When working with PowerShell, the first thing we come across is the command (Cmdlet).
The command call looks like this:
Verb-Noun -Parameter1 ValueType1 -Parameter2 ValueType2[]
Help
Help is called up in PowerShell using the Get-Help command. You can specify one of the parameters: example, detailed, full, online, showWindow.
Get-Help Get-Service -full will return a complete description of the Get-Service command Get-
Help Get-S * will show all available commands and functions starting with Get-S *
There is also detailed documentation on the official Microsoft website.
Here is an example of help for the Get-Evenlog command
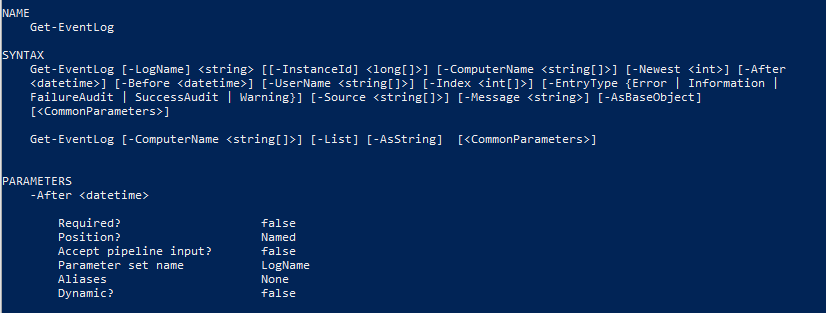
[], .
, . , .
EntryType, . .
Required. After , Required false. Position Named. , :
Get-EventLog -LogName Application -After 2020.04.26
LogName Named 0 , :
Get-EventLog Application -After 2020.04.26
:
Get-EventLog -Newest 5 Application
Alias
PowerShell (Alias).
Set-Location cd.
Set-Location “D:\”
cd “D:\”
History
Get-History
Invoke-History 1; Invoke-History 2
Clear-History
Pipeline
powershell . :
Get-Verb | Measure-Object
.
Get-Verb "get"
Get-Help Get-Verb -Full, Verb pipline input ByValue.

Get-Verb «get» «get» | Get-Verb.
Verb Get-Verb pipline input .
pipline input ByPropertyName. Verb.
Variables
$
$example = 4
>
, $example > File.txt
$example
Set-Content -Value $example -Path File.txt
Arrays
Array initialization:
$ArrayExample = @(“First”, “Second”)
Initializing an empty array:
$ArrayExample = @()
Getting the value by index:
$ArrayExample[0]
Get the whole array:
$ArrayExample
Adding an item:
$ArrayExample += “Third”
$ArrayExample += @(“Fourth”, “Fifth”)
Sorting:
$ArrayExample | Sort
$ArrayExample | Sort -Descending
But the array itself with this sorting remains unchanged. And if we want sorted data in the array, then we need to assign the sorted values:
$ArrayExample = $ArrayExample | Sort
In fact, there is no element removed from the array in PowerShell, but you can do it this way:
$ArrayExample = $ArrayExample | where { $_ -ne “First” }
$ArrayExample = $ArrayExample | where { $_ -ne $ArrayExample[0] }
Delete array:
$ArrayExample = $null
Loops
Loop syntax:
for($i = 0; $i -lt 5; $i++){}
$i = 0
while($i -lt 5){}
$i = 0
do{} while($i -lt 5)
$i = 0
do{} until($i -lt 5)
ForEach($item in $items){}
Exiting the break loop.
Skip continue.
Conditional statements
if () {} elseif () {} else
switch($someIntValue){
1 { “Option 1” }
2 { “Option 2” }
default { “Not set” }
}
Function
Function Definition:
function Example () {
echo &args
}
Function Launch:
Example “First argument” “Second argument”
Defining arguments in a function:
function Example () {
param($first, $second)
}
function Example ($first, $second) {}
Function Launch:
Example -first “First argument” -second “Second argument”
Exception
try{
} catch [System.Net.WebException],[System.IO.IOException]{
} catch {
} finally{
}