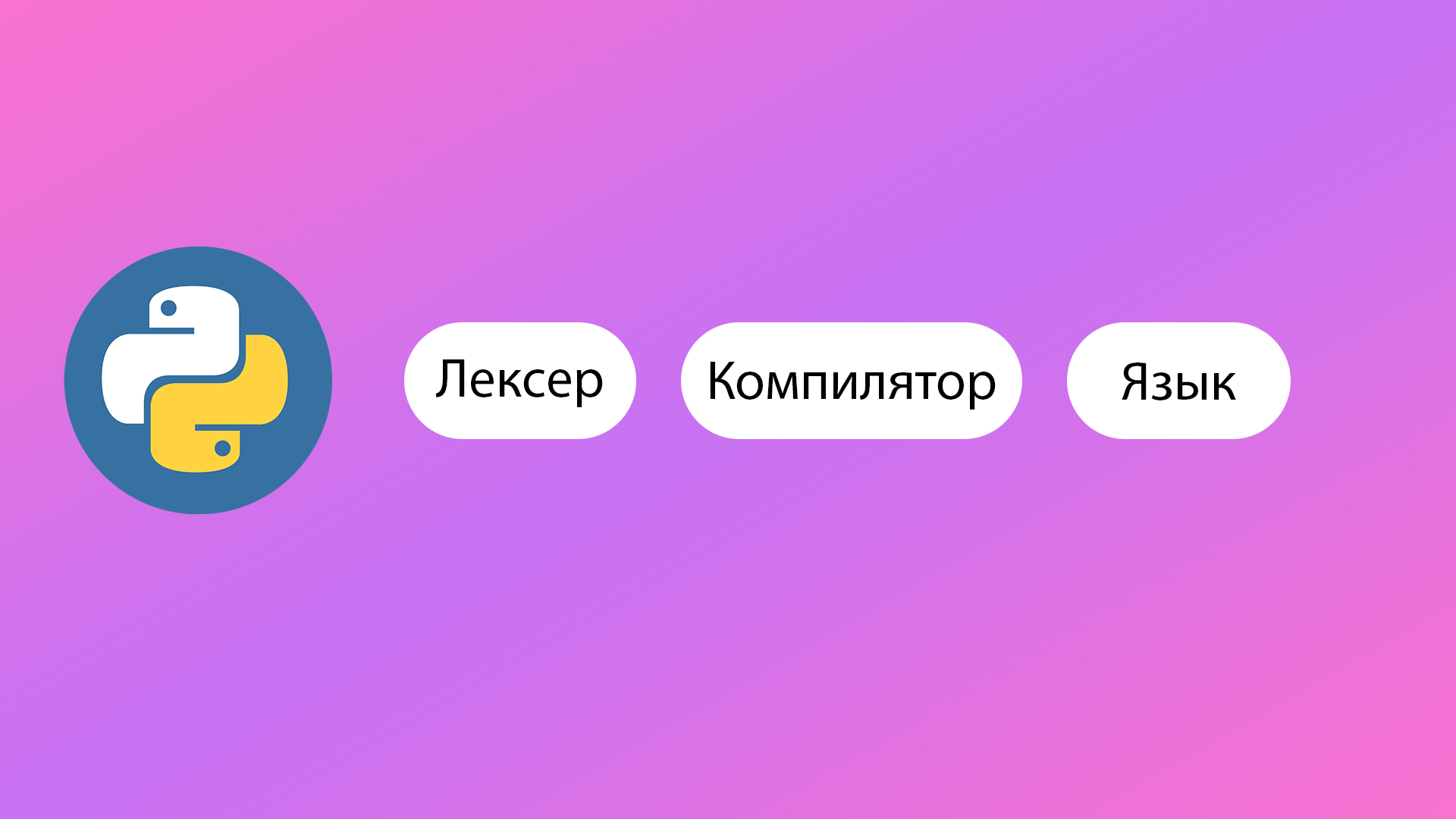
We write our "programming language" in Python3
1. Lexer
In this article we will do, so to speak, python2, create variables, output them to the console
If it comes to you, then I will do part 2 of the post: D
To begin with,let's determine the name of the language - for example, “xnn”. Now create the xnn.py file and write the main code there:import sys
import os
dir = os.path.abspath(os.curdir)
p = str(dir) + '\\' + str(sys.argv[1])
print(p)
modules = ['if', 'else', 'while', 'for', '=', '==', '{', '}', '[', ']', '(', ')', '//']
var = []
vars_ = {}
with open(p, 'r', encoding="UTF-8") as f:
for ex in f.read().split():
var.append(ex)
print(var)
What are we doing:- Import the os and sys libraries
- Using os, we determine where the compiler is launched from (in the future I will tell you why)
- Create the path to the file + take the file itself from the argument - we get it through sys.argv
- Next, we display the path itself, with the file (in the future it will need to be deleted)
- We create all the reserved words - for now, we don’t need it, but in the future we’ve got it for error output
- Create lists for lexer and variables
- We create the lexer itself, for this we open the file, in read mode, be sure to
encoding = "UTF-8"
if this is not done, then instead of Russian letters, there will be hieroglyphs! - Using for ex in read (). Split () we distribute all the text into words and put each word separately in ex
- Next, write each word in the var list
- And we will display the list itself - in the future it will be necessary to remove the output: /
Creating a .xnn File
Now we will create the file itself which we will parse, and also change the way of opening.
Open it and write it there:pr =
rprint pr
2. Creating a Compiler
Now create a compiler!To do this, we supplement our file with code so that it turns out like this:import sys
import os
dir = os.path.abspath(os.curdir)
p = str(dir) + '\\' + str(sys.argv[1])
print(p)
modules = ['if', 'else', 'while', 'for', '=', '==', '{', '}', '[', ']', '(', ')', '//']
var = []
vars_ = {}
try:
with open(p, 'r', encoding="UTF-8") as f:
for ex in f.read().split():
var.append(ex)
print(var)
a = -1
for i in var:
a = a + 1
if i == '=':
vars_[var[a-1]] = var[a+1]
if i == 'rprint':
let = var[a+1]
for key, value in vars_.items():
if key == let:
print(value)
except FileNotFoundError:
print('Error! !')
What are we doing?We iterate over all the values in var and when we find one or another reserved word, we do certain actionsVariable assignment
If i coincides with "=", add a value to the vars_ list , before "=" as key, and after "=" as value - thus forming a variableVariable output
If i matches “rprint”, we look in the list vars_ for a match for this variable, which we get through our friend, the var list.After finding, display the value of the variableWork in action
In order to compile the file, enter the command data in cmd:cd path/to/files
python xnn.py prog.xnn
The output is:
C:\Users\Hoopengo\Desktop\xnn\prog.xnn
['pr', '=', '', 'rprint', 'pr']
3. Compilation in .exe
Create a copy of the file folder and edit the xnn.py fileimport sys
import os
dir = os.path.abspath(os.curdir)
p = str(dir) + '\\' + str(sys.argv[1])
modules = ['if', 'else', 'while', 'for', '=', '==', '{', '}', '[', ']', '(', ')', '//']
var = []
vars_ = {}
try:
with open(p, 'r', encoding="UTF-8") as f:
for ex in f.read().split():
var.append(ex)
a = -1
for i in var:
a = a + 1
if i == '=':
vars_[var[a-1]] = var[a+1]
if i == 'rprint':
let = var[a+1]
for key, value in vars_.items():
if key == let:
print(value)
except FileNotFoundError:
print('Error! !')
Install auto-py-to-exeComplete installation information is available at this link.Let's enter the console:auto-py-to-exepip install auto-py-to-exe
auto-py-to-exe
The browser starts up. Choose the path to the file and One File there.
4. Creating an installer
In the copied folder, create the bat.py file:
import os
with open('path\\xnn.exe', 'r', encoding='UTF-8') as f:
lean = f.read()
directory_folder = r"C:\\Windows\\System32\\xnn.exe"
folder_path = os.path.dirname(directory_folder)
if not os.path.exists(folder_path):
os.makedirs(folder_path)
with open(directory_folder, 'w', encoding='UTF-8') as file:
file.write(lean)
After that, compile it into .exe and drop it into the same directory, it should look like this:
5. Installation
Now install.To do this, open the file bat.exe, after which the installation will be done.Restart the console and enter:cd path/to/file
xnn prog.xnn
And we get:
If it says “xnn is not an internal command”, then add the xnn.exe file to the “C: \ Windows \ System32 \” folder yourself.