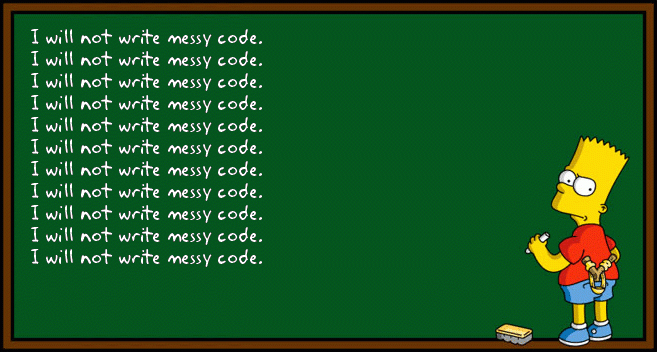
How can knowledge of neuropsychology help a programmer to style code? Before I started programming, I studied neuropsychology for a very long time. Subsequently, this knowledge helped me achieve high results in development in a short period of time.
In this article I want to share my experience and approach to code styling. We will consider the stylization on the programming side, and then touch on neuropsychology for a deeper understanding of the process and its degree of importance for the developer. Last year, I have been working at Secreate, where I’m developing mobile applications using the React Native framework, so the code examples in this article will be in Javascript. The knowledge gained from this article can be easily applied to any programming language. At the end of the article you will find useful examples of code styling. Enjoy reading!
What is stylization?
code style. — , : , , , . , -. .
:
import {SomeClass} from ‘some/file’
:
import { SomeClass } from ‘some/file’
:
import {SomeClass, someFunction, someConst, AnotherClass } from ‘some/file’
:
import {
SomeClass,
somefunction,
someConst,
AnotherClass,
} from ‘some/file’
:
function foo() {
some code...
}
:
function foo ()
{
some code…
}
:
condition ?
some very long long long long long expression :
another expression
:
condition
? some very long long long long long expression
: another expression
. , , , .
. , . . , . , , , . . , . . . , . — !
? , . . , , . ? , , .
, . . . , , . . , ( ).
. , . . , . . : , , . . , . , .
. , , . , . , ( ). ( ). , , . , , .
. , — , , . - . — . , , . - , , . , . .
, . , , . , , , . , “ ”.
, . , , . - , code style.
, . , , . , , . . , . , . .
, . , .
. , . ( ) .
2 . , .
:
<View>
<Text>Some text</Text>
<TouchableOpacity>
<Text>Title</Text>
<TouchableOpacity/>
</View>
Animated.timing(
animatedValue,
{
toValue: someValue,
duration: someDuration,
anotherOptions,
},
)
, .
const object = { field: value, anotherField: value }
, , .
const object = {
field: value,
anotherField: value,
moreFields,
}
. .
:
function someFunction() {
some code
}
Separate keywords with a blank line.
Example:
function sum(a, b) {
const result = a + b
return result
}
Or:
if (condition) {
do this
} else {
do that
}
switch (value) {
case someCase:
do this
break
case anotherCase:
do that
break
}
Separate function declarations and actions of different types with a blank line
Example:
function someFunction() {
do something
}
function anotherFunction() {
do another thing
}
Or:
function someFunction() {
const someConst
const anotherConst
let someLet
let anotherLet
if (condition) {
do something
}
this.function()
this.anotherFunction()
}
Sequence of elements
Follow a strict sequence of elements.
An example of an extension class React.Component:
- Static.
- Class Fields.
- Constructor
- State.
- Life cycle functions.
- Secondary functions.
- Action handlers.
- Helper render functions.
- Render.
Separate the self-closing tag with a space.
Example:
<View style={styles.underline} />
Transfer props to the next line if there are more than one
Example:
<TouchableOpacity
style={styles.button}
onPress={this.handlePress}
>
<Text>Title</Text>
</TouchableOpacity>
Long conditions
Break long conditions into several lines, starting each line with a conditional character.
Example:
if (
condition
&& anotherCondition
&& (
value < anotherValue
|| someCondition
)
) {
do something
}
Or:
<View>
{
this.state.isButtonVisible
? (
<TouchableOpacity>
<Text>Title</Text>
</TouchableOpacity>
)
: null
}
</View>
Separate conditional characters with spaces
Example:
const sum = a + b
Or:
return a < b ? a : b
Or:
const object = {
field: value,
...value > 0 ? { value } : {},
}