تتناول هذه المقالة إنشاء اختبار ذاتي بسيط إلى حد ما. ستكون هذه المقالة مفيدة لأتمتة المبتدئين.
يتم تقديم المواد بقدر الإمكان ، ومع ذلك ، سيكون من الأسهل بكثير فهم ما نتحدث عنه إذا كان لديك على الأقل الحد الأدنى من الأفكار حول لغة جافا: الفصول الدراسية والأساليب وما إلى ذلك.
سنحتاج إلى:
- بيئة التطوير المثبتة Intellij IDEA (هي IDE الأكثر شيوعًا ، في معظم الحالات يكفي إصدار مجاني من Community Edition) ؛
- تثبيت Java (jdk / openjdk) و Maven ، مسجلة في بيئة نظام التشغيل ؛
- متصفح Chrome و chromedriver - برنامج لإرسال الأوامر إلى المتصفح.
إنشاء المشروع
قم بتشغيل Intellij IDEA ، وانتقل إلى النقاط القليلة الأولى فيما يتعلق بإرسال الإحصائيات ، واستيراد المشاريع ، واختيار نظام الألوان ، وما إلى ذلك. - ما عليك سوى تحديد الخيارات الافتراضية.
في النافذة التي تظهر في النهاية ، حدد "إنشاء مشروع جديد" ، ونوع المشروع هو مافن. ستبدو النافذة كما يلي:

- Maven هو أداة بناء لمشاريع Java.
- Project SDK - إصدار Java المثبت على الكمبيوتر ؛
- إنشاء من النموذج الأصلي هو القدرة على إنشاء مشروع بنموذج أصلي محدد (في هذه المرحلة ، لا يلزم وضع علامة على مربع الاختيار هذا).
انقر فوق {التالي." سيتم فتح النافذة التالية:

Groupid و Artifactid هي معرّفات المشاريع في Maven. هناك قواعد معينة لملء هذه العناصر:
- Groupid — . Java: . , -, com.email.email;
- Artifactid — ;
- Version — .
«Finish»: IDE pom.xml:

, : Groupid, Artefiactid, Version. Pom.xml — . Pom- (), .
: Selenium Java Junit. Maven mvnrepository.com, Selenium Java :

( 3.14.0). :

«Maven» pom.xml
<dependencies> </dependencies>
. Junit ( 4.12).
pom-:
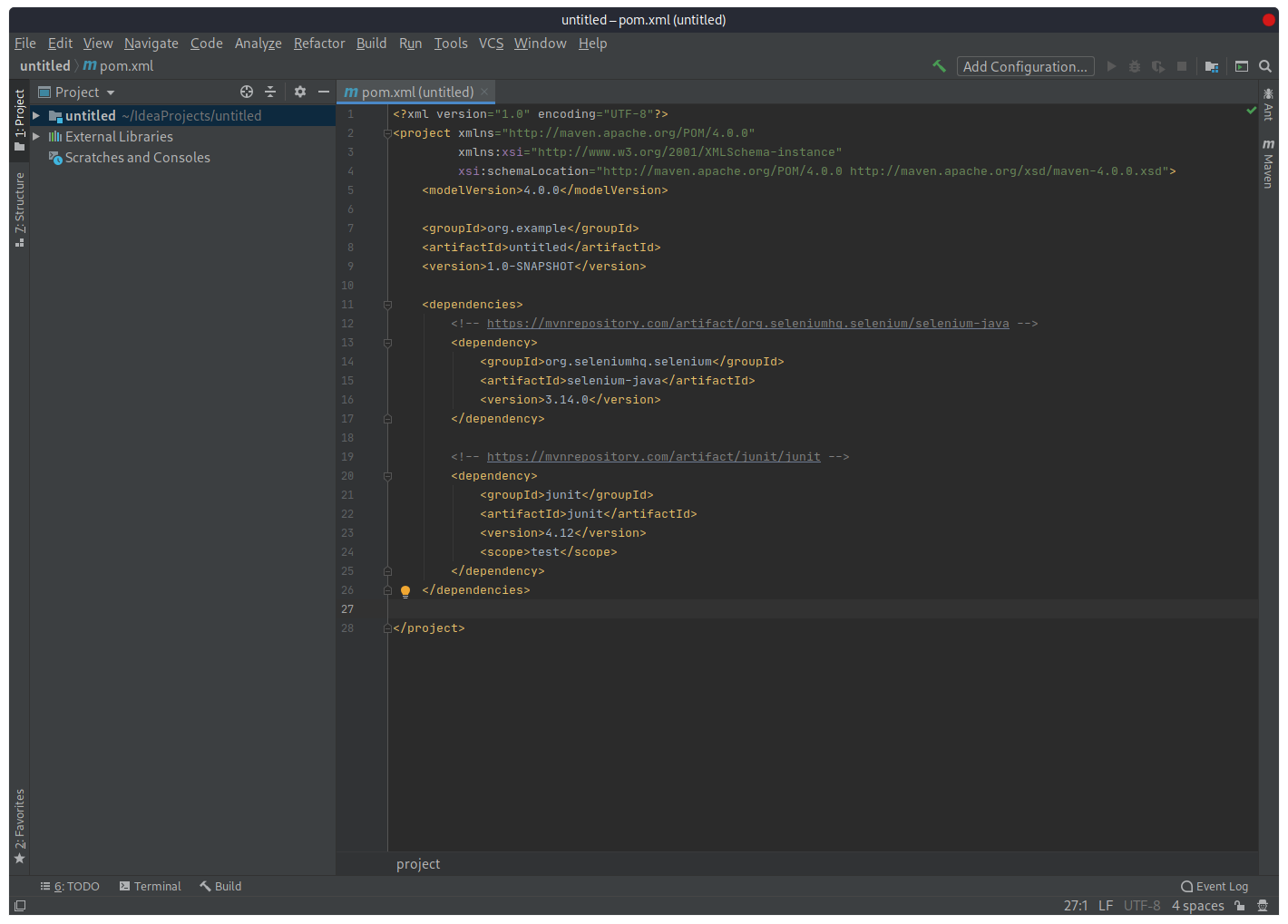
. src : «main» «test». , , «test». «test», «java» «New», «Package». . , Groupid — «org.example».
— Java, . «New», «Java Class».
Java , , LoginTest ( Java ). IDE :

IDE
, IDE. «Open Module Settings». «Sources» «Language level» 5. 8 ( , Java) :

Java: «File», Settings.
«Build, Execution, Deployment» -> «Compiler» -> «Java Compiler». 1.5. 8 :

Test Suite
:
- ;
- ;
- — ;
- «…».
, .
( ).
LoginTest . «setup()», . , :
WebDriver driver = new ChromeDriver();
WebDriver , chomedriver ( Windows .exe):
System.setProperty("webdriver.chrome.driver", "/usr/bin/chromedriver");
, :
driver.manage().window().maximaze();
, , . . : . Implicitly Wait, :
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
, , (10 ) 500 . , , , .
:
driver.get("https://passport.yandex.ru/auth")
( ).
«test» «resources», «conf.properties», :
loginpage = https:
chromedriver = /usr/bin/chromedriver

«org.example» «ConfProperties», «conf.properties» :
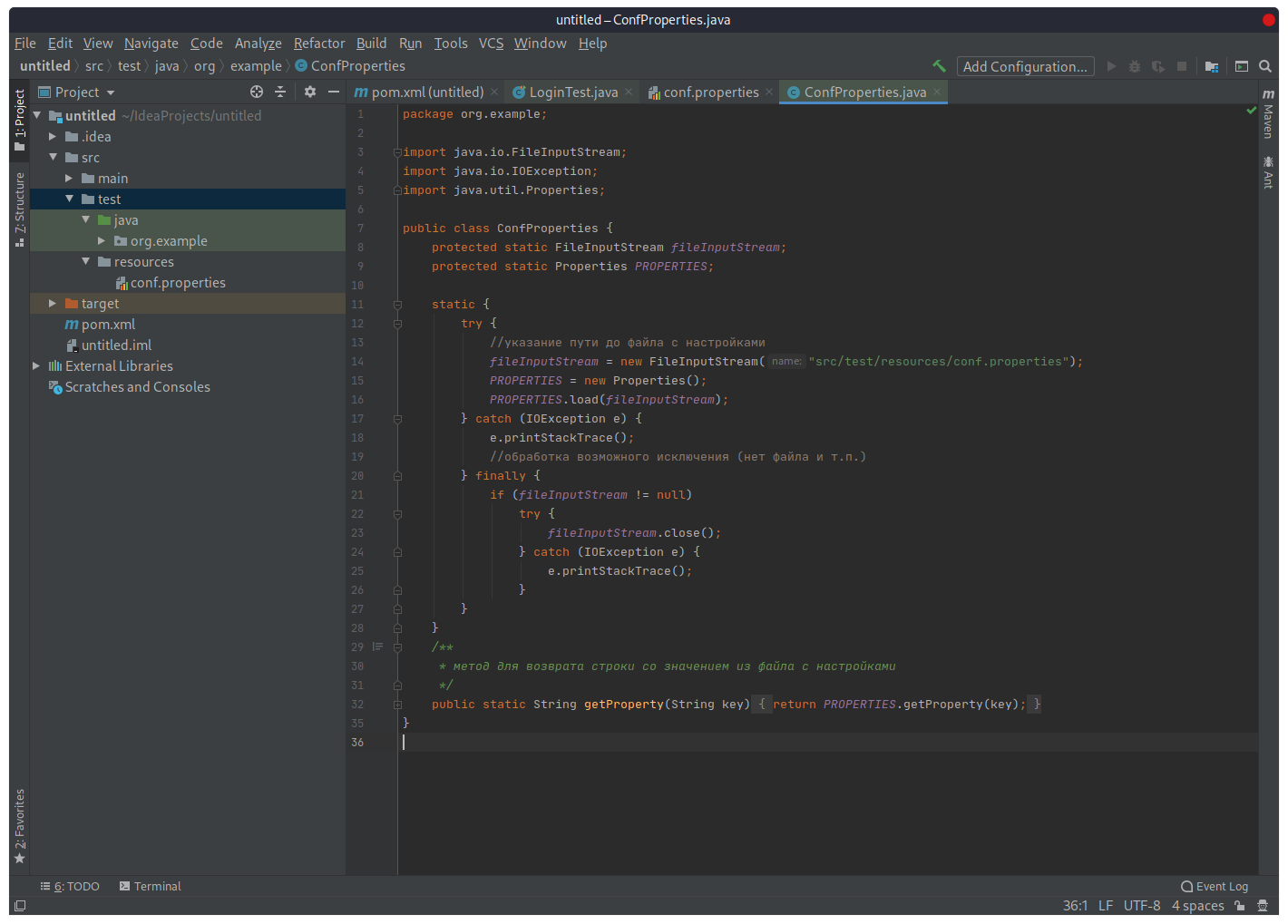
package org.example;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class ConfProperties {
protected static FileInputStream fileInputStream;
protected static Properties PROPERTIES;
static {
try {
fileInputStream = new FileInputStream("src/test/resources/conf.properties");
PROPERTIES = new Properties();
PROPERTIES.load(fileInputStream);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fileInputStream != null)
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace(); } } }
public static String getProperty(String key) {
return PROPERTIES.getProperty(key); } }
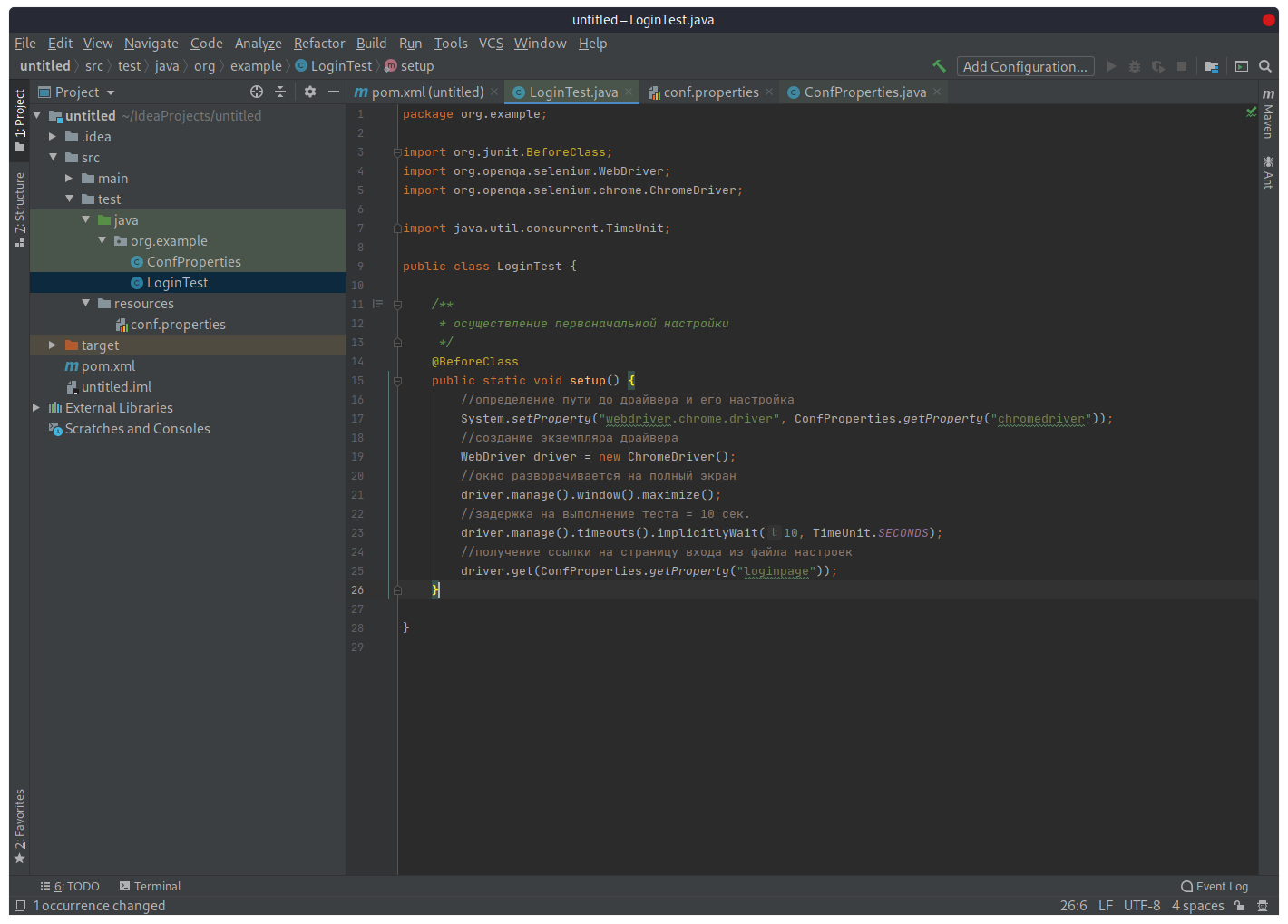
«setup()» Junit «@BeforeClass», , . Junit Test.
package org.example;
import org.junit.BeforeClass;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class LoginTest {
@BeforeClass
public static void setup() {
System.setProperty("webdriver.chrome.driver", ConfProperties.getProperty("chromedriver"));
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get(ConfProperties.getProperty("loginpage")); } }
Page Object
Page Object , , .
«org.example» LoginPage, .
(https://passport.yandex.ru/auth) Chrome. , , . « ». ( ) — .
. . , «Copy» -> «Copy XPath».
Page Object @FindBy.
:
@FindBy(xpath = "//*[@id="root"]/div/div/div[2]/div/div/div[3]/div[2]/div/div/div[1]/form/div[1]/div[1]/label")
private WebElement loginField;
loginField ( LoginPage, .. ).
, xpath ( « xpath ». , , id:

xpath:
@FindBy(xpath = "//*[contains(@id, 'passp-field-login')]")
, , .
.
«»:
@FindBy(xpath = "//*[contains(text(), '')]")
private WebElement loginBtn;
:
@FindBy(xpath = "//*[contains(@id, 'passp-field-passwd')]")
private WebElement passwdField;
.
:
public void inputLogin(String login) {
loginField.sendKeys(login); }
:
public void inputPasswd(String passwd) {
passwdField.sendKeys(passwd); }
:
public void clickLoginBtn() {
loginBtn.click(); }
, @FindBy , PageFactory. Webdriver:
public WebDriver driver;
public LoginPage(WebDriver driver) {
PageFactory.initElements(driver, this);
this.driver = driver; }

package org.example;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
public class LoginPage {
public WebDriver driver;
public LoginPage(WebDriver driver) {
PageFactory.initElements(driver, this);
this.driver = driver; }
@FindBy(xpath = "//*[contains(@id, 'passp-field-login')]")
private WebElement loginField;
@FindBy(xpath = "//*[contains(text(), '')]/..")
private WebElement loginBtn;
@FindBy(xpath = "//*[contains(@id, 'passp-field-passwd')]")
private WebElement passwdField;
public void inputLogin(String login) {
loginField.sendKeys(login); }
public void inputPasswd(String passwd) {
passwdField.sendKeys(passwd); }
public void clickLoginBtn() {
loginBtn.click(); } }
. .. , Page Object . ProfilePage, ( ), . , , .
, :

package org.example;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
public class ProfilePage {
public WebDriver driver;
public ProfilePage(WebDriver driver) {
PageFactory.initElements(driver, this);
this.driver = driver; }
@FindBy(xpath = "//*[contains(@class, 'account__name_hasAccentLetter')]")
private WebElement userMenu;
@FindBy(xpath = "//*[contains(@class, 'menu-item_action_exit menu__item menu__item_type_link')]")
private WebElement logoutBtn;
public String getUserName() {
String userName = userMenu.getText();
return userName; }
public void entryMenu() {
userMenu.click(); }
public void userLogout() {
logoutBtn.click(); } }
: getUserName() , .. «» . , . getUserName() :
public String getUserName() {
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//*[contains(@class, 'account__name_hasAccentLetter')]")));
String userName = userMenu.getText();
return userName; }
LoginTest - :
public static LoginPage loginPage;
public static ProfilePage profilePage;
public static WebDriver driver;
@BeforeClass . new. driver, , :
loginPage = new LoginPage(driver);
profilePage = new ProfilePage(driver);
(.. ):
driver = new ChromeDriver();
. loginTest() :
@Test
public void loginTest() {
loginPage.inputLogin(ConfProperties.getProperty("login"));
loginPage.clickLoginBtn();
loginPage.inputPasswd(ConfProperties.getProperty("password"));
loginPage.clickLoginBtn();
String user = profilePage.getUserName();
Assert.assertEquals(ConfProperties.getProperty("login"), user); }
. @AfterClass ( , ).
«», .
@AfterClass
public static void tearDown() {
profilePage.entryMenu();
profilePage.userLogout();
driver.quit(); }
.
, :
package org.example;
import org.junit.AfterClass;
import org.junit.Assert;
import org.junit.BeforeClass;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class LoginTest {
public static LoginPage loginPage;
public static ProfilePage profilePage;
public static WebDriver driver;
@BeforeClass
public static void setup() {
System.setProperty("webdriver.chrome.driver", ConfProperties.getProperty("chromedriver"));
driver = new ChromeDriver();
loginPage = new LoginPage(driver);
profilePage = new ProfilePage(driver);
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get(ConfProperties.getProperty("loginpage")); }
@Test
public void loginTest() {
loginPage.inputLogin(ConfProperties.getProperty("login"));
loginPage.clickLoginBtn();
loginPage.inputPasswd(ConfProperties.getProperty("password"));
loginPage.clickLoginBtn();
String user = profilePage.getUserName();
Assert.assertEquals(ConfProperties.getProperty("login"), user); }
@AfterClass
public static void tearDown() {
profilePage.entryMenu();
profilePage.userLogout();
driver.quit(); } }
Intellij Idea :
, Idea , loginTest() :
