The complexity of the Web is changing daily, and its capabilities are growing just as fast, especially with 3D rendering. Who is just starting to join the 3D theme - welcome to cat.
Let's go from afar
WebGL is a software library for JavaScript that allows you to create 3D graphics that work in browsers. This library is based on the architecture of the OpenGL library. WebGL uses the GLSL shader programming language , which has C-like syntax. WebGL is interesting in that the code is modeled directly in the browser. For this, WebGL uses the canvas object that was introduced in HTML5.Working with WebGL, and with shaders in particular, is a rather time-consuming process. In the development process, it is necessary to describe each point, line, face, and so on. In order to visualize all this, we need to register a rather voluminous piece of code. To increase development speed, Three.js library was developed .Three.js is a JavaScript library containing a set of predefined classes for creating and displaying interactive 3D graphics in WebGL.Three.js for WebGL is the same as jQuery for JavaScript. The library offers declarative syntax, and abstracts from the headaches associated with 3D in the browser. Let's take a general overview and see how to get started if you are new to the 3D world.More on Three.js
The Three.js library, as already mentioned, makes working with WebGL easier. When using Three.js, there is no need to write shaders (but the possibility remains), and it becomes possible to operate on familiar concepts.A large number of developers work on the library. The main ideologist and developer is Ricardo Cobello, known under the creative pseudonym Mr.Doob .Modeling graphics using Three.js can be compared with the set, as we have the opportunity to operate with concepts such as scene, light, camera, objects and their materials.Three so-called Three.js whales include:- Scene - a kind of platform where all the objects that we create are placed;
- Camera - in fact - this is the “eye” that will be directed to the scene. The camera takes and displays objects that are located on the stage;
- Renderer - a visualizer that allows you to display a scene captured by the camera.
Three.js has several types of cameras:- Perspective camera
- Stereo camera
- Orthographic camera
- Cube camera
The most common ones are the Perspective Camera and Orthographic Camera.Perspective camera
This is the most common projection mode used to render a 3D scene.The perspective camera is designed to simulate what the human eye sees. The camera perceives all objects in a perspective projection, that is: the farther the object is from us, the smaller it seems.
The perspective camera takes 4 arguments:- FOV Field Of View (/ ) — , .
- Aspect ratio — , , . . , , .
- Near & Far — , . , , , .

Orthographic Camera
In this projection mode, the size of the object in the displayed image remains constant, regardless of its distance from the camera. That is, it is a camera remote at an infinite distance from objects.In this case, all perpendicular lines remain perpendicular, all parallel - parallel. If we move the camera, the lines and objects will not be distorted.This can be useful when displaying 2D scenes and UI elements.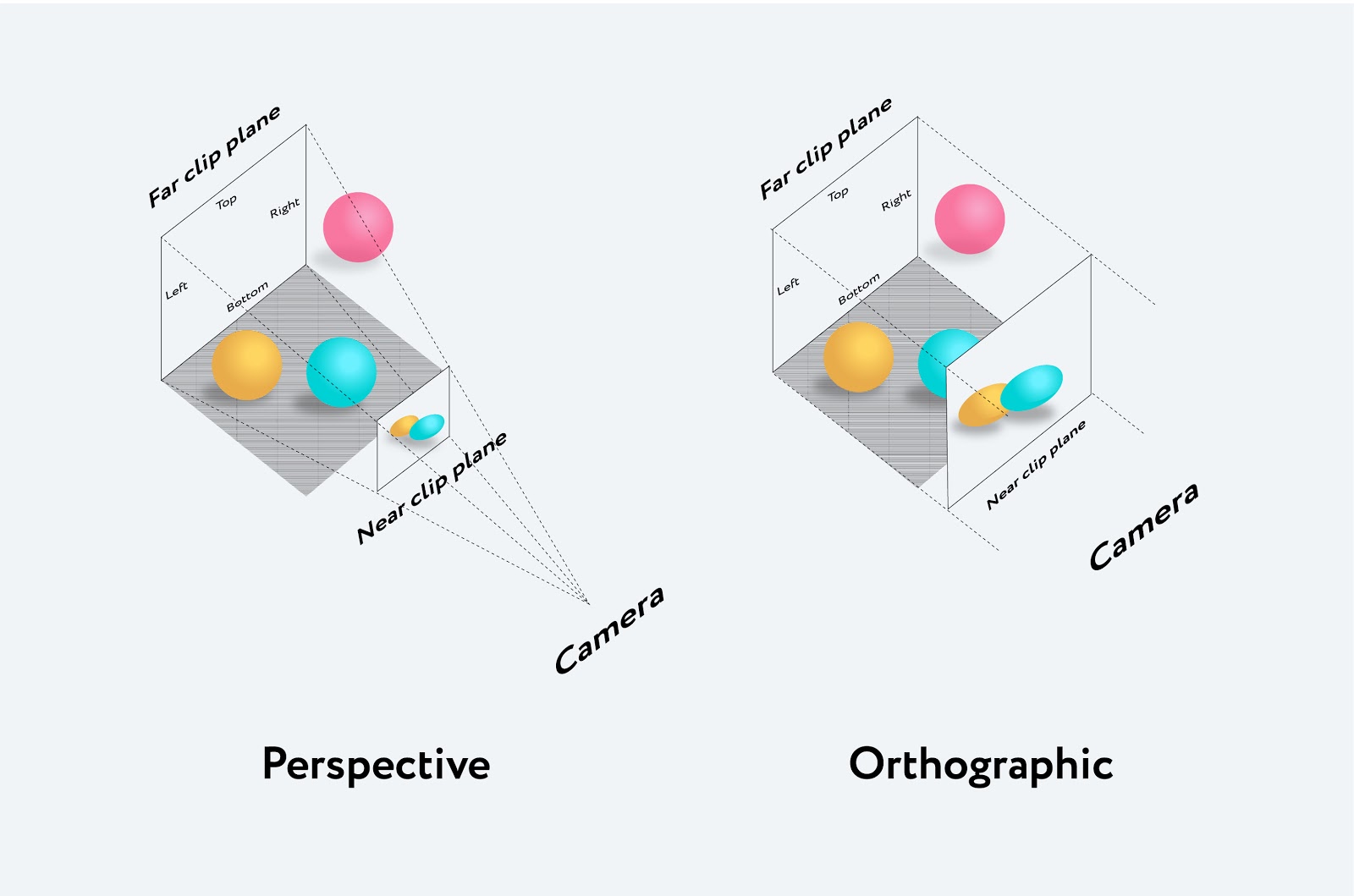
Lighting
Without lighting on the stage, you will get the impression that you are in a dark room. In addition, by lighting the stage, you can give greater realism. Technically, each lighting can be set to color.Lighting Examples:- Ambient Light — , ; , .
- Directional Light — , . , , , , ; , .
- Point Light — , . ( ).
- Spot Light - this light is emitted from one point in one direction, along a cone, expanding as you move away from the light source.
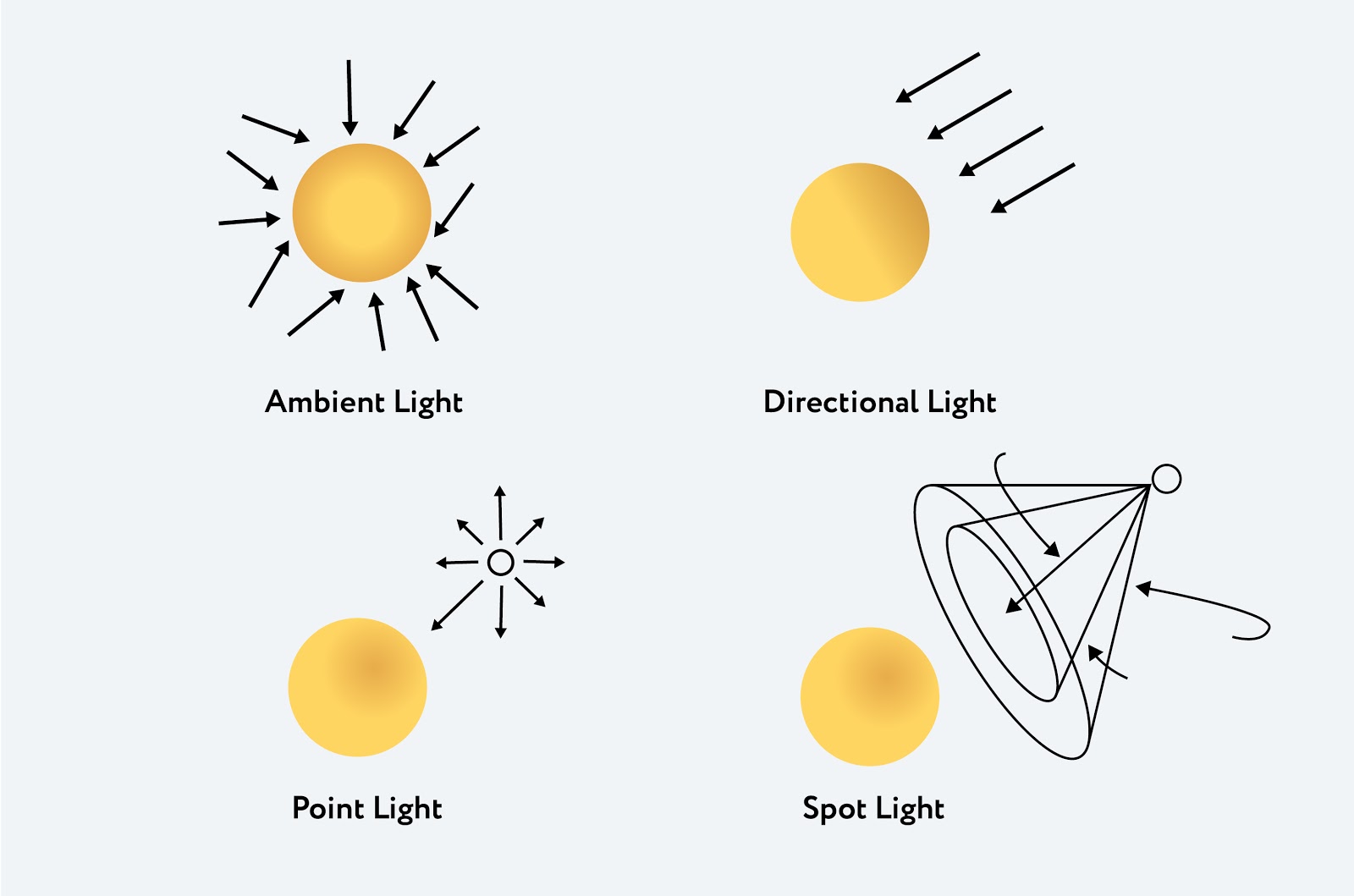
Creating objects on stage
The object created on stage is called Mesh.Mesh is a class that represents objects based on a triangular polygonal mesh.This class takes 2 arguments:- Geometry - describes the shape (position of vertices, faces, radius, etc.)
- Material - describes the appearance of objects (color, texture, transparency, etc.)
Let's try to create 2 simple shapes: a cube and a sphere.First, go to the site three.js , download the latest version of the library. Then we connect the library in the head section or to the beginning of the body section of our document, and you're done:<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8>
<title>First Three.js app</title>
<style>
body { margin: 0; }
canvas { width: 100%; height: 100% }
</style>
</head>
<body>
<script src="js/three.js"></script>
<script>
</script>
</body>
</html>
Further, so that we can display the created object, it is necessary to create a scene, add a camera and configure the render.Add scene:var scene = new THREE.Scene();
Add a perspective camera:var camera = new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 0.1, 1000 );
The camera takes on 4 parameters, which were mentioned above:- angle of view or FOV, in our case it is a standard angle of 75;
- the second parameter is the aspect ratio or aspect ratio;
- the third and fourth parameters are the minimum and maximum distance from the camera, which will fall into the rendering.
Add and configure the render:var renderer = new THREE.WebGLRenderer();
renderer.setSize( window.innerWidth, window.innerHeight );
document.body.appendChild( renderer.domElement );
What we did: first create a render object, then set its size according to the size of the visible area, and finally add it to the page to create an empty canvas element that we will work with.After creating the render, we indicate where to display the canvas tag . In our case, we added it to the body tag .To create the cube itself, we first set the geometry:var geometry = new THREE.BoxGeometry( 10, 10, 10);
A cube is created using the BoxGeometry class . This is a class that contains the vertices and faces of a cube. We transfer the sizes:- width : cube width, size of sides along the x axis
- height : cube height i.e. y-side size
- depth : depth of the cube i.e. side size on the Z axis
To color the cube, set the material:var material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } );
In our case, MeshBasicMaterial is set and the color parameter 0x00ff00 is passed , i.e. green color. This material is basically used to give the figure a uniform color. The downside is that the figure disappears in depth. But this material is quite useful when rendering wireframes using the {wireframe: true} parameter .Now we need a Mesh object that takes geometry and applies material to it:var cube = new THREE.Mesh( geometry, material );
scene.add( cube );
camera.position.z = 25;
Add Mesh to the scene and move the camera back, since all objects after the scene.add () method are added by default with coordinates (0,0,0), due to which the camera and the cube will be at the same point.In order to animate the cube, we need to draw everything inside the render loop using requestAnimationFrame :function render() {
requestAnimationFrame( render );
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render( scene, camera );
}
render();
requestAnimationFrame is a request to the browser that you want to animate something. We pass it a function to call, that is, the render () function.Here we set the parameters of the rotation speed. As a result, the loop renders our scene 60 times per second and causes the cube to rotate.Now let's draw a sphere:var geometry = new THREE.SphereGeometry(1, 32, 32);
To build a sphere, the SphereGeometry class is used , which takes care of:- radius (default value is 1)
- widthSegments - the number of horizontal segments (triangles). The minimum value is 3, the default value is 8
- heightSegments - the number of vertical segments. The minimum value is 2, the default value is 6
By the way, the more you specify the number of triangles, the smoother the surface of the sphere will be.Next, we try to use another material - MeshNormalMaterial - a multicolor material that compares normal vectors in RGB colors:var material = new THREE.MeshNormalMaterial();
var sphere = new THREE.Mesh( geometry, material );
scene.add( sphere );
camera.position.z = 3;
There are a lot of types of material. Some materials can be combined and applied simultaneously to one figure. Read more here .The last step is to set the rendering cycle:function render() {
requestAnimationFrame( render );
sphere.rotation.x += 0.01;
sphere.rotation.y += 0.01;
renderer.render( scene, camera );
}
render();
And we get the following:Let's try to create a more complex figure, and apply more complex material.As an example, take the material MeshPhongMaterial , which takes into account the illumination. Therefore, first we need to add some light to the scene. Below we add a SpotLight with a yellow tint and set its position on the coordinate axis:var scene = new THREE.Scene();
var spotLight = new THREE.SpotLight(0xeeeece);
spotLight.position.set(1000, 1000, 1000);
scene.add(spotLight);
var spotLight2 = new THREE.SpotLight(0xffffff);
spotLight2.position.set( -200, -200, -200);
scene.add(spotLight2);
SpotLight , as mentioned above, radiates from one point in one direction, along a cone, expanding as you move away from the light source. In addition to color, spot light can take on arguments: intensity, distance, angle, penumbra, decay , and also cast shadows.You can read about other types of light and their capabilities here .Now define the shape itself:var geometry = new THREE.TorusGeometry( 10, 3, 16, 100 );
The TorusGeometry class is designed to build tori or “rollers”. This class takes on the following parameters:- radius, default is 1;
- pipe diameter, default 0.4;
- radialSegments or the number of triangle segments, defaults to 8;
- tubularSegments or number of face segments, default is 6
Add material:var material = new THREE.MeshPhongMaterial( {
color: 0xdaa520,
specular: 0xbcbcbc,
} );
This material is intended for shiny surfaces. We give it a golden color, and add a specular property , which affects the luster of the material and its color. The default color is 0x111111 - dark gray.Render, and here’s what we ended up with:A little more about Three.js features
To include Three.js in a project, you just need to run npm install three .If you combine files using Webpack or Browserify , which allow you to implement require ('modules') in the browser, combining all your dependencies, you have the option to import the module into your source files and continue to use it in normal mode:var THREE = require('three');
var scene = new THREE.Scene();
...
It is also possible to use ES6 syntax import :import * as THREE from 'three';
const scene = new THREE.Scene();
...
Or, if you want to import only certain parts of the Three.js library, for example Scene :import { Scene } from 'three';
const scene = new Scene();
...
Conclusion
With the help of almost a couple of lines of code, we created 2 simple shapes, and one is a bit more complicated. Naturally, Three.js has a lot more features. Three.js has a lot of shapes inside the box, materials, types of lighting, etc. This is just a small part of the basics.Three.js library allows you to create and create really healthy things. Here are some sticky examples:Example 1Example 2Example 3If you want to start learning 3D in JavaScript, you can find everything you need here or here .