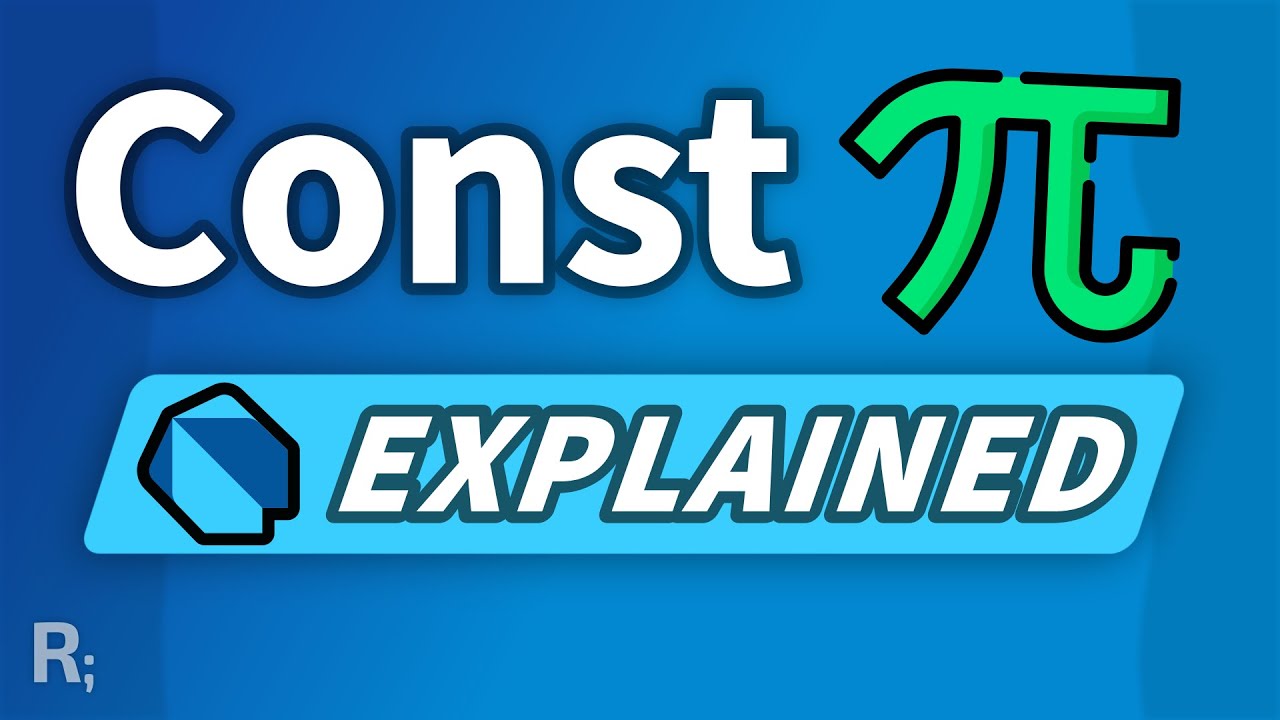
Constants are not just a strange version of final
variables that will haunt you in a dream with all the errors associated with them. Compile-time
constants are a good way to improve the performance of your application, without creating the same object several times, and so to speak, “pre-creating” objects at compile time.
const or final?
, , . , , const
final
. .
void run() {
const myConstNumber = 5;
final myFinalNumber = 5;
myConstNumber = 42;
myFinalNumber = 42;
}
final
, const
, . , const
.
class MyClass {
final int myFinalField;
const int myConstField;
MyClass(this.myFinalField, this.myConstField);
}
, , final
, const
. , , (. ). ...
Dart . , "hello"
, , 3.14
, , .
, .
void run() {
const myList = [1, 2, 3];
const myMap = {'one': 1};
const mySet = {1, 2, 3};
}
: , if, spread
, .
, const
final
, , . Dart , , . .

, , , . , .
void run() {
final finalVariable = 123456;
const constVariable = 123456;
const notWorking = finalVariable;
const working = constVariable;
}
, – , , , ...
Flutter const
, , EdgeInsets, . - , .
const EdgeInsets.all(8)
, . , const
, .
, const
. : final
.
class MyConstClass {
final int field1;
final List<String> field2;
const MyConstClass(this.field1, this.field2);
}
void run() {
const constVariable = 123456;
const x = MyConstClass(constVariable, ['hello', 'there']);
}
, , const
.
class MyWannabeConstClass {
final Future<int> field;
const MyWannabeConstClass(this.field);
}
void run() {
const x = MyWannabeConstClass(Future.value(123));
}
Dart , , , ""? , . const
, . .
void run() {
final implicitNew = MyWannabeConstClass(Future.value(123));
final explicitNew = new MyWannabeConstClass(Future.value(123));
}
const
, , , Flutter .
, const
final
.
, new
, const
.
void run() {
const implicitConst = MyConstClass(1);
const explicitConst = const MyConstClass(1);
}
const
, , , .
void run() {
final regularVariable = const MyConstClass(1);
}
Conclusion
Hopefully this guide has been able to clarify the meaning of const
constructors and, in general, constants in Dart. Try to use const
it where possible, and you will make small performance improvements in your applications line by line. And you know how it happens with small improvements - they give an overall result.