The Chrome Developer Tools Console is probably one of the most widely used and most useful specialized browser tools. The console gives the programmer many interesting features. It helps in debugging, profiling, and monitoring page code. The material we are translating today is dedicated to telling you about some of the features of the Chrome console that are not as widely known as they deserve.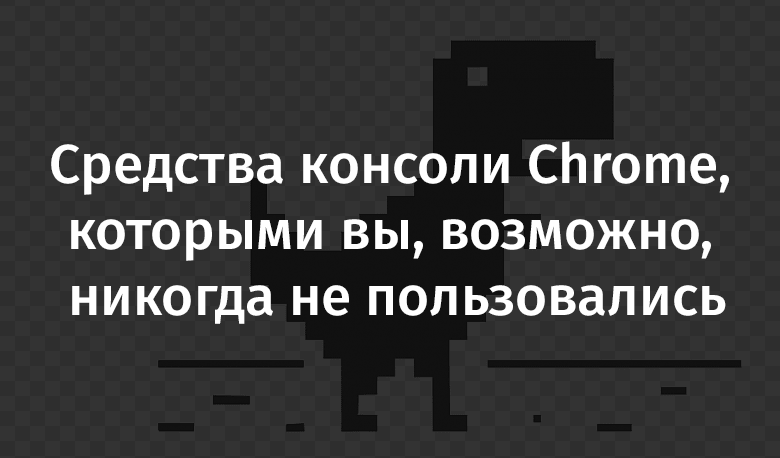
Monitor function
The function is monitor
used to organize monitoring of other functions. In particular, it allows you to find out when a certain function was called, and with what parameters it was called. Consider an example (its code is entered in the Chrome console):function traceFunc (arg) { }
monitor(traceFunc)
The function is traceFunc
passed to the function monitor
as an argument. Now, when the function traceFunc
is called, we will receive a notification about this (again, in the console):traceFunc(90)
function traceFunc was called with arguments: 90
Using the monitor functionUnmonitor function
The function unmonitor
allows you to disable monitoring of a function that was previously monitored using the function monitor
. In order to stop monitoring the function traceFunc
, we use the following construction:unmonitor(traceFunc)
If you call after this traceFunc
, then no notifications will be displayed in the console.traceFunc(90)
Calling a function whose monitoring is disabled does not result in outputting information about this call to the consoleMonitorEvents function
The scheme for using the function monitorEvents
looks like this:monitorEvents(object,[,events])
This function allows you to track the occurrence of object events and displays information about these events in the console.Suppose there is a button on the page described by the following code:<button id="button">Button</button>
Let's start tracking the event of click
this button:monitorEvents(button, "click")
If after starting the observation of an event, click
click on the button - information about the event will be displayed in the console.Button and start monitoring its events click Youcan monitor several events by passingmonitorEvents
an array asfunctions of thesecond argument:monitorEvents(button, ["click", "mouseover"])
Now under observation are events click
and mouseover
. As a result, the console receives messages about clicking on the button, and that it was held over the mouse.Monitoring events of the click and mouseover buttonsYou can organize monitoring of event groups:monitorEvents(button, ["click", "mouse"])
A string mouse
is a universal identifier for a group of mouse-related events. For example - mouseover
, mousemove
, mouseout
. As in the case of subscribing to specific events, subscribing to a universal event identifier will lead to the issuance of notifications when such events occur.Monitoring button events related to clicking on it and with the mouseOf course, the function ismonitorEvents
applicable to other objects, and not just buttons. The same applies to events that can be watched with it. These are not onlyclick
mouse events.UnmonitorEvents function
The function unmonitorEvents
allows you to cancel the monitoring of object events started by the function monitorEvents
.Suppose we decide to track button clicks:monitorEvents(button, "click")
As a result, when the button is clicked, information about these events gets to the console. In order to stop monitoring, we need this design:unmonitorEvents(button, "click")
Now the system stops monitoring the click
button event .After refusing to monitor click events, reports about clicks on a button cease to get to the console$ _ Construction
The design $_
allows you to access the result of the last expression executed in the console.Suppose we entered an expression in the console 2 + 2
and executed it. It gave us 4
.Then we entered and executed the expression 3*2
. It gave us 6
.It turned out that we performed two expressions, the most recent of which is 3*2
. If now enter into the console $_
and click Enter
- the result of the expression is displayed 3*2
, that is - 6
.Using the $ _ constructCopy function
The function copy
allows you to copy the data transferred to it to the clipboard.Let's execute, for example, the following command:copy("nnamdi")
This will copy the line to the clipboard nnamdi
. In order to check this, execute the above command in the console, and then try pasting what is on the clipboard into the address bar of the browser. You should get something that resembles the following image.Software copying data to the clipboardClear function
The function clear
allows you to clear the history of command execution in the console. This function is useful in cases when the console is full of various materials displayed in it, and you would like to remove from it everything that is displayed in it.Keys function
The function keys(object)
, like the method Object.keys
, returns an array of object property keys:const obj = {prop1: 0, prop2: 1, prop3: 2}
keys(obj)
Using the keys functionValues function
The function values
is similar to a method Object.values
. It returns an array of object property values.const obj = {prop1: 0, prop2: 1, prop3: 2}
values(obj)
Using the values functionGetEventListeners function
The function getEventListeners
returns information about events registered for the object. For example, if you register certain events for a button, the function getEventListeners
passed to this button will return an object containing, in the form of arrays, a description of the button's events.Suppose the page has this code:<button id="button"
onclick="clickHandler()"
onmousedown="mousedownHandler()"
onmouseover="mouseoverHandler()">
Button
</button>
<script>
function clickHandler() {
}
function mousedownHandler() {
}
function mouseoverHandler() {
}
</script>
This code describes the button and three events: click
, onmousedown
, and onmouseover
. Events are assigned handlers clickHandler
, mousedownHandler
and mouseoverHandler
.Call the following command in the console:getEventListeners(button)
Information about button event handlersAn object issued after a command executesgetEventListeners(button)
has propertiesclick
,mousedown
andmouseover
, whose names correspond to the names of events whose handlers are assigned to the button. Properties contain arrays of objects containing detailed information about events.Function $
The function $
returns the first of the DOM elements that match the selector passed to it. The function call diagram looks like this:$(elementName, [,node])
Suppose the page has the following code:<button id="button1" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button1</button>
<button id="button2" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button2</button>
<button id="button3" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button3</button>
<button id="button4" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button4</button>
This code describes four buttons.Let's execute the following command:$("button")
The following shows what gets into the console after its execution.The result of the $ ("button")commandButton1
.As you can see, the command returned a button. This button is the first elementbutton
in the document. After we have at our disposal a DOM node of an element, we can refer to its properties and methods.For example, in order to find out the identifier of a button, you can use this construction:$("button").id
Clarification of the identifier of the buttonUsing the function$
, you can specify the DOM node, the first element of which we are interested in.Suppose buttonsButton2
,Button3
andButton4
from the previous example are placed in an element<div>
:<button id="button1" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button1</button>
<div>
<button id="button2" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button2</button>
<button id="button3" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button3</button>
<button id="button4" onclick="clickHandler()" onmousedown="mousedownHandler()" onmouseover="mouseoverHandler()">Button4</button>
</div>
To select the first button from those that are nested in <div>
, you can use the following command:$("button", document.querySelector("div"))
Accessing an element nested in another elementAs you can see, it now$
returns a buttonButton2
, since this is the first elementbutton
nested in the element<div>
Function $$
The function $$
works the same as the above function $
. But it returns all the matching elements. It is called like this:$$(element, [,node])
Suppose there are four buttons on a page, as in the previous examples.Call the following command:$$("button")
Using the $$ functionAfter the call$$
, as seen in the previous figure, we have at our disposal an array of elementsbutton
.Elements can also be selected from the one specified when$$
the DOM node iscalled. For example, if a page has several buttons enclosed in<div>
, we can, to select all these buttons, use this design:$$("button", document.querySelector("div"))
Using the $$ function to select items nested in another itemSummary
The Chrome console is a useful tool. As new versions of Chrome become available, the capabilities of its console expand. Therefore, it is useful to periodically look here in search of something new and interesting.Dear readers! What Chrome console tools do you use most often?