I began to keep a list of the most frequently used functions, solving algorithmic problems on LeetCode and HackerRank.Being a good programmer does not mean remembering all the built-in functions of a certain language. But this does not mean that their memorization is useless. Especially - when it comes to preparing for an interview.Today I want to share with everyone who wants my cheat sheet for working with strings in Python. I designed it as a list of questions, which I use for self-testing. Although these questions are not drawn to the full-fledged tasks that are offered at the interviews, their development will help you in solving real programming problems.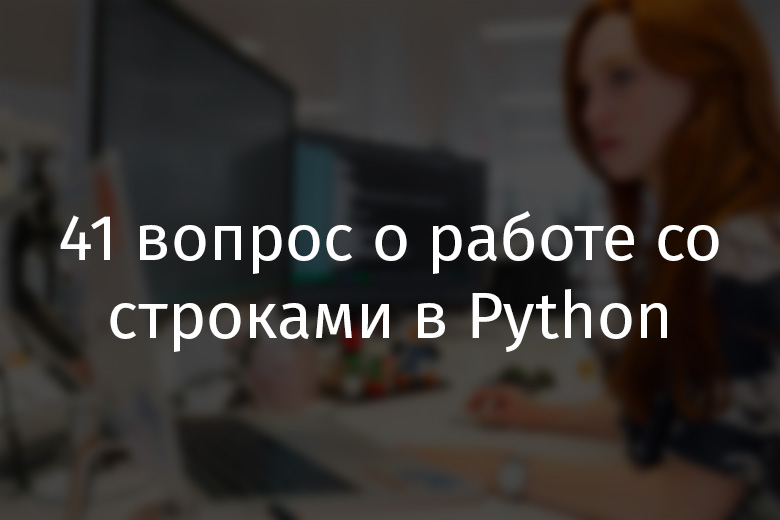
1. How to check two objects for identity?
The operator is
returns True
if a reference to the same memory area is written in two variables. This is what we are talking about when talking about the "identity of objects."Do not confuse is
and ==
. The operator ==
checks only the equality of objects.animals = ['python','gopher']
more_animals = animals
print(animals == more_animals)
print(animals is more_animals)
even_more_animals = ['python','gopher']
print(animals == even_more_animals)
print(animals is even_more_animals)
Pay attention to the fact that animals
they are even_more_animals
not identical, although they are equal to each other.In addition, there is a function id()
that returns the identifier of the memory address associated with the variable name. When this function is called, the same identifier will be returned for two identical objects.name = 'object'
id(name)
2. How to check that every word in a line begins with a capital letter?
There is a string method istitle()
that checks whether every word in a string starts with a capital letter.print( 'The Hilton'.istitle() )
print( 'The dog'.istitle() )
print( 'sticky rice'.istitle() )
3. How to check a string for another string in it?
There is a statement in
that will return True
if the string contains the search string.print( 'plane' in 'The worlds fastest plane' )
print( 'car' in 'The worlds fastest plane' )
4. How to find the index of the first occurrence of a substring in a string?
There are two methods that return the index of the first occurrence of a substring in a string. This is find()
and index()
. Each of them has certain features.The method find()
returns -1
if the required substring in the string is not found.'The worlds fastest plane'.find('plane')
'The worlds fastest plane'.find('car')
A method index()
in such a situation throws an error ValueError
.'The worlds fastest plane'.index('plane')
'The worlds fastest plane'.index('car')
5. How to count the number of characters in a string?
The function len()
returns the length of the string.len('The first president of the organization..')
6. How to count how many times a certain character occurs in a string?
The method count()
that returns the number of occurrences in the string of the specified character will help us answer this question .'The first president of the organization..'.count('o')
7. How to make the first character of the string in capital letter?
In order to do this, you can use the method capitalize()
.'florida dolphins'.capitalize()
8. What are f-lines and how to use them?
Python 3.6 introduces a new feature, the so-called "f-strings." Their use greatly simplifies string interpolation. Using f-lines is reminiscent of a method format()
.When f-lines are declared, a letter is written before the opening quotation mark f
.name = 'Chris'
food = 'creme brulee'
f'Hello. My name is {name} and I like {food}.'
9. How to find a substring in a given part of a string?
The method index()
can be called by passing optional arguments to it, representing the index of the initial and final fragment of the string, within which you need to search for a substring.'the happiest person in the whole wide world.'.index('the',10,44)
Pay attention to the fact that the above construction returns 23
, and not 0
, as it were, would not limit our search.'the happiest person in the whole wide world.'.index('the')
10. How to insert the contents of a variable into a string using the format () method?
The method format()
allows you to achieve results similar to those that can be obtained using f-lines. True, I believe that it is format()
not so convenient to use, since all variables have to be specified as arguments format()
.difficulty = 'easy'
thing = 'exam'
'That {} was {}!'.format(thing, difficulty)
11. How do I know if a string contains only numbers?
There is a method isnumeric()
that returns True
if all characters in the string are numbers.'80000'.isnumeric()
Using this method, keep in mind that he does not count punctuation marks as numbers.'1.0'.isnumeric()
12. How to split a string by a given character?
Here we will be helped by a method split()
that breaks a string by a given character or by several characters.'This is great'.split(' ')
'not--so--great'.split('--')
13. How to check a string that it is composed only of lowercase letters?
The method islower()
returns True
only if the string is composed solely of lowercase letters.'all lower case'.islower()
'not aLL lowercase'.islower()
14. How to check that a string begins with a lowercase letter?
This can be done by calling the above method islower()
for the first character of the string.'aPPLE'[0].islower()
15. Is it possible in Python to add an integer to a string?
This is possible in some languages, but Python will throw an error when trying to perform a similar operation TypeError
.'Ten' + 10
16. How to βflipβ a line?
In order to βflipβ a line, it can be split by presenting it as a list of characters, βflipβ the list, and, combining its elements, form a new line.''.join(reversed("hello world"))
17. How to combine the list of lines into one line, the elements of which are separated by hyphens?
The method join()
can combine list items into strings, separating individual strings using the specified character.'-'.join(['a','b','c'])
18. How do I know that all characters in a string are in ASCII?
The method isascii()
returns True
if all characters in the string are in ASCII.print( 'Γ'.isascii() )
print( 'A'.isascii() )
19. How to cast the entire string to upper or lower case?
To solve these problems, you can use the methods upper()
and lower()
, which, respectively, lead all the characters of the strings to upper and lower case.sentence = 'The Cat in the Hat'
sentence.upper()
sentence.lower()
20. How to convert the first and last characters of a string to uppercase?
Here, as in one of the previous examples, we will refer to the characters of the string by index. Lines in Python are immutable, so we will build new lines based on existing ones.animal = 'fish'
animal[0].upper() + animal[1:-1] + animal[-1].upper()
21. How to check a string that it is composed only of capital letters?
There is a method isupper()
that is similar to the one already considered islower()
. But isupper()
returns True
only if the entire string consists of uppercase letters.'Toronto'.isupper()
'TORONTO'.isupper()
22. In what situation would you use the splitlines () method?
The method splitlines()
separates strings by line break characters.sentence = "It was a stormy night\nThe house creeked\nThe wind blew."
sentence.splitlines()
23. How to get a line slice?
To obtain a slice of a string, the following syntax is used:string[start_index:end_index:step]
Here step
is the step with which string characters from a range will be returned start_index:end_index
. A step
value of 3 indicates that every third character will be returned.string = 'I like to eat apples'
string[:6]
string[7:13]
string[0:-1:2]
24. How to convert an integer to a string?
You can use the constructor to convert a number to a string str()
.str(5)
25. How do I know that a string contains only alphabetic characters?
The method isalpha()
returns True
if all characters in the string are letters.'One1'.isalpha()
'One'.isalpha()
26. How to replace all occurrences of a certain substring in a given line?
If you do without exporting a module that allows you to work with regular expressions, you can use the method to solve this problem replace()
.sentence = 'Sally sells sea shells by the sea shore'
sentence.replace('sea', 'mountain')
27. How to return a character of a line with the minimum ASCII code?
If you look at the ASCII codes of the elements, it turns out, for example, that uppercase letters have lower codes than lowercase. The function min()
returns the character of the string having the smallest code.min('strings')
28. How to check a string that it contains only alphanumeric characters?
Alphanumeric characters include letters and numbers. To answer this question, you can use the method isalnum()
.'Ten10'.isalnum()
'Ten10.'.isalnum()
29. How to remove spaces from the beginning of a line (from its left side), from its end (from the right side), or on both sides of a line?
Here, methods lstrip()
, rstrip()
and , respectively, are useful to us strip()
.string = ' string of whitespace '
string.lstrip()
string.rstrip()
string.strip()
30. How to check that a string starts with a given sequence of characters, or ends with a given sequence of characters?
To answer this question, you can resort, respectively, to the methods startswith()
and endswith()
.city = 'New York'
city.startswith('New')
city.endswith('N')
31. How to encode a string in ASCII?
The method encode()
allows you to encode strings using the specified encoding. The default encoding is used utf-8
. If a certain character cannot be represented using the specified encoding, an error will be generated UnicodeEncodeError
.'Fresh Tuna'.encode('ascii')
'Fresh Tuna Γ'.encode('ascii')
32. How do I know that a string includes only spaces?
There is a method isspace()
that returns True
only if the string consists solely of spaces.''.isspace()
' '.isspace()
' '.isspace()
' the '.isspace()
33. What happens if you multiply a row by 3?
A new line will be created, representing the original line repeated three times.'dog' * 3
34. How to make the first character of each word in a string uppercase?
There is a method title()
that uppercase the first letter of each word in a string.'once upon a time'.title()
35. How to combine two lines?
You can use the operator to concatenate strings +
.'string one' + ' ' + 'string two'
36. How to use partition () method?
The method partition()
breaks the string by the given substring. After that, the result is returned as a tuple. Moreover, the substring along which the breakdown was carried out is also included in the tuple.sentence = "If you want to be a ninja"
print(sentence.partition(' want '))
37. Lines in Python are immutable. What does it mean?
The fact that strings are immutable suggests that after a string object is created, it cannot be changed. When βmodifyingβ strings, the original strings do not change. Instead, completely new objects are created in memory. You can prove this using the function id()
.proverb = 'Rise each day before the sun'
print( id(proverb) )
proverb_two = 'Rise each day before the sun' + ' if its a weekday'
print( id(proverb_two) )
When concatenating 'Rise each day before the sun'
and ' if its a weekday'
in memory, a new object is created with a new identifier. If the original object were to change, then the objects would have the same identifier.38. If you declare the same line twice (writing it into 2 different variables) - how many objects will be created in memory? 1 or 2?
An example of such work with strings is the following code fragment:animal = 'dog'
pet = 'dog'
With this approach, only one object is created in memory. When I came across this for the first time, it did not seem intuitive to me. But this mechanism helps Python save memory when working with long strings.This can be proved by resorting to a function id()
.animal = 'dog'
print( id(animal) )
pet = 'dog'
print( id(pet) )
39. How to use the maketrans () and translate () methods?
The method maketrans()
allows you to describe the mapping of one character to another, returning a conversion table.The method translate()
allows you to apply the specified table to convert the string.
mapping = str.maketrans("abcs", "123S")
"abc are the first three letters".translate(mapping)
Please note that the bar is made of symbols replacement a
, b
, c
and s
, respectively, the symbols 1
, 2
, 3
and S
.40. How to remove vowels from a string?
One answer to this question is that characters in a string are sorted using the List Comprehension mechanism. Symbols are checked by comparing with a tuple containing vowels. If the character is not included in the tuple, it is appended to a new line.string = 'Hello 1 World 2'
vowels = ('a','e','i','o','u')
''.join([c for c in string if c not in vowels])
41. In what situations do you use the rfind () method?
The method rfind()
is similar to the method find()
, but, unlike find()
, it scans the string not from left to right, but from right to left, returning the index of the first found occurrence of the desired substring.story = 'The price is right said Bob. The price is right.'
story.rfind('is')
Summary
I often explain to one product manager, a person aged, that developers are not dictionaries that store descriptions of object methods. But the more methods the developer remembers, the less he will have to google, and the faster and more pleasant he will work. I hope that now you can easily answer the questions discussed here.Dear readers! What about string handling in Python, would you advise those who are preparing for an interview?