This post is the second in a series of articles on auto-tracking - the new reactivity system in Ember.js. I also discuss the concept of reactivity in general, and how it manifests itself in JavaScript.
From a translator: Chris Garrett - works for LinkedIn and is one of the core contributors to the Ember js framework. He took an active part in creating the new edition of the framework - Ember Octane . One of the cornerstones of this edition is the new reactivity system based on automatic tracking (autotracking). Despite the fact that his series was written for Ember developers, it touches on concepts that are useful for all web programmers to know.
- What is reactivity?
- What makes a reactive system good? β This post
- How auto tracking works
- Auto Tracking Case - TrackedMap
- Case for auto tracking - @localCopy
- Auto Tracking Case - RemoteData
- Case for auto tracking - effect ()
, . , :
: , .
, , . : ?
, , . . , , . , , .
, , . , , . ! , , - ( ).
HTML
HTML . , , . HTML ( CSS) , - JavaScript!
-, HTML ? ? HTML :
<form action="/my-handling-form-page" method="post">
<label>
Email:
<input type="email" />
</label>
<label>
Password:
<input type="password" />
</label>
<button type="submit">Log in</button>
</form>
. . β , , - . , , .
ββ : , , , . HTML , , , β (JavaScript). , HTML ? , HTML ?
HTML, input
select
. , , , . , , .
<style>
input[type='checkbox'] + ul {
display: none;
}
input[type='checkbox']:checked + ul {
display: inherit;
}
</style>
<nav>
<ul>
<li>
<label for="dropdown">Dropdown</label>
<input id="dropdown" type="checkbox" />
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
</li>
</ul>
</nav>
β Β« , CSSΒ». . ./index.html
- HTML / CSS .
HTML (, checked ). HTML- , . , , :
1. , , ,
, , HTML- . - , 10 , , .
. - . , JS .
push
push. , , . , JavaScript, .
. , , , , , - . , - <edit-word>
:
customElements.define('edit-word',
class extends HTMLElement {
constructor() {
super();
const shadowRoot = this.attachShadow({mode: 'open'});
this.form = document.createElement('form');
this.input = document.createElement('input');
this.span = document.createElement('span');
shadowRoot.appendChild(this.form);
shadowRoot.appendChild(this.span);
this.isEditing = false;
this.input.value = this.textContent;
this.form.appendChild(this.input);
this.addEventListener('click', () => {
this.isEditing = true;
this.updateDisplay();
});
this.form.addEventListener('submit', e => {
this.isEditing = false;
this.updateDisplay();
e.preventDefault();
});
this.input.addEventListener('blur', () => {
this.isEditing = false;
this.updateDisplay();
});
this.updateDisplay()
}
updateDisplay() {
if (this.isEditing) {
this.span.style.display = 'none';
this.form.style.display = 'inline-block';
this.input.focus();
this.input.setSelectionRange(0, this.input.value.length)
} else {
this.span.style.display = 'inline-block';
this.form.style.display = 'none';
this.span.textContent = this.input.value;
this.input.style.width = this.span.clientWidth + 'px';
}
}
}
);
- , . isEditing
, updateDisplay
span
form
. , . , updateDisplay
.
, , isEditing
. , . :
2.
isEditing
, . , , β .
, , .
Ember
Ember Classic push. (observers) (evert listeners) , , , . , (binding), (dependency chaining), .
fullName
:
import { computed, set } from '@ember/object';
class Person {
firstName = 'Liz';
lastName = 'Hewell';
@computed('firstName', 'lastName')
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
let liz = new Person();
console.log(liz.fullName); 'Liz Hewell';
set(liz, 'firstName', 'Elizabeth');
console.log(liz.fullName); 'Elizabeth Hewell';
Classic Ember . , , , Ember . , set()
, .

, (observers) , (computed) (templates) . , , , . ( ) .
Ember , , . . , - . , , . - () β , , .
, , , . , , . , , .
Observables, Streams Rx.js
push, , β Observable. JavaScript RxJS Angular .
, . , , , .
let count = 0;
document.addEventListener(
'click',
() => console.log(`Clicked ${++count} times`)
);
import { fromEvent } from 'rxjs';
import { scan } from 'rxjs/operators';
fromEvent(document, 'click')
.pipe(scan(count => count + 1, 0))
.subscribe(count => console.log(`Clicked ${count} times`));
Ember, β , . , , .
, . , , , . , .

, (debounce), , . :
3.
, . , , . , , , , .
, , push, . (lazy) , , , Ember Classic, . , , push, , .
, , . pull.
pull
, , pull, β . , , . , , , - , . , , , .
, , pull. , , . , .
, pull , . , Β«Virtual DOMΒ».
React VirtualDOM
Virtual DOM, , React.js . , HTML . , , , HTML, React , , HTML.
HTML-. , . -.

, React, , - . , setState
API ( useState
).
class Toggle extends React.Component {
state = { isToggleOn: true };
handleClick = () => {
this.setState(state => ({
isToggleOn: !state.isToggleOn
}));
}
render() {
return (
<button onClick={this.handleClick}>
{this.state.isToggleOn ? 'ON' : 'OFF'}
</button>
);
}
}
, ( ) .

, (consistency), , setState
useState
. , ( ). - React , :
4.
React , , . , React :
class Example extends React.Component {
state = {
value: 123;
};
render() {
let part1 = <div>{this.state.value}</div>
this.setState({ value: 456 });
let part2 = <div>{this.state.value}</div>
return (
<div>
{part1}
{part2}
</div>
);
}
}
, , part1
, part2
. , , - , . , , . React , .
, React , . API, shouldComponentUpdate()
useMemo()
, React .
API , ββ . , .
Vue:
Vue Virtual DOM, . Vue data
:
const vm = new Vue({
data: {
a: 1
}
});
Vue setState
useState
( , API), . data
, , . observables.
, :
const vm = new Vue({
el: '#example',
data: {
message: 'Hello'
},
computed: {
reversedMessage() {
return this.message.split('').reverse().join('')
}
}
})
reversedMessage
message
, message
.
Vue , React, . , (memoization) , - , . push, , .
Elm
, , JavaScript. , , (autotracking) , , - .
Elm β , . , ( HTML + JS). , - .
, Elm - , . , Elm .
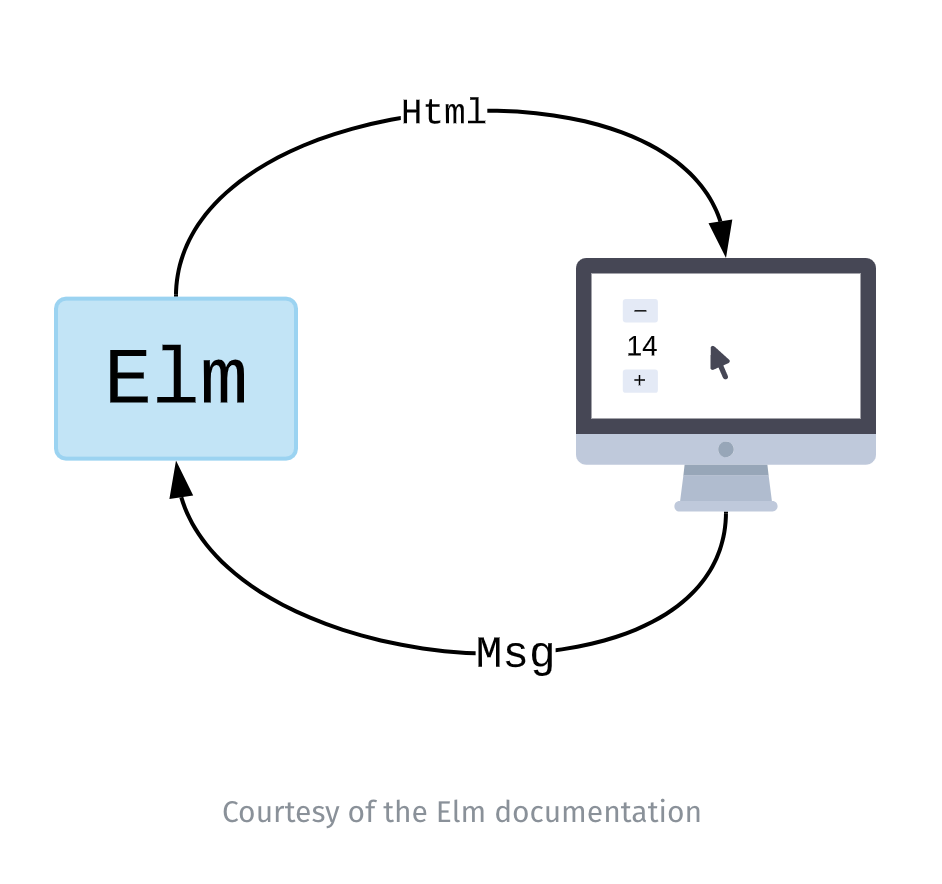
- Elm , (memoization). , . / , .
let lastArgs;
let lastResult;
function memoizedRender(...args) {
if (deepEqual(lastArgs, args)) {
return lastResult;
}
lastResult = render(...args);
lastArgs = args;
return lastResult;
}
«» , , Elm .
. , HTML , React / Vue / Virtual DOM.
, , , . Elm, , - .
, Elm JavaScript . JavaScript, , . , . Redux β , , .
, , β , , . , .
!
, , :
- HTML / CSS
- push
- JavaScript
β Ember
β Observables / Rx.js - pull
β React.js
β Vue.js
β Elm
:
- , , ,
- Reading a state in a system leads to a reactive derivative state
- The system minimizes unnecessary work by default.
- System prevents conflicting derived state
I do not claim that this list is exhaustive, but it covers much of what makes reactive systems reliable and usable. In the next post, we will delve into auto tracking and find out how it implements these principles.