Problem
Eines der Probleme von Java ist die Ausführlichkeit und die Menge des benötigten Standardcodes. Das ist allgemein bekannt.
Schauen wir uns eine einfache Cat- Klasse in Java an. Wir möchten, dass jedes Cat-Objekt die folgenden Attribute (Felder) aufweist:
- Name
- Anzahl der Leben
- Farbe
Ziemlich einfach, oder? Schauen wir uns nun den Code in Java an. Lassen Sie uns der Einfachheit halber unsere Klasse unveränderlich (unveränderlich) machen - ohne Setter werden wir alles in unserem Konstruktor konfigurieren.
public final class Cat {
private final String name;
private final int numberOfLives;
private final String color;
public Cat(String name, int numberOfLives, String color) {
this.name = name;
this.numberOfLives = numberOfLives;
this.color = color;
}
public String getName() {
return name;
}
public int getNumberOfLives() {
return numberOfLives;
}
public String getColor() {
return color;
}
}
Es ist schon ziemlich lang, nicht wahr?
. equals() hashCode().
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Cat cat = (Cat) o;
return numberOfLives == cat.numberOfLives &&
Objects.equals(name, cat.name) &&
Objects.equals(color, cat.color);
}
@Override
public int hashCode() {
return Objects.hash(name, numberOfLives, color);
}
? , toString():
@Override
public String toString() {
return "Cat{" +
"name='" + name + '\'' +
", numberOfLives=" + numberOfLives +
", color='" + color + '\'' +
'}';
}
. ! ( IDE ) . , (, ) .
, :
private final String name;
private final int numberOfLives;
private final String color;
— , . IDE , , Lombok, .
Java , , Cat. — , , equals(), hashCode() toString(). , , . — , . . , hashCode() equals()?
Records
Java 14 , Record, JEP 359: Records (Preview).
50 , :
public record Cat(String name, int numberOfLives, String color) { }
, ?
, — :
- equals(), hashCode() toString()
, .
public final class Cat extends java.lang.Record {
private final java.lang.String name;
private final int numberOfLives;
private final java.lang.String color;
public Cat(java.lang.String name, int numberOfLives, java.lang.String color) { }
public java.lang.String toString() { }
public final int hashCode() { }
public final boolean equals(java.lang.Object o) { }
public java.lang.String name() { }
public int numberOfLives() { }
public java.lang.String color() { }
}
, Cat. , , — getColor() color().
java.lang.Record.
equals() , . toString() :
Cat[name=Fluffy, numberOfLives=9, color=White]
, , .
, .
, . , , , . :
public record Cat(String name, int numberOfLives, String color) {
public boolean isAlive() {
return numberOfLives >= 0;
}
}
.
(Custom)
, . , Cat :
Cat cat = new Cat("Fluffy", 9, "White");
, — , .
, , 9. , , 9 . , .
public record Cat(String name, int numberOfLives, String color) {
public Cat(String name, String color) {
this(name, 9, color);
}
}
. . , . , , :
public record Cat(String name, int numberOfLives, String color) {
public Cat(String name,int numberOfLives, String color) {
if(numberOfLives < 0) {
throw new IllegalArgumentException("Number of lives cannot be less than 0.");
}
if(numberOfLives > 9) {
throw new IllegalArgumentException("Cats cannot have that many lives.");
}
this.name = name;
this.numberOfLives = numberOfLives;
this.color = color;
}
}
, ( ), . , .
public record Cat(String name, int numberOfLives, String color) {
public Cat {
}
}
java.lang.Class , , .
isRecord(). , , - :
Cat cat = new Cat("Fluffy", 9, "White");
if(cat.getClass().isRecord()) {
}
getRecordComponents(). , . java.lang.reflect.RecordComponent. , , :
!
, , Java 14 ( 2/2020).
Preview feature ( )
(Records) Java 14. . ?
VM — Java SE, , , . JDK ; , Java SE.
JDK , , Java SE , , . , ( ), ( ), .
JDK, . . , , , .
, JDK 14.
IntelliJ IDEA
IntelliJ IDEA Preview feature File → Project Structure.
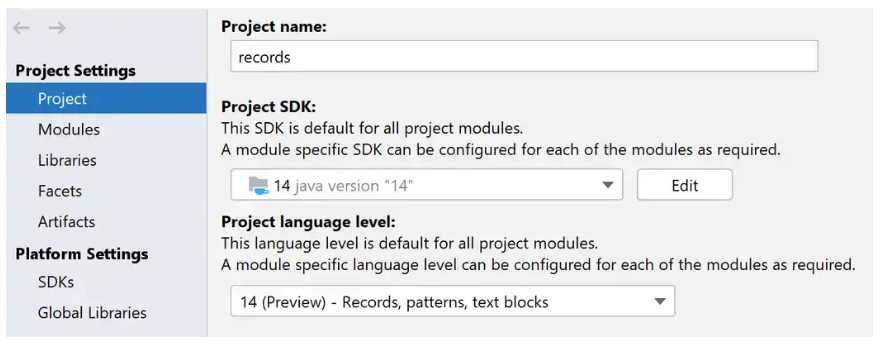
Um Einträge in IntelliJ IDEA verwenden zu können, benötigen Sie Version 2020.1 und höher. Ab 2/2020 ist es als Early Access Program Build verfügbar . IDEA bietet derzeit grundlegende Unterstützung für Aufnahmen, die vollständige Unterstützung sollte jedoch in der Release-Version verfügbar sein.
Manuelle Zusammenstellung
Eine Alternative besteht darin, das Projekt manuell zusammenzusetzen. Dann müssen Sie die folgenden Optionen für javac bereitstellen:
javac --release 14 --enable-preview ...
Dies dient zur Zusammenstellung. Zur Laufzeit stellen Sie einfach --enable-Preview bereit
java --enable-preview ...
Maven-Projekte
Für Maven-Builds können Sie die folgende Konfiguration verwenden:
<build>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<release>14</release>
<compilerArgs>
--enable-preview
</compilerArgs>
```14</source>
<target>14</target>
</configuration>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<argLine>--enable-preview</argLine>
</configuration>
</plugin>
<plugin>
<artifactId>maven-failsafe-plugin</artifactId>
<configuration>
<argLine>--enable-preview</argLine>
</configuration>
</plugin>
</plugins>
</build>