Queue
(Warteschlange) - Eine Datenstruktur auf der Festplatte oder im RAM, die Links zu Nachrichten speichert und deren Kopien consumers
(an Verbraucher) weitergibt. Queue
Es ist ein Erlang-Prozess mit einem Status (in dem die Nachrichten selbst zwischengespeichert werden können). 1 Tausend Warteschlangen können ungefähr 80 MB belegen.
Binding
(Bindung) - Eine Regel, die dem Austauscher mitteilt, welche der Warteschlangen Nachrichten empfangen soll.
Inhaltsverzeichnis
Temporäre Warteschlangen
Wenn die Warteschlange mit dem Parametersatz erstellt wird autoDelete
, kann sich diese Warteschlange automatisch selbst löschen . Diese Warteschlangen werden normalerweise erstellt, wenn der erste Client eine Verbindung herstellt, und gelöscht, wenn alle Clients die Verbindung trennen.
exclusive
, . , /, . exclusive
, autoDelete
.
:
durable
, /. Queue.Delete
.
Highly Available
HA RabbitMQ. , , .
- HA , , HA . - , .
HA .

:
- HA . HA HA RabbitMQ (2-3 )
RPC
. Queue.Declare
, :
RabbitMQ.Client:
channel.QueueDeclare(
queue: "my_queue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null
);
queue
— , .durable
— true, /exclusive
— true,autoDelete
— true, arguments
— . .
arguments
x-message-ttl
(x-message-time-to-live
) — . x-message-ttl
, , . x-message-ttl
. . . x-message-ttl
, .x-expires
— . . , , Basic.Cancel
. , Basic.Get
. , . , .x-max-length
— . ,

x-max-lenght-bytes
— . ( )x-overflow
— . : drop-head
( ) reject-publish
. drop-head
, . reject-publish
,x-dead-letter-exchange
— exchange, ,x-dead-letter-routing-key
—x-max-priority
— 255 (RabbitMQ 3.5.0 ). , . ,x-queue-mode
— . . . , , ,x-queue-master-locator
— ,x-ha-policy
— HA . all
, . nodes
,x-ha-nodes
— , HA

, RPC
Queue.DeclareOk
. ( Queue.Declare
), Channel.Close
OperationInterruptedException, .
Queue.Declare
. , , , .
Queue.Declare
, Channel.Close
OperationInterruptedException, 403 .
, >= 10 , , GC , , .
Queue
RabbitMQ
guest
(username: guest
password: guest
). , guest
. Queues
Add a new queue
. :
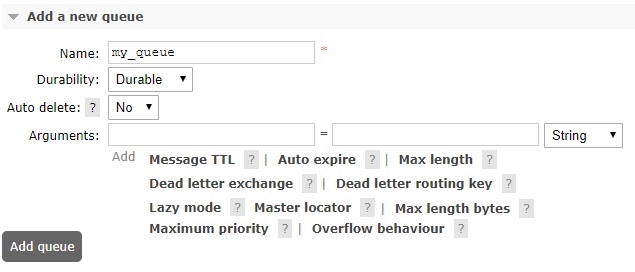
Add queues
, .

. , consumers
, / , .
Binding
RPC
. Queue.Bind
, :
RabbitMQ.Client:
channel.QueueBind(
queue: queueName,
exchange: "my_exchange",
routingKey: "my_key",
arguments: null
);
queue
—exchange
—routingKey
—arguments
—

, RPC
Queue.BindOk
.
Binding
RabbitMQ
guest
(username: guest
password: guest
). , guest
. Queues
my_queue
. bindings
:

Bind
, :
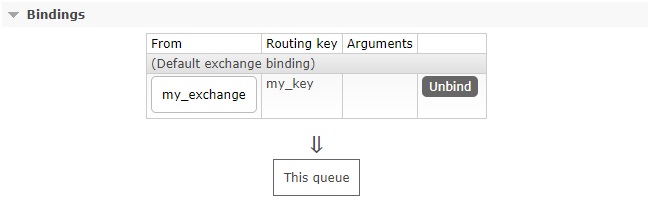
Code
In diesem Abschnitt beschreiben wir die Warteschlange und die Bindung mithilfe von C # -Code. Wenn wir also eine Bibliothek entwickeln müssen. Vielleicht ist es nützlich für die Wahrnehmung.
public interface IQueue
{
string Name { get; }
bool IsDurable { get; }
bool IsExclusive { get; }
bool IsAutoDelete { get; }
IDictionary<string, object> Arguments { get; }
}
public class Queue : IQueue
{
public Queue(
string name,
bool isDurable = true,
bool isExclusive = false,
bool isAutoDelete = false,
IDictionary<string, object> arguments = null)
{
Name = name ??
throw new ArgumentNullException(name, $"{name} must not be null");
IsDurable = isDurable;
IsExclusive = isExclusive;
IsAutoDelete = isAutoDelete;
Arguments = arguments ?? new Dictionary<string, object>();
}
public string Name { get; }
public bool IsDurable { get; }
public bool IsExclusive { get; }
public bool IsAutoDelete { get; }
public IDictionary<string, object> Arguments { get; }
}
public static class QueueMode
{
public const string Default = "default";
public const string Lazy = "lazy";
}
public interface IBinding
{
IExchange Exchange { get; }
string RoutingKey { get; }
IDictionary<string, object> Arguments { get; }
}
public class Binding : IBinding
{
public Binding(
IExchange exchange,
string routingKey,
IDictionary<string, object> arguments)
{
Exchange = exchange;
RoutingKey = routingKey;
Arguments = arguments;
}
public IExchange Exchange { get; }
public string RoutingKey { get; }
public IDictionary<string, object> Arguments { get; }
}