Article material taken from my zen channel .
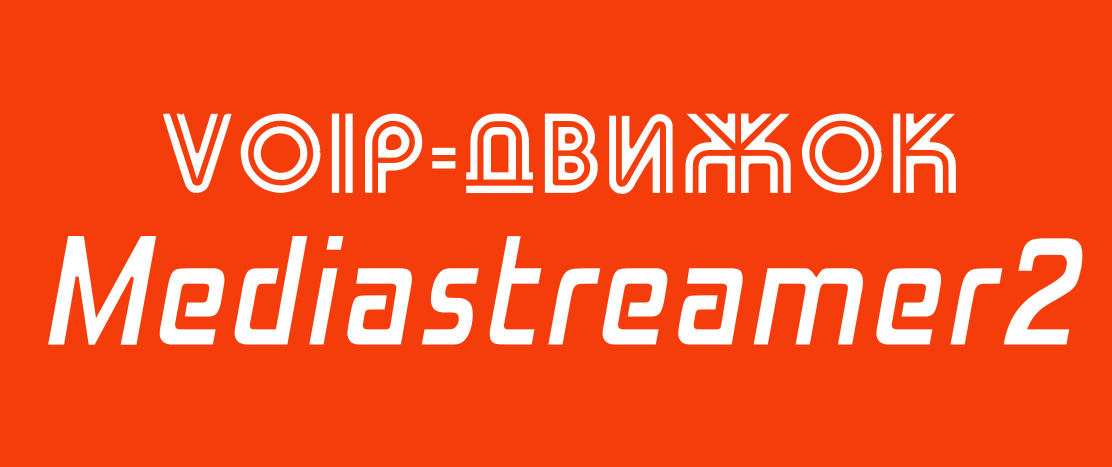
Tone Detector
In the last article, we created a signal level meter. In this we will learn how to detect a tone.

In the old days, when not every family had a TV, and half of them switched the channels with the help of pliers, in the reviews of the foreign technical press there was intriguing news that one of the TV manufacturers equipped their devices with a wireless remote control. From the details it was known that the remote control works without batteries due to the use of an unusual approach - the remote was mechanical and was a hybrid of a musical instrument - a metallophone and a revolver. The revolver’s drum contained metal cylinders of different lengths, and when the striker hit one of them, the cylinder began to ring at its own frequency. Presumably in ultrasound. The electronics in the TV heard this signal and, having determined its frequency, performed the corresponding action - switch the channel, change the volume,turn off the TV.
, .
, . , . , 6 , / , , / . :
struct _MSToneDetectorDef{
char tone_name[8];
int frequency;
int min_duration;
float min_amplitude;
};
typedef struct _MSToneDetectorDef MSToneDetectorDef;
10 , . . MS_TONE_DETECTOR_ADD_SCAN.
, , , . ms_filter_set_notify_callback(). , , , ( ).
, , , :
struct _MSToneDetectorEvent{
char tone_name[8];
uint64_t tone_start_time;
};
typedef struct _MSToneDetectorEvent MSToneDetectorEvent;
.
.
#include <mediastreamer2/msfilter.h>
#include <mediastreamer2/msticker.h>
#include <mediastreamer2/dtmfgen.h>
#include <mediastreamer2/mssndcard.h>
#include <mediastreamer2/msvolume.h>
#include <mediastreamer2/mstonedetector.h>
#include <mediastreamer2/mseventqueue.h>
static void tone_detected_cb(void *data, MSFilter *f, unsigned int event_id,
MSToneDetectorEvent *ev)
{
printf(" : %s\n", ev->tone_name);
}
int main()
{
ms_init();
MSFilter *voidsource = ms_filter_new(MS_VOID_SOURCE_ID);
MSFilter *dtmfgen = ms_filter_new(MS_DTMF_GEN_ID);
MSFilter *volume = ms_filter_new(MS_VOLUME_ID);
MSSndCard *card_playback =
ms_snd_card_manager_get_default_card(ms_snd_card_manager_get());
MSFilter *snd_card_write = ms_snd_card_create_writer(card_playback);
MSFilter *detector = ms_filter_new(MS_TONE_DETECTOR_ID);
ms_filter_call_method(detector, MS_TONE_DETECTOR_CLEAR_SCANS, 0);
MSTicker *ticker=ms_ticker_new();
ms_filter_link(voidsource, 0, dtmfgen, 0);
ms_filter_link(dtmfgen, 0, volume, 0);
ms_filter_link(volume, 0, detector, 0);
ms_filter_link(detector, 0, snd_card_write, 0);
ms_filter_set_notify_callback(detector,
(MSFilterNotifyFunc)tone_detected_cb, NULL);
ms_ticker_attach(ticker,voidsource);
MSToneDetectorDef scan[6]=
{
{"V+", 440, 100, 0.1},
{"V-", 540, 100, 0.1},
{"C+", 640, 100, 0.1},
{"C-", 740, 100, 0.1},
{"ON", 840, 100, 0.1},
{"OFF", 940, 100, 0.1}
};
int i;
for (i = 0; i < 6; i++)
{
ms_filter_call_method(detector, MS_TONE_DETECTOR_ADD_SCAN,
&scan[i]);
}
MSDtmfGenCustomTone dtmf_cfg;
dtmf_cfg.tone_name[0] = 0;
dtmf_cfg.duration = 1000;
dtmf_cfg.frequencies[0] = 440;
dtmf_cfg.frequencies[1] = 0;
dtmf_cfg.amplitude = 1.0;
dtmf_cfg.interval = 0.;
dtmf_cfg.repeat_count = 0.;
char key='9';
printf(" , .\n"
" 0.\n");
while(key != '0')
{
key = getchar();
if ((key >= 49) && (key <= 54))
{
printf(" : %c\n", key);
dtmf_cfg.frequencies[0] = 440 + 100*(key-49);
ms_filter_call_method(dtmfgen, MS_DTMF_GEN_PLAY_CUSTOM,
(void*)&dtmf_cfg);
}
ms_usleep(20000);
}
}
We compile and run the program. If everything works correctly, then after starting we should get something like this:
$ ./mstest4
ALSA lib conf.c:4738:(snd_config_expand) Unknown parameters 0
ALSA lib control.c:954:(snd_ctl_open_noupdate) Invalid CTL default:0
ortp-warning-Could not attach mixer to card: Invalid argument
ALSA lib conf.c:4738:(snd_config_expand) Unknown parameters 0
ALSA lib pcm.c:2266:(snd_pcm_open_noupdate) Unknown PCM default:0
ALSA lib conf.c:4738:(snd_config_expand) Unknown parameters 0
ALSA lib pcm.c:2266:(snd_pcm_open_noupdate) Unknown PCM default:0
ortp-warning-Strange, sound card Intel 82801AA-ICH does not seems to be capable of anything, retrying with plughw...
, .
0.
ortp-warning-alsa_set_params: periodsize:256 Using 256
ortp-warning-alsa_set_params: period:8 Using 8
Press any key from "1" to "6", confirming with the "Enter" key, you should get something like this listing:
2
: 2
: V-
1
: 1
: V+
3
: 3
: C+
4
: 4
: C-
0
$
We see that command tones are sent successfully and the detector detects them.
In the next article, we will turn to transmitting a sound signal over an Ethernet network using the RTP protocol and immediately apply it in our remote control.