Recently, an article from @sanReal appeared on Habr , where Alexander talked about what techniques and capabilities of Svelte he learned from his own experience. I was a little surprised not to see in his list the mention of one of the most powerful framework tools - Actions . In addition, communicating with people in the @sveltejs community who are already creating very good applications using Svelte, I sometimes notice that not everyone uses Actions, even where their application would ideally solve the problem. In this article I will tell you what Actions are and, with the simplest examples, I will show their application.
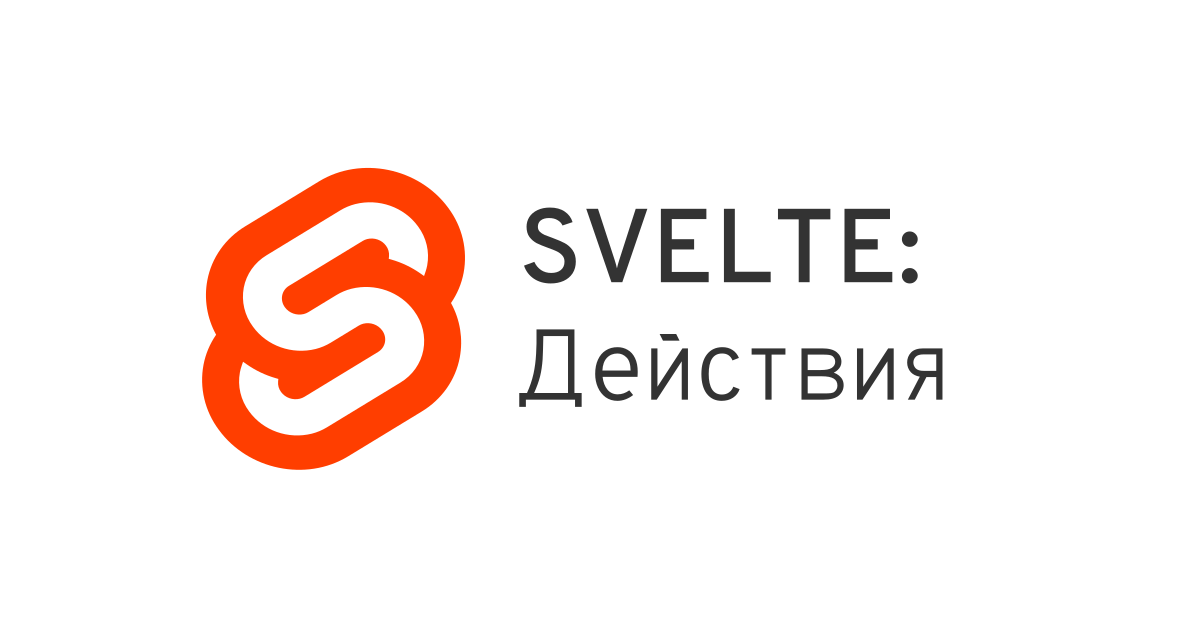
Suppose we have a text box in which the user must enter a phone number. We need to ensure that the user can enter only numbers in the field.
: +7 <input type="text" />
When the task is to endow a standard HTML element with additional features, it is common practice when working in any component of the framework to create a reusable component around this element, inside which the logic of non-standard behavior is implemented. You can do the same in Svelte:
<script>
function clean_value(e){
e.target.value = e.target.value.replace(/[^\d]/g,'');
}
</script>
<input type="text" on:input={clean_value} />
...
: +7 <InputDigits />
View in action
The task has been completed, but the element itself is <input/>
now hidden inside the component, so we will no longer be able to manipulate it as a regular HTML element - to "hang" on it any CSS classes, event handlers, or some special attributes will not be so simple.
Svelte — ( Actions). Svelte , , , , — "" , . — - HTML-. , DOM. DOM-, .
:
<script>
function onlydigits(node) {
function clean_value(){
node.value = node.value.replace(/[^\d]/g,'');
}
node.addEventListener('input',clean_value);
return {
destroy: ()=>node.removeEventListener('input',clean_value)
}
}
</script>
: +7 <input type="text" use:onlydigits />
onlydigits
, . , DOM- node
input
. , destroy
, DOM-, .
Svelte, - HTML- , use
. <input/>
use:onlydigits
.
. , 10 :
<script>
function onlydigits(node) { ... }
function max10symbols(node) {
function trim_value(){
node.value = node.value.substring(0, 10);
}
node.addEventListener('input',trim_value);
return {
destroy: ()=>node.removeEventListener('input',trim_value)
}
}
</script>
: +7 <input type="text" use:onlydigits use:max10symbols />
<input />
— use:onlydigits
use:max10symbols
. .
use
, . , . :
<script>
function format_by_pattern(node,pattern) {
function set_cursor(){
const match = node.value.match(/[\d]/gi);
const pos = match ? node.value.lastIndexOf(match.pop())+1 : 0;
node.setSelectionRange(pos, pos);
}
function format_value(e){
let digits = node.value.replace(/[^\d]/g,'').split('');
node.value = pattern.replace(/[*]/g,(m)=>digits.shift()||m);
set_cursor();
}
node.addEventListener('input',format_value);
format_value();
return {
destroy: ()=>node.removeEventListener('input',format_value)
}
}
</script>
: +7 <input type="text" use:format_by_pattern={'(***) ***-**-**'} /> <br/>
: <input type="text" use:format_by_pattern={' **** №******'} />
<input />
HTML-. format_by_pattern
, . , Svelte, JavaScript-.
, , , . .
<script>
function format_by_pattern(node,pattern) {
function set_cursor(){...}
function format_value(){...}
...
return {
destroy: ...,
update: (new_pattern)=>{
pattern=new_pattern;
format_value();
}
}
}
let zagran = false;
</script>
: +7 <input type="text" use:format_by_pattern={'(***) ***-**-**'} /> <br/>
: <input type="text" use:format_by_pattern={zagran ? ' ** №*******' : ' **** №******'} /> <br/>
<input type="checkbox" bind:checked={zagran}/> -
, . , , bind:checked
, zagran
true
false
. , Svelte use:format_by_pattern
.
, , DOM-, <input />
DOM-, . .
, , , — update
. , . .
. , . , , DOM- — , . Svelte. Svelte , , .
Note: Very soon in Moscow will be held Svelte Russia Meetup # 1 . Register, there is still room. For those who will not be able to attend in person, a broadcast and recording of the event will be organized.