Working with strings can be difficult due to the fact that it involves solving many diverse problems. For example, to simply cast a string to a camel style, you need a few lines of code:function camelize(str) {
return str.replace(/(?:^\w|[A-Z]|\b\w|\s+)/g, function(match, index) {
if (+match === 0) re
turn "";
return index === 0 ? match.toLowerCase() : match.toUpperCase();
});
}
This piece of code, by the way, in the role of answering the question of bringing strings to the “camel” style, gathered the most votes on Stack Overflow. But even he is not able to correctly process, for example, such a line:---Foo---bAr---
The result of processing a string --- Foo --- bAr ---But what if these strings, nevertheless, need to be processed? You can fix this example, or you can resort to the help of specialized libraries. They simplify the implementation of complex algorithms and, in addition, provide the programmer with tools that are much more flexible and versatile than, say, the above example. This may well mean that to solve a certain difficult task, you will need to call only one method.Let's talk about several JavaScript libraries designed to work with strings.1. String.js
The library string.js
, or simply S
, it is a small (less than 5 Kb minified and compressed) JavaScript library that can be used in the browser and in the Node.js. It gives the programmer a large set of methods for working with strings. These are object methods string.js
, which, for convenience, include standard string methods. An object string.js
is a kind of wrapper for regular strings.▍Installation
To install the library string.js
, just use the following command:npm i string
▍ Noteworthy methods
Between method
The method between(left, right)
extracts from the string the substring contained between the lines left
and right
. For example, you can use this method to extract elements between two HTML tags:var S = require('string');
S('<a>This is a link</a>').between('<a>', '</a>').s
Camelize method
The method camelize()
removes spaces, underscores, dashes from the processed string, and converts this string to a “camel” style. This method can be used to solve the problem mentioned at the beginning of the material.var S = require('string');
S('---Foo---bAr---').camelize().s;
Humanize method
The method humanize()
brings the processed string to a readable form. If something like this would have to be implemented from scratch, then quite a few lines of code would have to be written.var S = require('string');
S(' capitalize dash-CamelCase_underscore trim ').humanize().s
StripPunctuation method
The method stripPunctuation()
removes punctuation marks and various special characters such as asterisks and square brackets from the string. If you create such a function yourself - there is a high risk of forgetting about any character that it should process.var S = require('string');
S('My, st[ring] *full* of %punct)').stripPunctuation().s;
→ Here you can find more detailed information about this library and its methods.2. Voca
The JavaScript library Voca
offers us valuable features that enhance the usability of strings. Among its features are the following:- Change case of characters.
- Deletes the specified characters at the beginning and end of the timeline.
- Adding a string to a given length.
- Converting a string to a string, the words in which are separated by hyphens.
- Writing a string in Latin letters.
- Assembly of lines according to patterns.
- Trimming strings to the specified length
- Escaping special characters for HTML.
The modular design of the library allows, if necessary, to load not the entire library, but individual functions. This allows you to optimize bundles of applications using this library. The library code is fully tested, well-documented and well maintained.▍Installation
To install, Voca
run the following command:npm i voca
▍ Noteworthy methods
CamelCase Method
The method camelCase()
converts the string passed to it into a string written in the "camel" style.var v = require('voca');
v.camelCase('foo Bar');
v.camelCase('FooBar');
v.camelCase('---Foo---bAr---');
Latinize method
The method latinize()
returns the result of converting the string passed to it into a string written in Latin letters.var v = require('voca');
v.latinise('cafe\u0301');
v.latinise('août décembre');
v.latinise(' ');
IsAlphaDigit Method
The method isAlphaDigit()
returns true
if the string passed to it contains only alphanumeric characters.var v = require('voca');
v.isAlphaDigit('year2020');
v.isAlphaDigit('1448');
v.isAlphaDigit('40-20');
CountWords method
The method countWords()
returns the number of words in the string passed to it.var v = require('voca');
v.countWords('gravity can cross dimensions');
v.countWords('GravityCanCrossDimensions');
v.countWords('Gravity - can cross dimensions!');
EscapeRegExp Method
The method escapeRegExp()
returns a string based on the string passed to it, in which special characters are escaped.var v = require('voca');
v.escapeRegExp('(hours)[minutes]{seconds}');
→ Details about the library Voca
can be found here3. Anchorme.js
This is a compact and fast JavaScript library for finding URLs and email addresses in text and for converting them into working hyperlinks. Here are its main characteristics:- High sensitivity, low false positive rate.
- Validation of links and email addresses from the IANA Complete List.
- Validation of port numbers (if any).
- Validation of IP addresses (if available).
- Support for URLs written with characters other than Latin characters.
▍Installation
To install, anchorme.js
run the following command:npm i anchorme
▍Use
import anchorme from "anchorme";
const input = "some text with a link.com";
const resultA = anchorme(input);
The string processing can be configured using an object with parameters that can be passed to the library.→ Details anchorme.js
can be found here4. Underscore.string
A library underscore.string
is an extension underscore.js
for working with strings, which can be used separately from underscore.js
. This library was influenced by project ideas prototype.js
, right.js
and underscore.js
.This library gives the developer many useful functions designed to work with strings. Here are some of them:- capitalize ();
- clean ();
- includes ();
- count ();
- escapeHTML ();
- unescapeHTML ();
- insert ();
- splice ();
- startsWith ();
- endsWith ();
- titleize ();
- trim ();
- truncate ().
▍Installation
Here is the command you can install the library with:npm i underscore.string
▍ Noteworthy methods
NumberFormat method
The method is numberFormat()
designed to format numbers.var _ = require("underscore.string");
_.numberFormat(1000, 3)
_.numberFormat(123456789.123, 5, '.', ',');
Levenshtein method
The method levenshtein()
returns the Levenshtein distance between two lines. Read more about the algorithm used in this method here .var _ = require("underscore.string");
_.levenshtein('kitten', 'kittah');
Chop method
The method chop()
splits the string passed to it into parts.var _ = require("underscore.string");
_.chop('whitespace', 3);
→ Here is a page where you can find details about the libraryunderscore.string
5. Stringz
The main feature of the library stringz
is that it can work with Unicode characters.If, without using any libraries, the following code is executed, it turns out that the string length is 2. The
thing is that the JS-string method length()
returns the number of code points of the string, and not the number of characters. In fact, some characters are in ranges 010000–03FFFF
and 040000–10FFFF
can use up to 4 bytes (32 bits) per code point, but this does not change the answer: in order to represent some characters, you need more than 2 bytes, as a result they are represented by more than one code point.→ Here is the material about the problems of processing Unicode characters in JavaScript.▍Installation
To install this library you need the following command:npm i stringz
▍ Noteworthy methods
Limit method
The method limit()
casts the string to the specified length.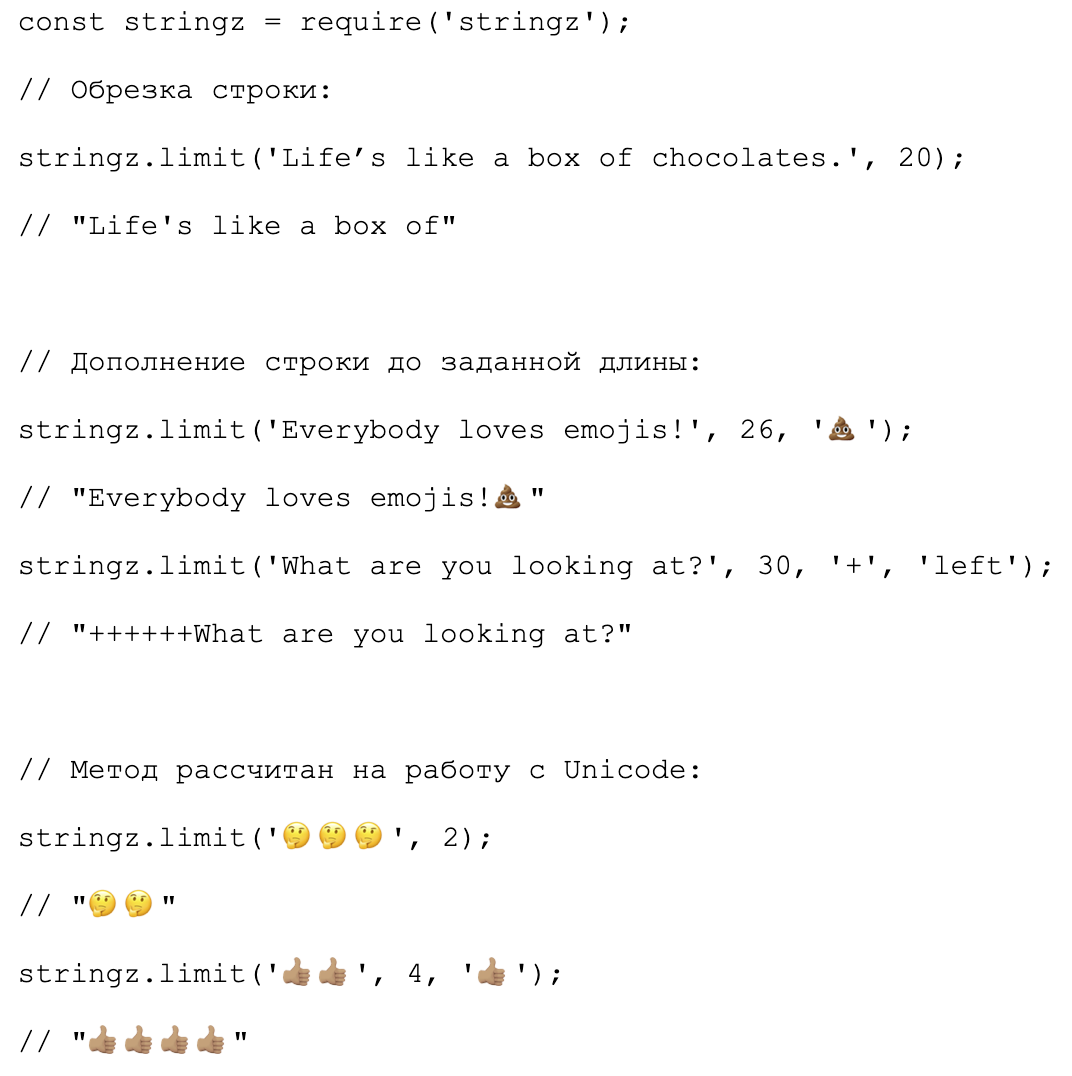
ToArray method
The method toArray()
converts a string to an array.
→ Here is the library repository page.And what auxiliary tools do you use to work with strings?We remind you that we are continuing the prediction contest in which you can win a brand new iPhone. There is still time to break into it, and make the most accurate forecast on topical values.
