When processing an incoming HTTP request, a large number of actions are required, such as:
- Logging an incoming HTTP request
- Validation of the HTTP method
- Authentication (basic, MS AD, ...)
- Token validation (if necessary)
- Reading the body of an incoming request
- Reading the header of the incoming request
- Actually processing the request and generating a response
- Install HSTS Strict-Transport-Security
- Setting Content-Type for outgoing response
- Logging outgoing HTTP response
- Record header of the outgoing response
- Record Outgoing Response Body
- Error handling and logging
- Defer recovery processing for recovery after a possible panic
Most of these actions are typical and do not depend on the type of request and its processing.
For productive operation, for each action it is necessary to provide error handling and logging.
Repeating all this in every HTTP handler is extremely inefficient.
Even if you put all the code into separate subfunctions, you still get about 80-100 lines of code for each HTTP handler without taking into account the actual processing of the request and the formation of the response .
The following describes the approach I use to simplify the writing of HTTP handlers in Golang without the use of code generators and third-party libraries.
backend Golang
.
, , โ , - .
HTTP
UML HTTP EchoHandler.
, , :
- defer . UML โ RecoverWrap.func1, .
- . HTTP handler. UML Process โ .
- HTTP handler. UML EchoHandler.func1 โ .
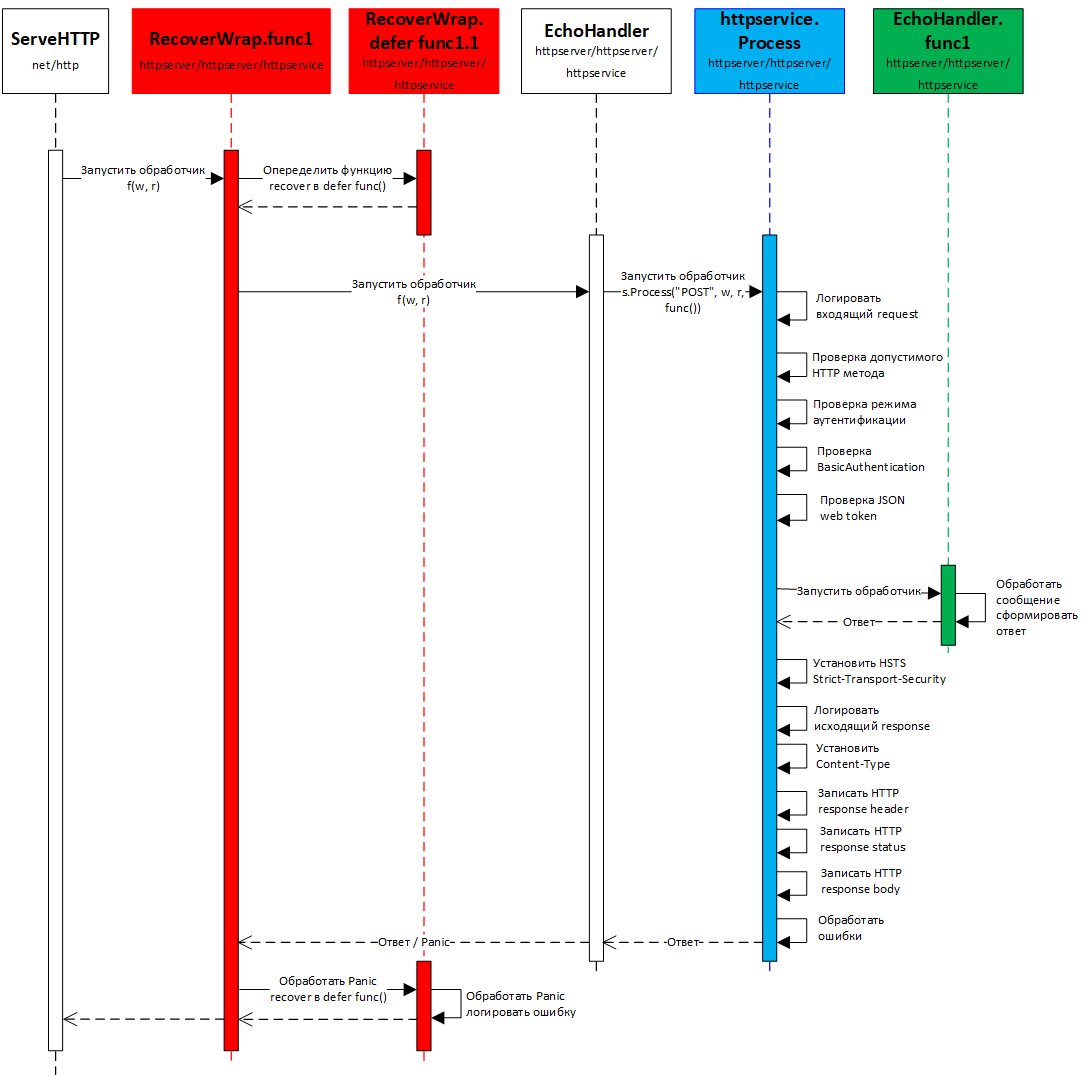
.
HTTP EchoHandler, "" .
, EchoHandler, ( RecoverWrap), EchoHandler.
router.HandleFunc("/echo", service.RecoverWrap(http.HandlerFunc(service.EchoHandler))).Methods("GET")
RecoverWrap .
defer func() EchoHandler.
func (s *Service) RecoverWrap(handlerFunc http.HandlerFunc) http.HandlerFunc {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
defer func() {
var myerr error
r := recover()
if r != nil {
msg := "HTTP Handler recover from panic"
switch t := r.(type) {
case string:
myerr = myerror.New("8888", msg, t)
case error:
myerr = myerror.WithCause("8888", msg, t)
default:
myerr = myerror.New("8888", msg)
}
s.processError(myerr, w, http.StatusInternalServerError, 0)
}
}()
if handlerFunc != nil {
handlerFunc(w, r)
}
})
}
, EchoHandler. , HTTP Process .
func (s *Service) EchoHandler(w http.ResponseWriter, r *http.Request) {
_ = s.Process("POST", w, r, func(requestBuf []byte, reqID uint64) ([]byte, Header, int, error) {
header := Header{}
for key := range r.Header {
header[key] = r.Header.Get(key)
}
return requestBuf, header, http.StatusOK, nil
})
}
HTTP Process. :
- method string โ HTTP HTTP
- w http.ResponseWriter, r *http.Request โ
- fn func (requestBuf [] byte, reqID uint64) ([] byte, Header, int, error) - the processing function itself, it receives an incoming request buffer and a unique HTTP request number (for logging purposes), returns a prepared outgoing response buffer , header of the outgoing response, HTTP status and error.
func (s *Service) Process(method string, w http.ResponseWriter, r *http.Request, fn func(requestBuf []byte, reqID uint64) ([]byte, Header, int, error)) error {
var myerr error
reqID := GetNextRequestID()
if s.logger != nil {
_ = s.logger.LogHTTPInRequest(s.tx, r, reqID)
mylog.PrintfDebugMsg("Logging HTTP in request: reqID", reqID)
}
mylog.PrintfDebugMsg("Check allowed HTTP method: reqID, request.Method, method", reqID, r.Method, method)
if r.Method != method {
myerr = myerror.New("8000", "HTTP method is not allowed: reqID, request.Method, method", reqID, r.Method, method)
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Check authentication method: reqID, AuthType", reqID, s.cfg.AuthType)
if (s.cfg.AuthType == "INTERNAL" || s.cfg.AuthType == "MSAD") && !s.cfg.UseJWT {
mylog.PrintfDebugMsg("JWT is of. Need Authentication: reqID", reqID)
username, password, ok := r.BasicAuth()
if !ok {
myerr := myerror.New("8004", "Header 'Authorization' is not set")
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Get Authorization header: username", username)
if myerr = s.checkAuthentication(username, password); myerr != nil {
mylog.PrintfErrorInfo(myerr)
return myerr
}
}
if s.cfg.UseJWT {
mylog.PrintfDebugMsg("JWT is on. Check JSON web token: reqID", reqID)
cookie, err := r.Cookie("token")
if err != nil {
myerr := myerror.WithCause("8005", "JWT token does not present in Cookie. You have to authorize first.", err)
mylog.PrintfErrorInfo(myerr)
return myerr
}
if myerr = myjwt.CheckJWTFromCookie(cookie, s.cfg.JwtKey); myerr != nil {
mylog.PrintfErrorInfo(myerr)
return myerr
}
}
mylog.PrintfDebugMsg("Reading request body: reqID", reqID)
requestBuf, err := ioutil.ReadAll(r.Body)
if err != nil {
myerr = myerror.WithCause("8001", "Failed to read HTTP body: reqID", err, reqID)
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Read request body: reqID, len(body)", reqID, len(requestBuf))
mylog.PrintfDebugMsg("Calling external function handler: reqID, function", reqID, fn)
responseBuf, header, status, myerr := fn(requestBuf, reqID)
if myerr != nil {
mylog.PrintfErrorInfo(myerr)
return myerr
}
if s.cfg.UseHSTS {
w.Header().Add("Strict-Transport-Security", "max-age=63072000; includeSubDomains")
}
if s.logger != nil {
mylog.PrintfDebugMsg("Logging HTTP out response: reqID", reqID)
_ = s.logger.LogHTTPOutResponse(s.tx, header, responseBuf, status, reqID)
}
mylog.PrintfDebugMsg("Set HTTP response headers: reqID", reqID)
if header != nil {
for key, h := range header {
w.Header().Set(key, h)
}
}
mylog.PrintfDebugMsg("Set HTTP response status: reqID, Status", reqID, http.StatusText(status))
w.WriteHeader(status)
if responseBuf != nil && len(responseBuf) > 0 {
mylog.PrintfDebugMsg("Writing HTTP response body: reqID, len(body)", reqID, len(responseBuf))
respWrittenLen, err := w.Write(responseBuf)
if err != nil {
myerr = myerror.WithCause("8002", "Failed to write HTTP repsonse: reqID", err)
mylog.PrintfErrorInfo(myerr)
return myerr
}
mylog.PrintfDebugMsg("Written HTTP response: reqID, len(body)", reqID, respWrittenLen)
}
return nil
}