Problem
One of Java's problems is its verbosity and the amount of standard code needed. This is common knowledge.
Let's look at a simple Cat class in Java. We want each Cat object to have the following attributes (fields):
- Name
- Number of lives
- Colour
Pretty simple, right? Now let's look at the code in Java. For simplicity, let's make our class immutable (immutable) - without setters, we will configure everything in our constructor.
public final class Cat {
private final String name;
private final int numberOfLives;
private final String color;
public Cat(String name, int numberOfLives, String color) {
this.name = name;
this.numberOfLives = numberOfLives;
this.color = color;
}
public String getName() {
return name;
}
public int getNumberOfLives() {
return numberOfLives;
}
public String getColor() {
return color;
}
}
It's already quite long, isn't it?
. equals() hashCode().
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Cat cat = (Cat) o;
return numberOfLives == cat.numberOfLives &&
Objects.equals(name, cat.name) &&
Objects.equals(color, cat.color);
}
@Override
public int hashCode() {
return Objects.hash(name, numberOfLives, color);
}
? , toString():
@Override
public String toString() {
return "Cat{" +
"name='" + name + '\'' +
", numberOfLives=" + numberOfLives +
", color='" + color + '\'' +
'}';
}
. ! ( IDE ) . , (, ) .
, :
private final String name;
private final int numberOfLives;
private final String color;
β , . IDE , , Lombok, .
Java , , Cat. β , , equals(), hashCode() toString(). , , . β , . . , hashCode() equals()?
Records
Java 14 , Record, JEP 359: Records (Preview).
50 , :
public record Cat(String name, int numberOfLives, String color) { }
, ?
, β :
- equals(), hashCode() toString()
, .
public final class Cat extends java.lang.Record {
private final java.lang.String name;
private final int numberOfLives;
private final java.lang.String color;
public Cat(java.lang.String name, int numberOfLives, java.lang.String color) { }
public java.lang.String toString() { }
public final int hashCode() { }
public final boolean equals(java.lang.Object o) { }
public java.lang.String name() { }
public int numberOfLives() { }
public java.lang.String color() { }
}
, Cat. , , β getColor() color().
java.lang.Record.
equals() , . toString() :
Cat[name=Fluffy, numberOfLives=9, color=White]
, , .
, .
, . , , , . :
public record Cat(String name, int numberOfLives, String color) {
public boolean isAlive() {
return numberOfLives >= 0;
}
}
.
(Custom)
, . , Cat :
Cat cat = new Cat("Fluffy", 9, "White");
, β , .
, , 9. , , 9 . , .
public record Cat(String name, int numberOfLives, String color) {
public Cat(String name, String color) {
this(name, 9, color);
}
}
. . , . , , :
public record Cat(String name, int numberOfLives, String color) {
public Cat(String name,int numberOfLives, String color) {
if(numberOfLives < 0) {
throw new IllegalArgumentException("Number of lives cannot be less than 0.");
}
if(numberOfLives > 9) {
throw new IllegalArgumentException("Cats cannot have that many lives.");
}
this.name = name;
this.numberOfLives = numberOfLives;
this.color = color;
}
}
, ( ), . , .
public record Cat(String name, int numberOfLives, String color) {
public Cat {
}
}
java.lang.Class , , .
isRecord(). , , - :
Cat cat = new Cat("Fluffy", 9, "White");
if(cat.getClass().isRecord()) {
}
getRecordComponents(). , . java.lang.reflect.RecordComponent. , , :
!
, , Java 14 ( 2/2020).
Preview feature ( )
(Records) Java 14. . ?
VM β Java SE, , , . JDK ; , Java SE.
JDK , , Java SE , , . , ( ), ( ), .
JDK, . . , , , .
, JDK 14.
IntelliJ IDEA
IntelliJ IDEA Preview feature File β Project Structure.
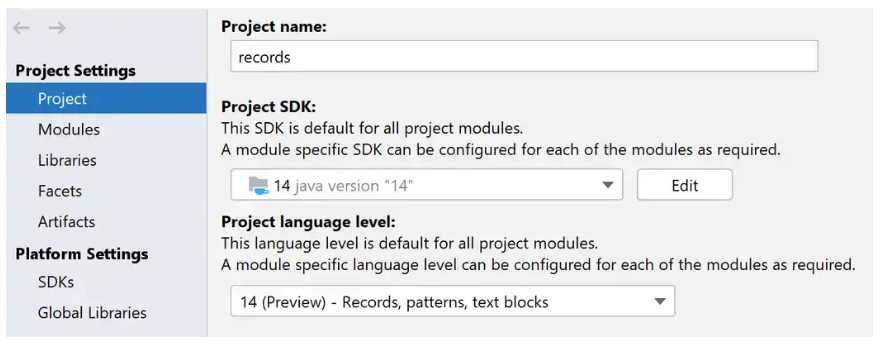
To use entries in IntelliJ IDEA, you will need version 2020.1 and later. As of 2/2020, it is available as an Early Access Program build . IDEA currently has basic support for recordings, but full support should be available in the release version.
Manual compilation
An alternative is to manually assemble the project. Then you need to provide the following options for javac:
javac --release 14 --enable-preview ...
This is for compilation. At runtime, you simply provide --enable-preview
java --enable-preview ...
Maven projects
For Maven builds, you can use the following configuration:
<build>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<release>14</release>
<compilerArgs>
--enable-preview
</compilerArgs>
```14</source>
<target>14</target>
</configuration>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<argLine>--enable-preview</argLine>
</configuration>
</plugin>
<plugin>
<artifactId>maven-failsafe-plugin</artifactId>
<configuration>
<argLine>--enable-preview</argLine>
</configuration>
</plugin>
</plugins>
</build>